Tailwind Toggle Component
Alpine.js and Tailwind toggle component by Pines UI Library.
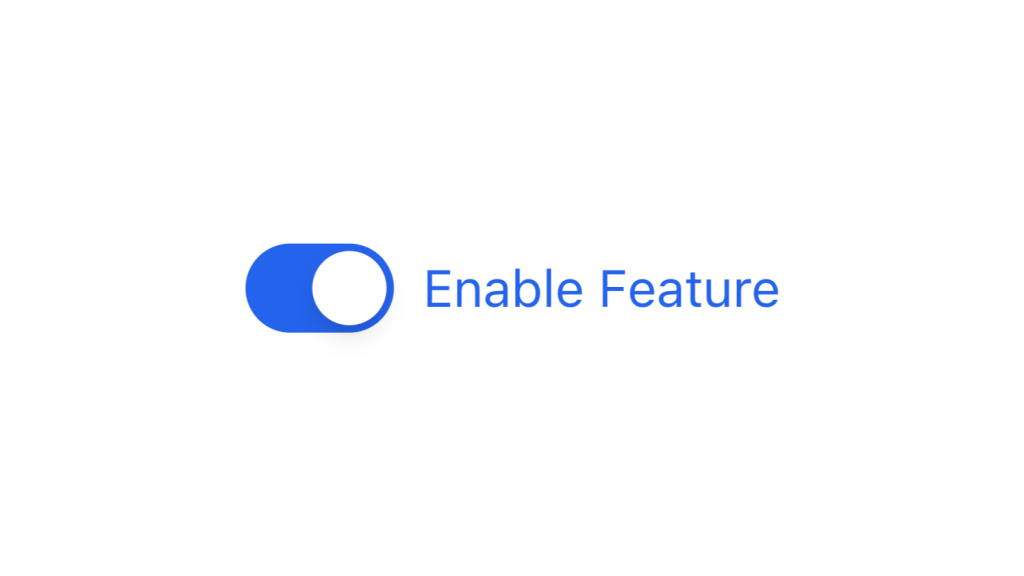
- Documentation
- Custom config file
- Open Source
- JavaScript Plugin
- Copy & Paste
- Tailwind CSS v3
- Responsive Design
Creating Interactive Toggle Components with Tailwind CSS
Tailwind CSS has revolutionized the way developers approach styling by offering a utility-first framework that emphasizes reusable classes and rapid development. Among the myriad of interactive elements you can build with Tailwind, toggle components—like switches for dark mode, settings, or feature toggles—are both essential and highly customizable.
Why Use Toggle Components?
Toggle switches are ubiquitous in web applications. They allow users to switch between two states effortlessly—such as enabling/disabling features, toggling dark mode, or accepting terms and conditions. Well-designed toggles enhance user experience by providing intuitive controls that align with the overall design aesthetic of the application.
Building a Basic Toggle Switch
Let’s start by constructing a simple toggle switch using Tailwind CSS. This foundational component can be expanded and customized to fit various use cases.
Basic Toggle Structure
Here’s a minimalist example of a toggle switch:
<button class="w-14 h-8 flex items-center bg-gray-300 rounded-full p-1 transition-colors duration-300 focus:outline-none">
<span class="w-6 h-6 bg-white rounded-full shadow-md transform transition-transform duration-300"></span>
</button>
Breaking It Down:
Container (
<button>
):w-14 h-8
: Sets the width and height.flex items-center
: Centers the toggle circle vertically.bg-gray-300
: Sets the background color.rounded-full
: Makes the container fully rounded.p-1
: Adds padding around the toggle circle.transition-colors duration-300
: Smoothly transitions background color changes.focus:outline-none
: Removes the default focus outline for a cleaner look.
Toggle Circle (
<span>
):w-6 h-6
: Dimensions of the toggle handle.bg-white
: Sets the circle’s color.rounded-full
: Makes the toggle handle fully rounded.shadow-md
: Adds a subtle shadow for depth.transform transition-transform duration-300
: Enables smooth sliding animation when toggled.
Interactivity with JavaScript
To make the toggle functional, JavaScript can be used to handle state changes. Depending on your project setup, frameworks like React, Vue, or even vanilla JavaScript can manage the toggle state by adding or removing classes that adjust the component’s appearance.
Example with Vanilla JavaScript:
<button id="toggle" class="...">
<span id="toggle-circle" class="..."></span>
</button>
<script>
const toggle = document.getElementById('toggle');
const toggleCircle = document.getElementById('toggle-circle');
toggle.addEventListener('click', () => {
toggle.classList.toggle('bg-blue-500');
toggleCircle.classList.toggle('transform translate-x-full');
// Add additional state management as needed
});
</script>
In this example, clicking the toggle button changes its background color and moves the toggle circle to indicate the active state.
Customizing the Toggle Switch
Tailwind's utility classes make it incredibly easy to tailor toggle switches to match your design requirements. Let’s explore some customization options:
a. Adjusting Size
You might need larger or smaller toggle switches based on your design. Tailwind’s width (w-
), height (h-
), and padding (p-
) classes allow you to adjust these dimensions effortlessly.
Example: Larger Toggle
<button class="w-16 h-10 ...">
<span class="w-8 h-8 ..."></span>
</button>
b. Changing Colors
Tailwind offers a vast palette of colors. You can switch the background and toggle handle colors to fit your theme.
Example: Dark Mode Toggle
<button class="bg-gray-700 ...">
<span class="bg-gray-100 ..."></span>
</button>
c. Adding Borders
For a more defined look, borders can be incorporated.
<button class="border border-gray-400 ...">
<span class="border border-gray-200 ..."></span>
</button>
d. Incorporating Icons
Sometimes, adding icons enhances the toggle's functionality, like displaying a sun and moon for theme switching.
Example with SVG Icons:
<button class="relative w-14 h-8 ...">
<span class="absolute left-1 top-1">
<!-- Sun Icon SVG -->
</span>
<span class="w-6 h-6 bg-white rounded-full shadow-md transform transition-transform duration-300"></span>
<span class="absolute right-1 top-1">
<!-- Moon Icon SVG -->
</span>
</button>
By layering SVG icons, users can immediately recognize the toggle’s purpose.
User Experience with Animations
Animations can significantly improve the user experience by providing visual feedback. Tailwind CSS simplifies adding smooth transitions and transforms.
a. Transition Effects
The transition
, duration
, and ease
classes help create fluid animations.
Example: Smooth Slide Animation
<button class="transition-colors duration-300 ...">
<span class="transform transition-transform duration-300"></span>
</button>
When the toggle state changes, the translate-x-full
class can move the toggle circle smoothly from one side to the other.
b. Hover and Focus States
Enhancing interactivity by adding hover and focus effects makes toggles feel more responsive.
<button class="hover:bg-gray-400 focus:ring-2 focus:ring-blue-500 ...">
<span class="hover:bg-gray-200 ..."></span>
</button>
These classes change the background color on hover and add a focus ring when the toggle is focused, aiding accessibility.
Accessibility
Accessibility is paramount in modern web development. Tailwind CSS doesn't inherently handle accessibility, but with proper HTML structure and ARIA attributes, you can make your toggle components accessible to all users.
a. Semantic HTML
Using the <button>
element for the toggle ensures it's keyboard-navigable and focusable by default.
b. ARIA Attributes
Adding ARIA attributes communicates the toggle’s state to assistive technologies.
Example:
<button aria-pressed="false" ...>
<span ...></span>
</button>
Toggling the aria-pressed
attribute to true
when active informs screen readers of the current state.
c. Keyboard Navigation
Ensure that the toggle can be operated using keyboard inputs like Enter
or Space
. The <button>
element handles this by default, but if you use a different element, you need to manage keyboard events manually.
d. Focus Indicators
Maintaining visible focus indicators aids users navigating via keyboard.
<button class="focus:outline-none focus:ring-2 focus:ring-blue-500 ...">
<span ...></span>
</button>
These classes add a ring around the toggle when it's focused, making it clear which element is active.
6. Integrating Toggle Components with JavaScript Frameworks
Tailwind CSS plays well with various JavaScript frameworks, allowing for dynamic and state-driven toggle components. Let’s briefly explore integration with popular frameworks like React and Vue.
a. React Integration
In React, you can manage the toggle state using hooks and conditionally apply Tailwind classes based on the state.
Example:
import { useState } from 'react';
function ToggleSwitch() {
const [isOn, setIsOn] = useState(false);
return (
<button
onClick={() => setIsOn(!isOn)}
className={`w-14 h-8 flex items-center rounded-full p-1 transition-colors duration-300 ${
isOn ? 'bg-blue-500' : 'bg-gray-300'
} focus:outline-none`}
aria-pressed={isOn}
>
<span
className={`w-6 h-6 bg-white rounded-full shadow-md transform transition-transform duration-300 ${
isOn ? 'translate-x-6' : 'translate-x-0'
}`}
></span>
</button>
);
}
This React component toggles its state on click, updating the classes accordingly to reflect the active or inactive state.
b. Vue Integration
In Vue, reactivity makes managing the toggle state straightforward.
Example:
<template>
<button
@click="isOn = !isOn"
:class="[
'w-14 h-8 flex items-center rounded-full p-1 transition-colors duration-300 focus:outline-none',
isOn ? 'bg-blue-500' : 'bg-gray-300'
]"
:aria-pressed="isOn"
>
<span
:class="[
'w-6 h-6 bg-white rounded-full shadow-md transform transition-transform duration-300',
isOn ? 'translate-x-6' : 'translate-x-0'
]"
></span>
</button>
</template>
<script>
export default {
data() {
return {
isOn: false,
};
},
};
</script>
This Vue component uses data binding to manage the toggle state, dynamically updating classes based on isOn
.
7. Responsive Design Considerations
In today’s multi-device landscape, ensuring your toggle components are responsive is crucial.
a. Scaling with Screen Size
Tailwind’s responsive modifiers allow you to adjust the toggle’s size and spacing based on the viewport.
Example:
<button class="w-14 h-8 sm:w-16 sm:h-10 ...">
<span class="w-6 h-6 sm:w-8 sm:h-8 ..."></span>
</button>
In this example, the toggle increases in size on screens 640px
and wider (sm
breakpoint).
b. Touch-Friendly Design
Ensure the toggle is large enough to be easily tapped on touch devices. Increasing the height and width using Tailwind’s utility classes can make toggles more accessible on mobile devices.
8. Managing Toggle State Persistence
For features like theme switching, it's often desirable to remember the user's choice across sessions. This can be achieved using browser storage mechanisms like localStorage
.
Example: Persisting Theme Toggle in JavaScript
<button id="theme-toggle" class="...">
<span id="toggle-circle" class="..."></span>
</button>
<script>
const toggle = document.getElementById('theme-toggle');
const toggleCircle = document.getElementById('toggle-circle');
// Check for saved theme in localStorage
const savedTheme = localStorage.getItem('theme');
if (savedTheme === 'dark') {
document.documentElement.classList.add('dark');
toggle.classList.add('bg-blue-500');
toggleCircle.classList.add('transform', 'translate-x-full');
}
toggle.addEventListener('click', () => {
document.documentElement.classList.toggle('dark');
toggle.classList.toggle('bg-blue-500');
toggleCircle.classList.toggle('transform');
toggleCircle.classList.toggle('translate-x-full');
// Save the current theme
if (document.documentElement.classList.contains('dark')) {
localStorage.setItem('theme', 'dark');
} else {
localStorage.setItem('theme', 'light');
}
});
</script>
In this script:
The toggle checks
localStorage
for a saved theme preference on page load and applies it.Clicking the toggle updates the theme and saves the preference.
This ensures that users’ choices persist across sessions, enhancing user experience.
9. Advanced Customizations and Variations
Beyond the basic toggle, Tailwind CSS allows for creating more intricate variations tailored to specific needs.
a. Multi-state Toggles
While standard toggles switch between two states, some applications require multi-state switches.
Example: Tri-State Toggle
You can expand the toggle to cycle through multiple states by managing more complex state logic and adjusting classes accordingly. However, this typically involves more intricate JavaScript handling.
b. Toggle with Labels
Adding labels improves usability by clearly indicating what the toggle controls.
Example:
<div class="flex items-center space-x-3">
<span>Dark Mode</span>
<button class="w-14 h-8 ...">
<span class="w-6 h-6 ..."></span>
</button>
</div>
Here, the label “Dark Mode” informs users about the toggle’s function.
c. Disabled State
Sometimes, toggles need to be disabled based on certain conditions.
Example:
<button class="w-14 h-8 bg-gray-300 rounded-full p-1 opacity-50 cursor-not-allowed" disabled>
<span class="w-6 h-6 bg-white rounded-full shadow-md"></span>
</button>
Applying opacity-50
and cursor-not-allowed
visually indicates that the toggle is inactive, and the disabled
attribute prevents interactions.
11. Troubleshooting Common Issues
No implementation is without its challenges. Here are some common issues and their solutions when working with Tailwind toggle components.
a. Toggle Not Responding to Clicks
Solution:
Ensure that the JavaScript event listeners are correctly attached.
Verify that no CSS is preventing the button from being clickable (e.g., overlapping elements).
b. Animation Jitters or Lag
Solution:
Use hardware-accelerated CSS properties like
transform
andopacity
for smoother animations.Limit the number of simultaneous transitions to reduce browser strain.
c. Accessibility Concerns
Solution:
Double-check ARIA attributes and ensure they accurately reflect the toggle’s state.
Test keyboard navigation and screen reader compatibility to identify and fix accessibility issues.
FAQ
How do I handle the toggle state in Tailwind Toggle Components?
Tailwind CSS handles the styling aspect of toggle components. To manage the toggle state (e.g., on/off), you can use JavaScript frameworks like Vue, React, or vanilla JavaScript to add or remove classes based on user interaction. Additionally, you can utilize Tailwind's dark variant for themes or group-hover for interactive states.
Can I create accessible toggle switches with Tailwind CSS?
Yes, accessibility is crucial. When building toggle switches with Tailwind, ensure to use semantic HTML elements like <button> and include ARIA attributes such as aria-pressed to indicate the toggle state. Also, manage keyboard interactions by handling focus states and enabling toggling via the keyboard.
How do I add animations to Tailwind Toggle Components?
Tailwind CSS offers utility classes for transitions and transformations. You can use classes like transition, duration-300, and ease-in-out to animate the toggle switch's movement and color changes smoothly. Additionally, Tailwind’s transform utilities allow you to shift the toggle handle when the state changes.
Is it possible to customize the size and color of Tailwind Toggle Components?
Absolutely. Tailwind's utility classes make it easy to adjust the size, color, and other styling aspects of toggle components. For example, you can modify the width, height, background-color, and border-radius using classes like w-16, h-8, bg-blue-500, and rounded-full to match your design requirements.