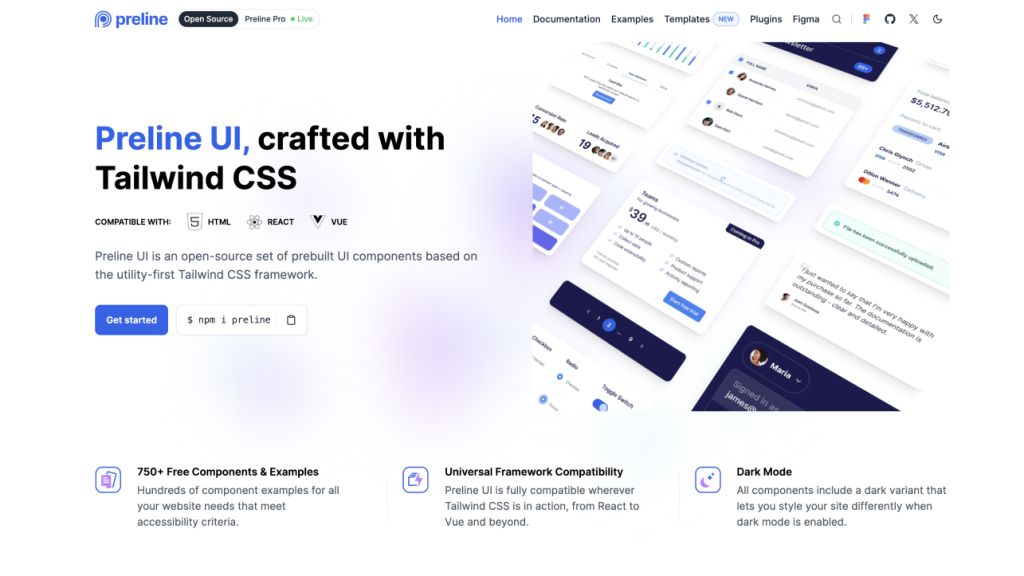
Preline UI
FreeOpen-source set of prebuilt UI components based on the utility-first Tailwind CSS.
Discover Alpine copy to clipboard components. Find the best free & premium copy to clipboard component, and UI elements, to copy the text to the clipboard
Open-source set of prebuilt UI components based on the utility-first Tailwind CSS.
38+ Tailwind Templates & Component Library
Discover the most popular Tailwind CSS ui components and elements. Browse top-notch Tailwind components to enhance your development process.
1300+ UI components for Tailwind CSS