Creating Responsive Login Cards Using React and Tailwind CSS
Learn to create responsive login cards using React and Tailwind CSS for user authentication.
A well-designed login card enhances user experience and sets the tone for the application's overall design. This guide will walk you through creating a responsive login card using React and Tailwind CSS.
Prerequisites
Before you begin, ensure you have the following installed on your development machine:
Node.js and npm: For managing packages and running the React application.
Create React App: To bootstrap your React project.
Tailwind CSS: For utility-first CSS styling.
Setting Up the React Project
1. Create a New React App
Open your terminal and run the following command to create a new React application:
npx create-react-app login-card-app
cd login-card-app
This sets up a new React project in a folder named login-card-app
.
2. Install Tailwind CSS
Install Tailwind CSS and its dependencies via npm:
npm install -D tailwindcss@latest postcss@latest autoprefixer@latest
npx tailwindcss init -p
This creates a tailwind.config.js
file and a postcss.config.js
file.
3. Configure Tailwind CSS
Update the tailwind.config.js
file to include all your React components:
module.exports = {
content: ["./src/**/*.{js,jsx,ts,tsx}"],
theme: {
extend: {},
},
plugins: [],
};
4. Add Tailwind Directives to CSS
Replace the content of src/index.css
with the Tailwind CSS directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
Building the Login Card Component
1. Create the LoginCard Component
In the src
directory, create a new file called LoginCard.js
:
touch src/LoginCard.js
2. Implement the Component
Open LoginCard.js
and add the following code:
import React from "react";
const LoginCard = () => {
return (
<div className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="w-full max-w-sm p-6 bg-white rounded-md shadow-md">
<h2 className="text-2xl font-semibold text-center text-gray-700">Login</h2>
<form className="mt-4">
<div>
<label className="block text-sm text-gray-800" htmlFor="email">
Email
</label>
<input
type="email"
className="w-full mt-2 p-2 border rounded-md focus:outline-none focus:ring-1 focus:ring-blue-600"
id="email"
placeholder="Enter Email"
/>
</div>
<div className="mt-4">
<label className="block text-sm text-gray-800" htmlFor="password">
Password
</label>
<input
type="password"
className="w-full mt-2 p-2 border rounded-md focus:outline-none focus:ring-1 focus:ring-blue-600"
id="password"
placeholder="Enter Password"
/>
</div>
<button
type="submit"
className="w-full mt-6 p-2 bg-blue-600 text-white rounded-md hover:bg-blue-700 transition duration-200"
>
Login
</button>
</form>
</div>
</div>
);
};
export default LoginCard;
This component creates a centered login card with email and password fields.
3. Update the App Component
In src/App.js
, import and render the LoginCard
component:
import React from "react";
import LoginCard from "./LoginCard";
function App() {
return (
<div>
<LoginCard />
</div>
);
}
export default App;
Making the Login Card Responsive
Tailwind CSS automatically handles responsiveness through its utility classes. However, you can further enhance responsiveness using responsive prefixes.
1. Adjust for Small Screens
Modify the input fields in LoginCard.js
:
<input
className="w-full mt-2 p-2 text-sm sm:text-base border rounded-md focus:outline-none focus:ring-1 focus:ring-blue-600"
// other props
/>
2. Test Responsiveness
Run your application using:
npm start
Open the application in a browser and resize the window to see how the login card adjusts to different screen sizes.
Conclusion
You've successfully created a responsive and accessible login card using React and Tailwind CSS. This component can be customized further to fit the branding and functionality of your application, providing users with a seamless authentication experience.
FAQ
Can I use this login card component in a Next.js application?
Yes. Since Next.js supports React components, you can integrate the login card into a Next.js app. Ensure you configure Tailwind CSS according to Next.js requirements by following their specific setup instructions.
How do I style the login card to match my application's theme?
Tailwind CSS allows for extensive customization through its configuration file. You can customize colors, fonts, and other styles by editing the tailwind.config.js file. Additionally, you can apply custom classes directly to your components to override default styles.
Is Tailwind CSS compatible with CSS-in-JS libraries?
Yes, Tailwind CSS can be used alongside CSS-in-JS libraries like styled-components or emotion. However, it's important to manage the styling to prevent conflicts and ensure that styles are applied as intended.
How do I deploy my React application with this login card component?
You can deploy your React application using hosting services like Netlify, Vercel, or GitHub Pages. Build your application using npm run build and follow the deployment instructions provided by your chosen hosting service
How can I add form validation to the login card?
Form validation can be implemented using React's state management along with conditionally rendering validation messages. For more advanced validation, consider using libraries like Formik or React Hook Form, which offer robust solutions for handling form state and validation.
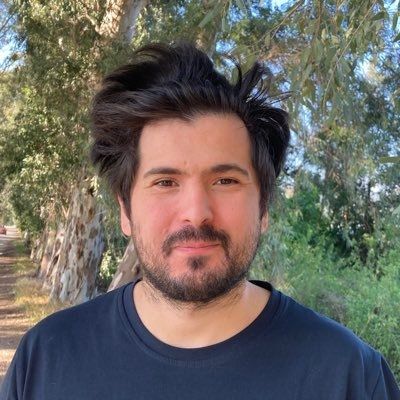
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.