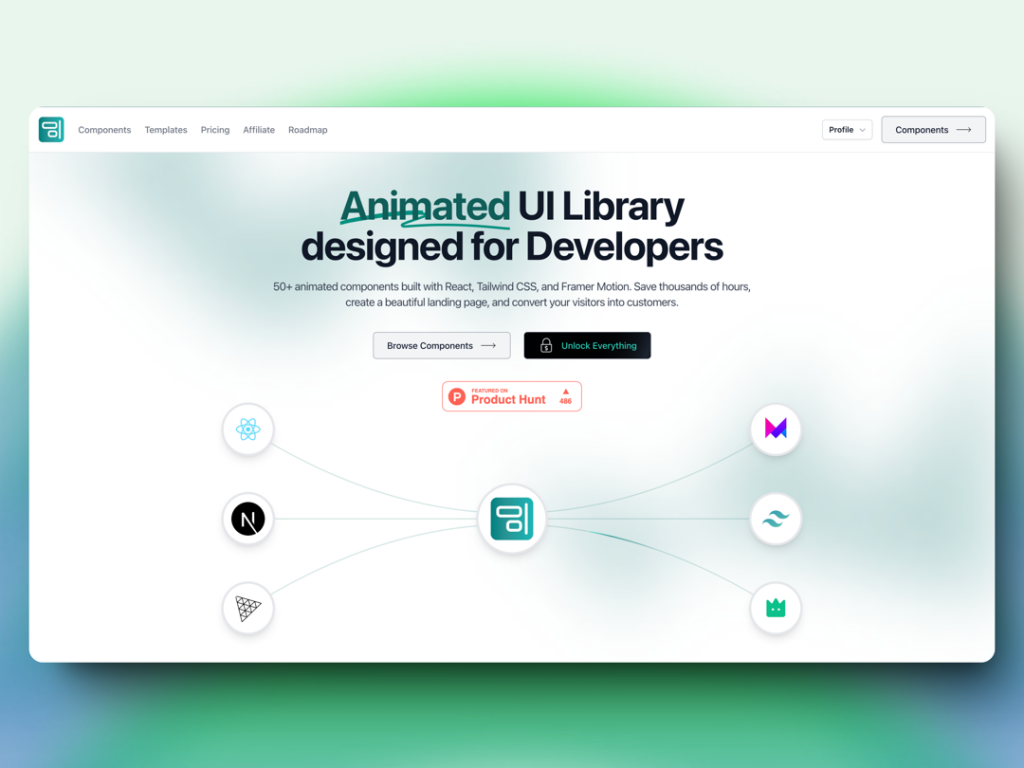
DynaUI
Paid70+ animated React components & templates with Framer Motion
Discover Tailwind video components. Find the best free & premium video UI elements and libraries for your Tailwind CSS projects.
Discover the most popular Tailwind CSS ui components and elements. Browse top-notch Tailwind components to enhance your development process.
1300+ UI components for Tailwind CSS