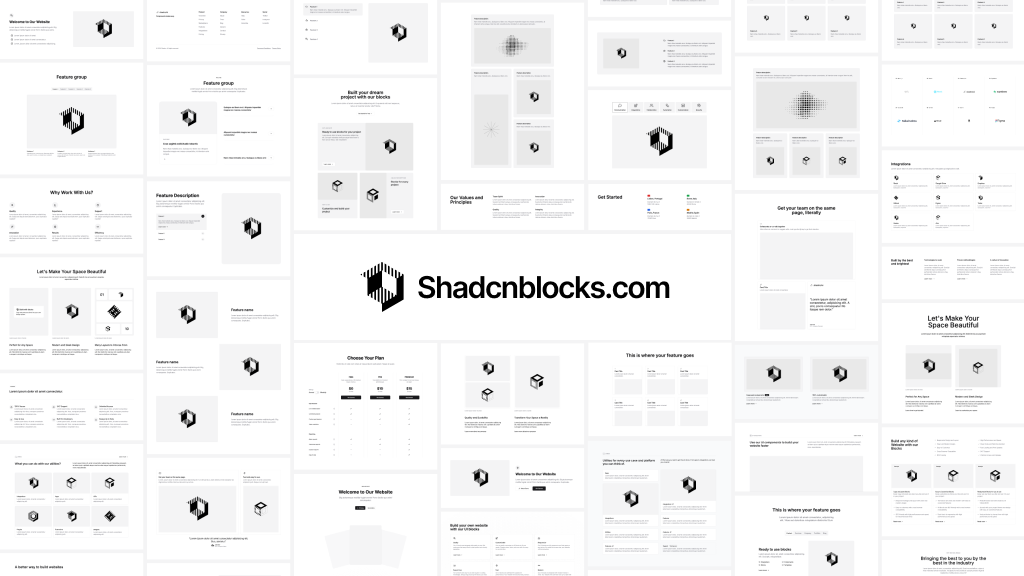
Shadcnblocks
PaidHundreds of premium components & blocks built for Tailwind & Shadcn UI
Learn about Vite Components that provide an optimized development workflow with lightning-fast builds and Hot Module Replacement (HMR)
Hundreds of premium components & blocks built for Tailwind & Shadcn UI
A Bundle of 40+ Tailwind Templates
Discover the most popular Tailwind CSS ui components and elements. Browse top-notch Tailwind components to enhance your development process.
1300+ UI components for Tailwind CSS
In the ever-evolving landscape of web development, efficiency and speed are paramount. Enter Vite, a modern frontend build tool that has been making waves for its lightning-fast performance and developer-friendly features.
Before diving into components, let's briefly touch on what Vite is all about. Created by Evan You, the mind behind Vue.js, Vite is a build tool that offers a faster and leaner development experience for modern web projects. It leverages native ES modules, providing near-instant server start times and lightning-fast hot module replacement (HMR), making the development process smoother and more efficient.
At its core, Vite Components refer to the individual building blocks of your application when using Vite as your development environment. Components are reusable pieces of code that encapsulate HTML, CSS, and JavaScript, allowing developers to build scalable and maintainable applications. Vite's architecture is designed to optimize the creation, management, and bundling of these components, ensuring they perform seamlessly in both development and production environments.
Components play a crucial role in modern web development. They promote reusability, maintainability, and scalability, enabling developers to build complex user interfaces by assembling simple, isolated parts. By breaking down your application into components, you can:
Enhance Code Reusability: Write a component once and reuse it across different parts of your application, saving time and reducing redundancy.
Improve Maintainability: Isolated components make it easier to debug, test, and update your code without affecting other parts of the application.
Boost Collaboration: Components provide clear boundaries within the codebase, allowing multiple developers to work on different parts simultaneously without conflicts.
Getting started with Vite is straightforward. Here's a simple rundown:
Install Vite: Begin by installing Vite using your preferred package manager. For example:
npm create vite@latest
Choose a Framework: Vite supports frameworks like Vue, React, Preact, and vanilla JavaScript. Select the one that aligns with your project needs.
Navigate to Your Project Directory:
cd your-project-name
Install Dependencies:
npm install
Start the Development Server:
npm run dev
With Vite set up, you're ready to start building and managing your components efficiently.
Creating components in Vite is similar to other frontend frameworks. Let's take Vue.js as an example:
Create a New Component File:
src/components/MyComponent.vue
Define the Component Structure:
<template>
<div class="my-component">
<h1>Hello, Vite!</h1>
</div>
</template>
<script>
export default {
name: 'MyComponent',
};
</script>
<style scoped>
.my-component {
color: #42b983;
}
</style>
Use the Component in Your Application:
<template>
<div id="app">
<MyComponent />
</div>
</template>
<script>
import MyComponent from './components/MyComponent.vue';
export default {
components: {
MyComponent,
},
};
</script>
This modular approach ensures that each component is self-contained, promoting easier maintenance and scalability.
Combining Vite with a component-based architecture offers several advantages:
Vite's use of native ES modules and its efficient bundling process result in rapid server start times and near-instantaneous hot module replacement. This means that changes to your components are reflected in the browser almost immediately, significantly speeding up the development process.
Vite intelligently bundles your components for production, ensuring optimal performance. It automatically splits your code, minimizing the bundle size and improving load times for end-users.
Vite's seamless integration with modern frameworks and support for TypeScript, JSX, and CSS preprocessors make it a versatile tool for developers. Its comprehensive documentation and active community further enhance the developer experience, providing ample resources and support.
Vite's plugin system allows you to extend its functionality to suit your project's specific needs. Whether you need support for additional frameworks, advanced linting, or custom build steps, Vite's ecosystem has you covered.
To get the most out of Vite and its component system, consider the following best practices:
Aim to create components that do one thing and do it well. Small, focused components are easier to manage, test, and reuse across your application.
Utilize Vite's HMR feature to see changes in real-time without refreshing the browser. This enhances productivity and provides immediate feedback during development.
When styling your components, use scoped styles to prevent CSS from leaking into other parts of your application. This ensures that styles remain modular and maintainable.
Take advantage of Vite's efficient import mechanism by importing only the components and modules you need. This helps keep your bundle size minimal and improves load times.
If possible, use TypeScript with your components. Type safety can prevent many common bugs and make your codebase more robust and easier to navigate.
While Vite has garnered much attention, it's essential to understand how it stacks up against other build tools like Webpack and Parcel.
Development Speed: Vite offers significantly faster development server start times and HMR compared to Webpack, thanks to its native ES module support.
Configuration: Vite is generally easier to configure out of the box, whereas Webpack can require more extensive setup for complex projects.
Ecosystem: Webpack has a larger ecosystem with more plugins and integrations, but Vite's ecosystem is growing rapidly.
Performance: Both Vite and Parcel emphasize zero-config setups and fast builds, but Vite often edges out in development speed due to its focus on native modules.
Flexibility: Vite provides greater flexibility and control over the build process, making it a better fit for larger or more customized projects.
Vite seamlessly integrates with various frontend frameworks, each offering its own approach to component development. Here's a quick look at how Vite works with some popular choices:
Vite and Vue.js were designed with each other in mind. Vue's single-file components (.vue files) work effortlessly with Vite, leveraging its optimized development and build processes to deliver a smooth experience.
For React developers, Vite provides excellent support for JSX and TypeScript. Its fast refresh capabilities ensure that component changes reflect immediately, enhancing the development workflow.
Vite's plugin system includes support for Svelte, allowing developers to build highly reactive components with ease. The combination of Vite's speed and Svelte's simplicity results in efficient and maintainable applications.
While Vite simplifies many aspects of development, you might encounter some challenges along the way. Here are a few common issues and how to address them:
Ensure that all your import paths are correct and that the necessary dependencies are installed. Sometimes, simply reinstalling your node modules can resolve these issues:
rm -rf node_modules
npm install
If your component styles aren't applying as expected, check if you're using scoped styles correctly. Also, verify that there are no CSS conflicts or specificity issues.
Sometimes, hot module replacement might fail. Restarting the development server often resolves this:
npm run dev
If problems persist, check your project's configuration and ensure that all necessary plugins are correctly set up.
To further optimize your workflow with Vite components, consider implementing the following strategies:
Integrate component libraries like Vuetify (for Vue) or Material-UI (for React) to speed up development with pre-built, customizable components.
Incorporate testing tools such as Jest or Vitest to automatically test your components. Automated testing ensures that your components behave as expected and reduces the risk of introducing bugs.
Use tools like ESLint and Prettier to maintain consistent code style across your components. Consistency improves readability and makes collaboration smoother.
Ensure that your components are accessible to all users by following accessibility best practices. This includes proper ARIA attributes, keyboard navigation support, and sufficient color contrast.
Embracing Vite Components can transform the way you develop web applications, offering unparalleled speed, flexibility, and efficiency. By understanding how Vite manages components and leveraging its powerful features, you can build robust, scalable, and maintainable applications with ease.
You can find answers for commonly asked questions about components.
Yes, Vite is flexible and allows integration with multiple frameworks within the same project, though it requires careful configuration to manage dependencies and build processes effectively.
Vite maintains backward compatibility and provides clear migration paths for updates, ensuring that your existing projects remain stable and can benefit from new features with minimal adjustments.
Vite supports various CSS pre-processors and scoped CSS, allowing you to style your components effectively without global conflicts.