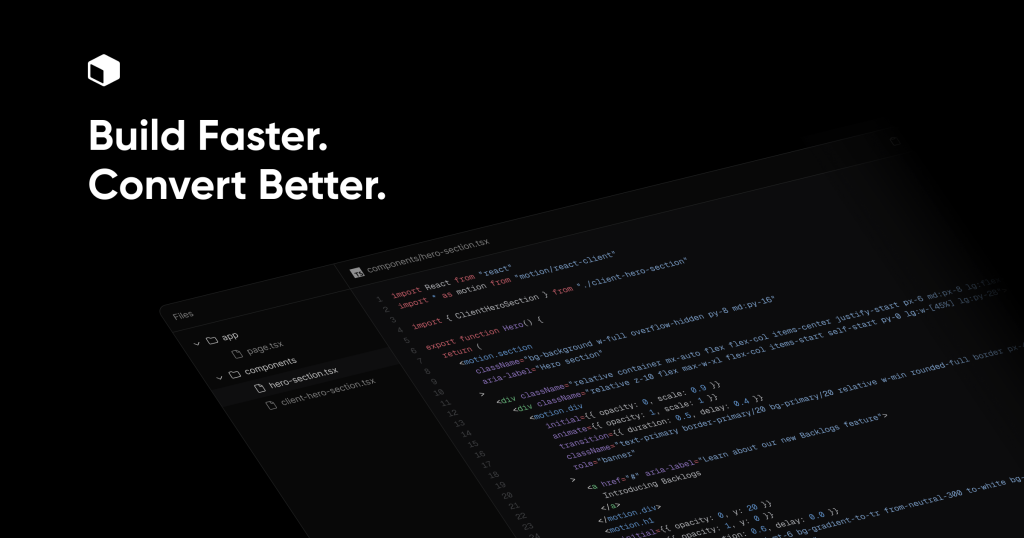
BadtzUI Pro
PaidPremium blocks & templates for high conversion and SEO
Discover free and premium HTML components. HTML components are reusable code snippets like buttons, forms, and menus that simplify web development.
Premium blocks & templates for high conversion and SEO
680+ Shadcn Tailwind blocks & templates
Preline: 740+ Tailwind pages & components
500+ Tailwind Plus UI components
225+ responsive Tailwind CSS components
600+ ready-made Tailwind CSS components
TailwindCSS and shadcn/ui UI kit
300+ drop-in Tailwind UI blocks for rapid design
Framer Motion components from ibelick
400+ UI Components for Tailwind CSS
Alpine.js-powered Tailwind announcement banner
Stacked or grid Tailwind avatar group component
450+ UI components for Tailwind CSS
Tailwind dropdown component with Alpine.js
50+ UI Components for Tailwind CSS
Responsive Tailwind grid components in HTML
Tailwind contact form component built with Alpine.js
Responsive and minimal table component built with Tailwind CSS.
500+ Tailwind components with Figma file
570+ Tailwind components with HTML, React
Tailwind roadmap, timeline component
Free Tailwind CSS components for HTML & JSX
Tailwind signin - login components
Legal content components
Tailwind logo cloud components
Responsive, rounded badge components
Tailwind team components
Tailwind newsletter, form components
Tailwind testimonial components
Tailwind integrations components
Tailwind how it works components
Tailwind filter components
Tailwind footer components
Tailwind feature components
Overlapping circular avatars in a neat stack
Tailwind stats, metrics components
Tailwind 404, error page components
Tailwind contact components
Tailwind call to action components
Tailwind blog components
Tailwind About Us components
Alpine.js and Tailwind text area component by Pines UI Library.
Tailwind tabs component with Alpine.js
Alpine.js and Tailwind tooltip component by Pines UI Library.
130+ Free Tailwind UI Sections
Alpine.js and Tailwind popover component by Pines UI Library.
Alpine.js and Tailwind toggle component by Pines UI Library.
Alpine.js and Tailwind image gallery component by Pines UI Library.
3 Tailwind navbar components in HTML and React
Alpine.js and Tailwind progress component by Pines UI Library.
Alpine.js and Tailwind radio group component by Pines UI Library.
Alpine.js and Tailwind video component by Pines UI Library.
Alpine.js and Tailwind image gallery component by Pines UI Library.
A clean sidebar that has hover states for navigation items
Alpine.js and Tailwind menu component by Pines UI Library.
Alpine.js and Tailwind slide-over component by Pines UI Library.
Alpine.js and Tailwind animated text component by Pines UI Library.
Tailwind tree view menu component
Alpine.js and Tailwind toast notification component by Pines UI Library.
Alpine.js and Tailwind hover card component by Pines UI Library.
Alpine.js and Tailwind modal component by Pines UI Library.
Alpine.js and Tailwind select dropdown menu component by Pines UI Library.
Alpine.js and Tailwind command palette component by Pines UI Library.
Alpine.js and Tailwind date picker component by Pines UI Library.
Alpine.js and Tailwind FAQ component by Pines UI Library.
52 Free to use Tailwind buttons
600+ Tailwind CSS Components and blocks
Tailwind-powered UI kit for React, Vue & Solid
Generate Tailwind CSS components using Claude 3.5 Sonnet
Animated Tailwind & Three.js web components
500+ customizable Tailwind components for React
Alpine.js Tailwind banner component to add a sticky CTA on landing pages.
Tailwind accordion component with Alpine.js
Tailwind hero components
Tailwind pricing components
0 javascript infinite carousel-slider component with logo cloud example
Countdown with transition effect
Tailwind CSS coupon code component
350+ free, customizable Tailwind CSS components
Open-source neobrutalism Tailwind UI component library
30+ landing page & UI components made with Tailwind CSS
20 free, pre-designed Tailwind CSS buttons ready to copy
A collection of free Tailwind CSS eCommerce components
Free Tailwind & Alpine component library
Clean sign-up form component in Tailwind CSS
Sticky bottom button for Tailwind CSS
Customizable password reset form built with Tailwind CSS
7 Tailwind portfolio, grid components by Tailspark
Free pricing UI component built with Tailwind CSS
Windows 11 desktop UI concept built with Tailwind CSS
Basic UI components for Tailwind CSS
Reusable Tailwind-based UI toolkit
19 HTML & React UI components
Button animation snippets made with TailwindCSS
Collection of 44 TailwindCSS buttons
Accessible, responsive UI components for Tailwind projects
Modular Tailwind CSS components with vanilla JS
80+ ready-made Tailwind CSS components
60+ customizable Tailwind CSS blocks
120 Free Tailwind CSS blocks & components
226 Free & Open Source Tailwind CSS Components
600+ TailGrids UI blocks for instant Tailwind designs
300+ Free Tailwind CSS components
Best Tailwind components and elements to use on your web projects.
Preline: 740+ Tailwind pages & components
Hundreds of premium components & blocks built for Tailwind & Shadcn UI
In the evolving landscape of web development, creating reusable and maintainable code is paramount. HTML Components have emerged as a cornerstone for building modular and scalable web applications.
By encapsulating HTML, CSS, and JavaScript into self-contained units, developers can streamline their workflows, enhance consistency, and simplify maintenance. This guide explores the essence of HTML Components, their benefits, how to create and use them effectively, and answers some common questions to help you leverage this powerful tool in your web projects.
HTML Components, often synonymous with Web Components, are a set of web platform APIs that allow developers to create reusable custom elements with encapsulated functionality and styling. These components can be used across various projects and frameworks, promoting consistency and efficiency in web development.
Web Components consist of four main technologies:
Custom Elements: Define new HTML tags and their behavior.
Shadow DOM: Encapsulate a component’s internal structure and styles, preventing them from affecting the rest of the document.
HTML Templates: Define reusable chunks of HTML that aren't rendered immediately but can be instantiated when needed.
ES Modules: Allow for modular JavaScript, enabling components to import and export functionality seamlessly.
Understanding these technologies is essential for effectively creating and managing HTML Components.
One of the primary advantages of HTML Components is their reusability. By creating a component once, you can use it across multiple projects or different parts of the same application without rewriting the code. This not only saves time but also ensures consistency in design and functionality.
HTML Components encapsulate their structure, style, and behavior. This means that the internal workings of a component are hidden from the rest of the application, preventing unintended interactions and making debugging easier. Encapsulation also allows developers to modify a component without affecting other parts of the application.
Web Components are built on standard web technologies, making them compatible with any framework or library that works with HTML. Whether you're using React, Vue, Angular, or vanilla JavaScript, HTML Components can be integrated seamlessly, offering flexibility in how you build your applications.
Creating an HTML Component involves defining a custom element and specifying its behavior and appearance. Here’s a streamlined process to create a simple component:
Use the class
syntax to define the behavior of your custom element by extending the HTMLElement
base class.
class MyButton extends HTMLElement {
constructor() {
super();
// Initialization code
}
connectedCallback() {
this.innerHTML = `<button>Click Me!</button>`;
}
}
customElements.define('my-button', MyButton);
Once defined, you can use your custom element just like any standard HTML tag.
<my-button></my-button>
This simple example creates a custom button component that can be reused throughout your application.
Styling is a crucial aspect of HTML Components, and with the Shadow DOM, styles can be scoped exclusively to the component. This prevents styles from leaking out or being affected by external styles, ensuring that each component maintains its intended appearance.
class MyCard extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
shadow.innerHTML = `
<style>
.card {
border: 1px solid #ccc;
padding: 16px;
border-radius: 8px;
box-shadow: 2px 2px 12px rgba(0,0,0,0.1);
}
</style>
<div class="card">
<slot></slot>
</div>
`;
}
}
customElements.define('my-card', MyCard);
In this example, the styles defined within the <style>
tag apply only to the .card
class inside the my-card
component, ensuring no conflicts with other styles on the page.
Effective communication between components is essential for creating dynamic and responsive applications. HTML Components achieve this through attributes, properties, and events.
Attributes are used to pass data from a parent component to a child component. These can be accessed within the child component via properties.
<user-card username="JaneDoe"></user-card>
Components can emit custom events to notify parent components of changes or actions.
class MyButton extends HTMLElement {
connectedCallback() {
this.innerHTML = `<button>Click Me!</button>`;
this.querySelector('button').addEventListener('click', () => {
this.dispatchEvent(new Event('button-clicked'));
});
}
}
customElements.define('my-button', MyButton);
In the parent component, you can listen for the button-clicked
event to handle the action accordingly.
<my-button id="btn"></my-button>
<script>
document.getElementById('btn').addEventListener('button-clicked', () => {
alert('Button was clicked!');
});
</script>
Slots allow you to pass content from a parent component into a child component, enhancing flexibility and reusability.
class MyWrapper extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
shadow.innerHTML = `<div class="wrapper"><slot></slot></div>`;
}
}
customElements.define('my-wrapper', MyWrapper);
<my-wrapper>
<p>This content is passed into the wrapper component.</p>
</my-wrapper>
HTML Components come with lifecycle callbacks that allow you to execute code at specific stages of a component’s existence, such as when it’s added to the DOM or removed.
connectedCallback()
: Invoked when the component is added to the DOM.
disconnectedCallback()
: Invoked when the component is removed from the DOM.
attributeChangedCallback()
: Invoked when an attribute of the component changes.
These callbacks provide hooks to manage resources, update the UI, or perform cleanup tasks.
Keep Components Modular: Design components to handle a single responsibility. This makes them easier to manage, test, and reuse.
Use Clear Naming Conventions: Name your custom elements with a prefix to avoid conflicts with standard HTML elements (e.g., my-button
, user-card
).
Leverage Shadow DOM for Encapsulation: Utilize Shadow DOM to encapsulate styles and structure, preventing unwanted side effects.
Expose a Clear API: Define clear and intuitive attributes and methods for your components to ensure they are easy to use and integrate.
Document Your Components: Provide documentation for your components' attributes, events, and methods to facilitate collaboration and future maintenance.
UI Widgets: Buttons, modals, dropdowns, and other interactive elements.
Form Elements: Custom input fields, sliders, and checkboxes that offer enhanced functionality.
Data Display: Components like tables, cards, and lists for presenting data consistently.
Navigation: Headers, footers, and sidebars that provide consistent navigation across the application.
Reusable Layouts: Components that define common layouts, reducing repetition in your codebase.
By leveraging HTML Components for these use cases, developers can build complex applications more efficiently and maintain a high level of consistency throughout their projects.
To ensure your HTML Components perform efficiently and integrate smoothly within your applications, consider the following optimization techniques:
While Shadow DOM provides excellent encapsulation, excessive use can lead to performance overhead. Use it judiciously, especially for components that require strict style and structure isolation.
For large applications, consider lazy loading components to reduce the initial load time. This can be achieved by dynamically importing components as needed, improving overall performance.
Keep your CSS within components minimal and avoid overly complex selectors. Leveraging CSS variables and reusable classes can help maintain efficient and scalable styles.
Ensure that the JavaScript within your components is optimized and free from unnecessary computations or memory leaks. This enhances the responsiveness and reliability of your components.
Transitioning from other frameworks or vanilla JavaScript to HTML Components involves several steps:
Analyze Existing Components: Identify the structure, styles, and behavior of your current components.
Define Custom Elements: Create new custom elements using the Custom Elements
API, encapsulating the existing functionality.
Integrate Styles and Templates: Move your styles into the Shadow DOM and utilize HTML Templates for reusable markup.
Handle State and Events: Adapt state management and event handling to align with HTML Components’ paradigms.
Test Thoroughly: Ensure that the migrated components function correctly within your application context.
A methodical approach ensures a smooth transition, preserving functionality while reaping the benefits of HTML Components.
HTML Components, powered by Web Components standards, offer a robust and flexible way to build reusable, encapsulated, and interoperable elements for web applications. By leveraging Custom Elements, Shadow DOM, and HTML Templates, developers can create modular components that enhance productivity, maintainability, and scalability.
Embracing HTML Components facilitates a more organized and efficient development process, enabling you to build complex applications with ease. Whether you’re working on a small project or a large-scale application, mastering HTML Components will empower you to craft dynamic and engaging user interfaces that stand the test of time.
Dive into the world of HTML Components, experiment with creating your own, and explore the vast ecosystem of tools and libraries available. With the knowledge and best practices outlined in this guide, you’re well-equipped to harness the full potential of HTML Components in your web development endeavors.
You can find answers for commonly asked questions about components.
Yes, HTML Components can be nested within each other, allowing you to build complex and hierarchical user interfaces by composing simpler components.
State within an HTML Component can be managed using JavaScript properties. For more complex state management, integrating with state management libraries or utilizing custom events to communicate state changes to parent components can be effective strategies.
Web Components are a set of standardized technologies that enable the creation of reusable and encapsulated HTML Components. They include Custom Elements, Shadow DOM, HTML Templates, and ES Modules, which together allow developers to build modular and interoperable components.
State within an HTML Component can be managed using JavaScript properties. For more complex state management, integrating with state management libraries or utilizing custom events to communicate state changes to parent components can be effective strategies.
Yes, HTML Components are framework-agnostic and can be integrated with popular JavaScript frameworks such as React, Vue, and Angular. This interoperability allows developers to use Web Components within their preferred frameworks seamlessly.