Using CSS-in-JS Libraries with Tailwind CSS
Find out whether CSS-in-JS and Tailwind CSS can be used together in your projects.
You can combine Tailwind CSS with CSS-in-JS solutions like Emotion or Styled Components, especially in React projects.
Example with Styled Components:
import styled from 'styled-components';
const Button = styled.button`
@apply bg-blue-500 text-white font-bold py-2 px-4 rounded;
&:hover {
@apply bg-blue-700;
}
`;
function App() {
return <Button>Click Me</Button>;
}
Note that you need to configure your build setup to handle @apply
in your CSS-in-JS library.
In the ever-evolving world of web development, styling solutions are constantly emerging to improve efficiency and maintainability. Two popular approaches are Tailwind CSS and CSS-in-JS libraries. Tailwind CSS is a utility-first CSS framework that provides low-level utility classes, while CSS-in-JS libraries like styled-components and Emotion allow you to write CSS directly within your JavaScript code.
But did you know you can combine these two methodologies to get the best of both worlds? In this blog post, we'll explore how to integrate CSS-in-JS libraries with Tailwind CSS to create a powerful and flexible styling system for your projects.
Why Combine Tailwind CSS with CSS-in-JS?
Before diving into the how, let's understand the why.
Benefits of Tailwind CSS
Utility-First Approach: Tailwind offers a comprehensive set of utility classes that speed up development by reducing the need to write custom CSS.
Consistency: Promotes design consistency across your application.
Customization: Highly customizable through configuration files.
Benefits of CSS-in-JS
Scoped Styles: Styles are scoped to components, preventing CSS conflicts.
Dynamic Styling: Easily apply styles based on component props and state.
Maintainability: Keep styles close to the components they affect.
Combined Advantages
By combining Tailwind CSS with CSS-in-JS, you can:
Use Tailwind's utility classes within your styled components.
Leverage dynamic styling capabilities of CSS-in-JS.
Maintain a consistent design system with the flexibility of component-based styling.
Setting Up the Environment
1. Install Dependencies
First, ensure you have the necessary packages installed. For this example, we'll use styled-components.
npm install tailwindcss styled-components
2. Configure Tailwind CSS
Initialize Tailwind CSS by creating a tailwind.config.js
file:
npx tailwindcss init
3. Create a Global Stylesheet
Since we're using CSS-in-JS, we need to import Tailwind's base styles. Create a GlobalStyle
component:
// GlobalStyle.js import { createGlobalStyle } from 'styled-components'; import 'tailwindcss/tailwind.css'; const GlobalStyle = createGlobalStyle` /* Your global styles here */ `; export default GlobalStyle;
Using Tailwind Classes in Styled Components
You can use Tailwind's utility classes within your styled components by interpolating the class names:
import styled from 'styled-components'; const Button = styled.button` @apply bg-blue-500 text-white font-bold py-2 px-4 rounded; &:hover { @apply bg-blue-700; } `;
Note: The @apply
directive is used in Tailwind CSS to include utility classes in your CSS.
Dynamic Styling with Props
CSS-in-JS shines when it comes to dynamic styling. You can adjust styles based on props:
const Button = styled.button` @apply text-white font-bold py-2 px-4 rounded; background-color: ${({ primary }) => (primary ? 'bg-blue-500' : 'bg-gray-500')}; &:hover { background-color: ${({ primary }) => (primary ? 'bg-blue-700' : 'bg-gray-700')}; } `;
Best Practices
Limit Scope: Use Tailwind classes for common utilities and CSS-in-JS for component-specific styles.
Theming: Leverage CSS-in-JS theming capabilities to manage your design system.
Performance: Be cautious of the generated CSS size; optimize by purging unused styles.
Conclusion
Combining Tailwind CSS with CSS-in-JS libraries offers a robust and flexible approach to styling in modern web applications. You get the utility and consistency of Tailwind with the dynamic and scoped styling of CSS-in-JS. Give it a try in your next project to enhance your development workflow.
FAQ
Can I use other CSS-in-JS libraries with Tailwind CSS?
Yes, you can use other libraries like Emotion or Linaria. The integration process is similar, but you should consult the specific library's documentation for details.
How does this setup affect performance?
Using both Tailwind CSS and CSS-in-JS can increase the size of your CSS bundle. To mitigate this, make sure to purge unused Tailwind classes in production and optimize your CSS-in-JS usage.
Is it necessary to use the @apply directive?
While not mandatory, @apply allows you to include Tailwind utility classes within your styled components, keeping your code DRY and consistent with Tailwind's methodology.
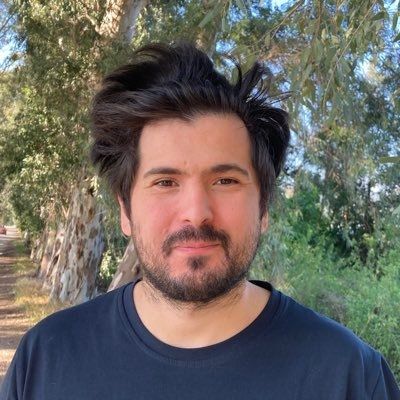
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.