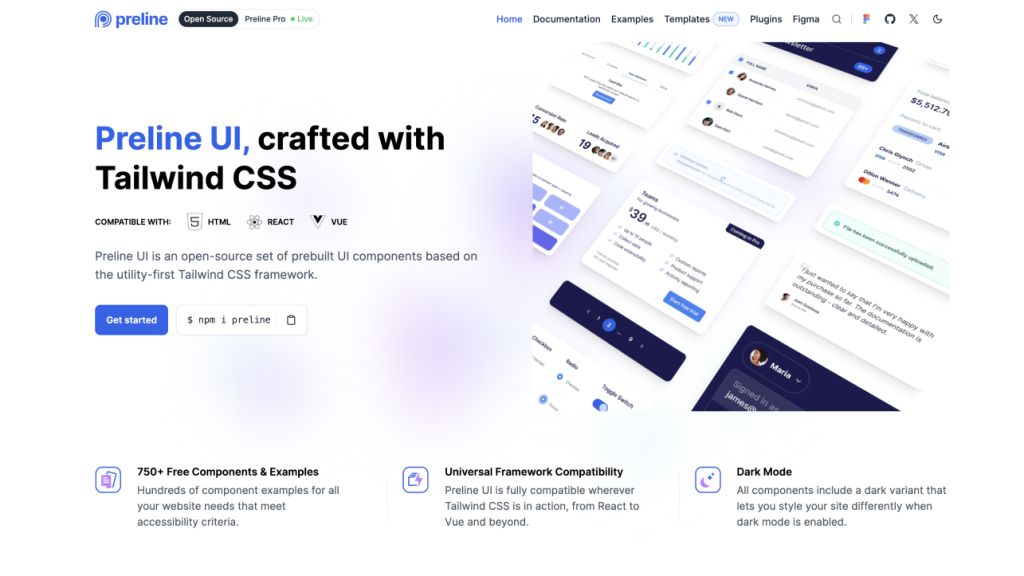
Preline UI
FreeOpen-source set of prebuilt UI components based on the utility-first Tailwind CSS.
Discover the ultimate collection of Nuxt.js components and libraries built on top of Tailwind CSS
Open-source set of prebuilt UI components based on the utility-first Tailwind CSS.
38+ Tailwind Templates & Component Library
450+ UI components for Tailwind CSS
Open-source frontend library built with Tailwind CSS
Discover the most popular Tailwind CSS ui components and elements. Browse top-notch Tailwind components to enhance your development process.
1300+ UI components for Tailwind CSS