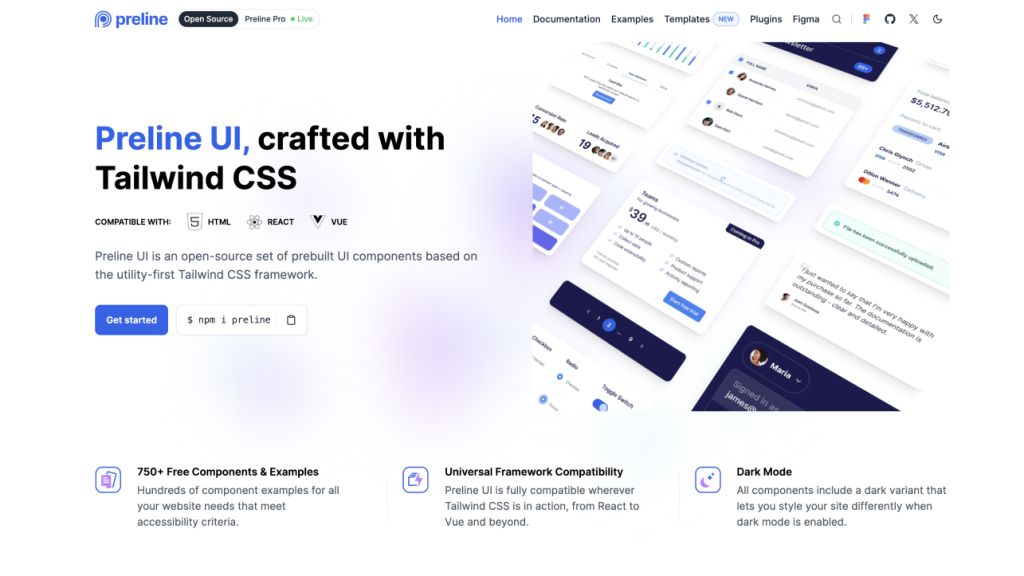
Preline UI
FreeOpen-source set of prebuilt UI components based on the utility-first Tailwind CSS.
Discover Tailwind accordion components to create collapsible sections. Accordion components allow users to expand and collapse content.
Open-source set of prebuilt UI components based on the utility-first Tailwind CSS.
38+ Tailwind Templates & Component Library
Tailwind tree view menu component
Discover the most popular Tailwind CSS ui components and elements. Browse top-notch Tailwind components to enhance your development process.
1300+ UI components for Tailwind CSS
Among the various UI elements, accordions stand out for their ability to present information in a compact and organized manner. Tailwind CSS, renowned for its utility-first approach, offers a seamless way to build elegant accordion components without the need for extensive custom CSS.
Accordions are vertically stacked lists of items, each of which can be "expanded" or "collapsed" to reveal or hide associated content. They are particularly effective in scenarios where you need to display multiple sections of content without overwhelming the user with information all at once. Common use cases include FAQs, settings panels, and content-heavy dashboards.
Tailwind CSS simplifies the process of building responsive and customizable components by providing a vast array of utility classes. With Tailwind, you can style your accordion components directly in your HTML, eliminating the need for writing custom CSS. This leads to faster development cycles and easier maintenance.
Utility-First Approach: Tailwind's utility classes allow for rapid styling without leaving your HTML.
Customization: Easily customize the look and feel to match your design requirements.
Responsive Design: Tailwind's responsive utilities make it straightforward to ensure your accordions look great on all devices.
Consistency: Maintain a consistent design language across your application by reusing utility classes.
Creating a basic accordion with Tailwind involves structuring your HTML with appropriate classes and leveraging JavaScript for interactivity. Here's a high-level overview of the steps involved:
Structure the HTML: Define the accordion's container, items, headers, and content sections.
Style with Tailwind: Use Tailwind's utility classes to style each part of the accordion.
Add Interactivity: Implement JavaScript to handle the expand and collapse actions.
Example Structure:
<div class="accordion">
<div class="accordion-item">
<h2 class="accordion-header">Item 1</h2>
<div class="accordion-content hidden">Content for item 1...</div>
</div>
<!-- Repeat for more items -->
</div>
Note: This is a simplified structure. Detailed implementations would include additional classes and JavaScript for functionality.
Tailwind's flexibility allows you to tailor your accordion components to fit your design needs. Here are some customization ideas:
Headers: Adjust font sizes, colors, padding, and background colors using Tailwind's utility classes.
Content: Control the spacing, typography, and borders of the content sections to match your overall design.
Add smooth animations to enhance user experience when expanding or collapsing sections. Tailwind provides transition utilities that can be applied to height, opacity, and transform properties.
<div class="accordion-content transition-all duration-300 ease-in-out">
<!-- Content here -->
</div>
Tailwind makes it easy to implement responsive designs, including light and dark modes. Utilize the dark:
variant to style your accordion differently based on the user's theme preference.
<div class="accordion-header bg-white dark:bg-gray-800">
<!-- Header content -->
</div>
To take your Tailwind accordion components to the next level, consider incorporating the following advanced features:
Create multi-level accordions by nesting accordion items within the content sections of parent items. This is useful for displaying hierarchical data or complex settings.
Enhance the visual appeal by adding icons or indicators (e.g., chevrons) that change orientation based on the expanded or collapsed state. Tailwind's utility classes can help rotate icons smoothly during state changes.
<span class="transform transition-transform duration-300" :class="{ 'rotate-180': isOpen }">
<!-- Chevron icon -->
</span>
For large amounts of content, consider loading content dynamically when a section is expanded. This approach can improve performance by reducing the initial load time.
Keep It Simple: Avoid overcomplicating the design. A clean and straightforward accordion is often more user-friendly.
Consistent Interaction: Ensure that all accordion items behave consistently, providing a predictable user experience.
Responsive Design: Test your accordions on various screen sizes to ensure they remain functional and visually appealing.
Feedback Mechanisms: Provide visual cues (e.g., changing icons) to indicate the current state of each accordion item.
Tailwind CSS can be seamlessly integrated with popular JavaScript frameworks like React, Vue, and Angular. By combining Tailwind's utility classes with the dynamic capabilities of these frameworks, you can create highly interactive and state-driven accordion components.
In a React application, you can manage the open state of each accordion item using state hooks. Tailwind classes can be conditionally applied based on the state to control visibility and styling.
const AccordionItem = ({ title, content }) => {
const [isOpen, setIsOpen] = useState(false);
return (
<div className="accordion-item">
<button
className="accordion-header"
onClick={() => setIsOpen(!isOpen)}
>
{title}
</button>
{isOpen && <div className="accordion-content">{content}</div>}
</div>
);
};
Note: The above example is a conceptual illustration and not a full code implementation.
You can add hover effects using Tailwind's hover utility classes. For example:
<button class="accordion-header hover:bg-gray-200 transition-colors duration-200">
Header Content
</button>
This changes the background color of the header when hovered over, providing visual feedback to the user.
Tailwind offers transition duration utilities that you can adjust to control animation speed. For example, duration-300
sets the transition to 300ms. Apply these classes to the accordion content for smooth animations.
<div class="accordion-content transition-all duration-300 ease-in-out">
<!-- Content -->
</div>
Tailwind CSS provides a powerful and flexible framework for building accordion components that are both visually appealing and highly functional.
You can find answers for commonly asked questions about components.
Yes, it's possible. By default, accordions might allow multiple items to be open simultaneously. If you want only one item open at a time, you'll need to implement logic in your JavaScript to close other items when a new one is opened.
Absolutely. Tailwind's responsive design utilities enable you to tailor the appearance and behavior of your accordions for different screen sizes. Use breakpoint prefixes like sm:, md:, lg:, and xl: to apply styles conditionally based on the device's screen width.