How to Make Tailwind More Readable
Best practices to organize Tailwind CSS classes
Tailwind CSS has revolutionized the way developers style web applications by offering a utility-first approach. However, the extensive use of utility classes can sometimes lead to cluttered and hard-to-read code. To maintain clarity and efficiency in your projects, it's essential to adopt strategies that enhance the readability of Tailwind CSS classes.
Group Related Classes Together
Organizing classes by their function can significantly improve code readability. By grouping related utility classes, you create a logical structure that makes it easier to understand and maintain your code.
Example:
<!-- Unorganized classes -->
<div class="text-center bg-blue-500 p-4 text-white rounded-md shadow-lg">
<!-- Organized classes -->
<div class="bg-blue-500 text-white p-4 rounded-md shadow-lg text-center">
In the organized example, classes are grouped by their purpose: background color, text color, padding, border-radius, shadow, and text alignment. This methodical arrangement enhances readability and maintainability.
Use Consistent Class Ordering
Maintaining a consistent order for utility classes across your project can further enhance readability. A common approach is to follow the "outside-in" methodology, starting with layout-related classes and moving inward to typography and other styles.
Suggested Order:
Layout & Positioning:
container
,flex
,grid
,absolute
,relative
Box Model:
p-4
,m-4
,w-1/2
,h-64
Typography:
text-center
,text-lg
,font-bold
Visual Styles:
bg-blue-500
,text-white
,shadow-lg
State Variants:
hover:bg-blue-700
,focus:outline-none
By adhering to a consistent class order, you create a predictable pattern that makes scanning and understanding the code more intuitive.
Extract Reusable Components
When you notice recurring patterns of utility classes, it's beneficial to extract them into reusable components. This not only reduces redundancy but also centralizes style management.
Example:
<!-- Repeated pattern -->
<button class="bg-green-500 text-white py-2 px-4 rounded-md hover:bg-green-600">Save</button>
<button class="bg-green-500 text-white py-2 px-4 rounded-md hover:bg-green-600">Submit</button>
<!-- Extracted component -->
<!-- In your CSS file -->
.btn-green {
@apply bg-green-500 text-white py-2 px-4 rounded-md hover:bg-green-600;
}
<!-- In your HTML -->
<button class="btn-green">Save</button>
<button class="btn-green">Submit</button>
By creating a .btn-green
class that applies the necessary Tailwind utilities, you simplify your HTML and make future updates more manageable.
Leverage Tailwind's Configuration File
Tailwind CSS provides a tailwind.config.js
file that allows for extensive customization. By defining custom themes, colors, spacing, and more, you can create a consistent design system that aligns with your project's needs.
Example:
// tailwind.config.js
module.exports = {
theme: {
extend: {
colors: {
brand: {
light: '#3fbaeb',
DEFAULT: '#0fa9e6',
dark: '#0c87b8',
},
},
},
},
};
With this configuration, you can use classes like bg-brand
and text-brand-dark
throughout your project, ensuring consistency and improving readability.
Utilize Editor Extensions
Enhance your development workflow by incorporating editor extensions that provide Tailwind CSS IntelliSense. These tools offer features like class name autocompletion, syntax highlighting, and real-time feedback, which can significantly improve code quality and readability.
Recommended Extensions:
Tailwind CSS IntelliSense: Provides autocompletion and linting for Tailwind classes.
Headwind: Automatically sorts Tailwind classes based on a predefined order.
By integrating these extensions into your development environment, you can maintain a clean and organized codebase with ease.
Implement Responsive Design Thoughtfully
Tailwind CSS excels in facilitating responsive design through its mobile-first approach and responsive utility variants. By applying responsive classes thoughtfully, you can create interfaces that adapt seamlessly across various devices.
Example:
<div class="p-4 md:p-8 lg:p-12">
<h1 class="text-xl md:text-2xl lg:text-3xl">Responsive Heading</h1>
</div>
In this example, padding and text size adjust at different breakpoints (md
and lg
), ensuring optimal readability and aesthetics on all screen sizes.
Avoid Overusing Utility Classes
While Tailwind's utility-first approach is powerful, overusing utility classes can lead to bloated and hard-to-maintain code. Strive for a balance between utility classes and semantic class names to keep your codebase clean.
Example:
<!-- Overuse of utility classes -->
<div class="flex flex-col items-center justify-center p-4 bg-gray-100 rounded-md shadow-md">
<!-- Balanced approach -->
<div class="card">
<!-- Additional content -->
</div>
In the balanced approach, the .card
class can encapsulate the common styles, reducing the number of utility classes in your HTML and enhancing readability.
Use ESLint plugins to format and sort Tailwind CSS classes
Enhancing the readability of Tailwind CSS classes is crucial for maintaining clean and maintainable codebases. Utilizing ESLint plugins can automate the formatting and sorting of these classes, thereby improving code clarity. Here's how to achieve this:
1. Install ESLint and the Tailwind CSS ESLint Plugin
Begin by installing ESLint and the eslint-plugin-tailwindcss
in your project:
npm install eslint eslint-plugin-tailwindcss --save-dev
2. Configure ESLint
Create or update your .eslintrc
configuration file to include the Tailwind CSS plugin and enable the relevant rules:
{
"plugins": ["tailwindcss"],
"rules": {
"tailwindcss/classnames-order": "warn",
"tailwindcss/no-custom-classname": "off"
}
}
This setup enables the classnames-order
rule, which enforces a consistent ordering of Tailwind CSS classes.
3. Integrate Prettier with Tailwind CSS Plugin
For automatic class sorting, integrate Prettier with the official Tailwind CSS plugin:
npm install prettier prettier-plugin-tailwindcss --save-dev
Then, add the plugin to your Prettier configuration file (e.g., .prettierrc
):
{
"plugins": ["prettier-plugin-tailwindcss"]
}
This configuration ensures that Tailwind CSS classes are automatically sorted in a consistent order during formatting.
4. Set Up Automatic Formatting
To maintain consistent formatting, configure your development environment to format files on save. In Visual Studio Code, for example, you can enable "Format on Save" in the settings. Ensure that both ESLint and Prettier extensions are installed and properly configured to work together.
5. Run ESLint and Prettier
Regularly run ESLint and Prettier to lint and format your codebase:
npx eslint . --fix
npx prettier --write .
By following these steps, you can leverage ESLint plugins and Prettier to automatically format and sort Tailwind CSS classes, enhancing the clarity and maintainability of your code.
Regularly Refactor and Review Code
Consistent code reviews and refactoring sessions are vital to maintaining readability in your Tailwind CSS projects. Regularly assess your code to identify areas where utility classes can be consolidated or where components can be extracted.
Tips:
Code Reviews: Encourage team members to review each other's code to ensure adherence to established conventions.
Refactoring Sessions: Schedule periodic refactoring to clean up redundant or outdated classes.
By fostering a culture of continuous improvement, you ensure that your codebase remains clean, efficient, and easy to navigate.
Incorporating these practices into your development workflow will significantly enhance the readability and maintainability of your Tailwind CSS projects. By organizing classes thoughtfully, leveraging Tailwind's configuration capabilities, and utilizing helpful tools, you can create clean, efficient, and scalable codebases.
FAQ
How can I organize Tailwind CSS classes to improve code readability?
Group related classes by their function, such as layout, spacing, typography, and colors, to create a logical and consistent structure.
What tools can assist in maintaining a consistent class order in Tailwind CSS?
Utilize editor extensions like Headwind to automatically sort classes in a predefined, consistent order.
When should I extract utility classes into reusable components in Tailwind CSS?
Extract recurring patterns of utility classes into reusable components to reduce redundancy and centralize style management.
How does the @apply directive enhance code maintainability in Tailwind CSS?
The @apply directive allows you to combine multiple utility classes into a single custom class, simplifying your HTML and improving maintainability.
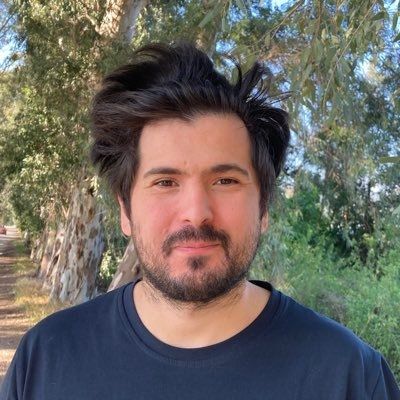
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.