Tailwind Popup Guide
Build responsive Tailwind popups in minutes
Pop‑ups—whether they’re quick alerts, confirmation modals, or full‑screen marketing offers—are everywhere on the modern web. Tailwind CSS makes styling these overlays ridiculously fast, but many devs still wrestle with the little details: trapping focus, wiring JavaScript triggers, and keeping the design lightweight.
This post walks you through a step‑by‑step plan to build Tailwind popups, modals, and alerts that are accessible, tiny, and easy to tweak.
Why bother with a custom Tailwind popup?
Speed: Utility classes mean no hunting for the right selector; spacing, colors, and shadows are just class strings.
Control: You aren’t locked into a heavyweight plugin—only the HTML you write plus a few lines of vanilla JS or Alpine.
Accessibility (a11y): When you add the right ARIA roles, screen‑reader users get the same clarity as sighted users.
Consistency: Tailwind’s design tokens keep every alert or marketing banner on‑brand without a design file hunt.
Prerequisites & Quick Setup
Either add Tailwind via CDN for a throwaway demo or install it in your build pipeline if you’re using Vite, Next, or Laravel. To stay concise we’ll use the CDN route:
<script src="https://cdn.tailwindcss.com"></script>
Drop that in the <head>
of a blank HTML file and you’re ready to roll.
Folder structure
index.html
app.js // optional, tiny vanilla JS helper
Nothing fancy—we’ll store the modal markup in the HTML so you can see everything in one place.
Step 1 – Skeleton HTML for the modal
A modal is just a positioned div that sits above the rest of your content. Flowbite’s example keeps things simple with a fixed overlay and a centered panel.
<!-- Overlay -->
<div id="overlay"
class="fixed inset-0 bg-black/50 flex items-center justify-center hidden">
<!-- Dialog -->
<div id="dialog"
class="bg-white w-full max-w-lg rounded-lg shadow-xl p-6
focus:outline-none"
role="dialog"
aria-modal="true"
aria-labelledby="dialogTitle">
<h2 id="dialogTitle" class="text-xl font-bold mb-4">
Example Tailwind Popup
</h2>
<p class="mb-6">
This is a lightweight modal built with pure Tailwind utilities.
</p>
<button id="closeBtn"
class="mt-2 inline-flex justify-center rounded-md
bg-indigo-600 px-4 py-2 text-white hover:bg-indigo-700
focus-visible:ring-2 focus-visible:ring-offset-2
focus-visible:ring-indigo-500">
Close
</button>
</div>
</div>
What’s going on?
The overlay uses
fixed inset-0 bg-black/50
to dim the background—no extra CSS file needed.The dialog gains
role="dialog"
andaria-modal="true"
so assistive tech knows it’s a modal window.hidden
on the overlay keeps the modal invisible until you toggle it with JS.
Step 2 – Vanilla JavaScript triggers
All you need is a click listener to toggle that hidden
class plus a keydown listener for the Escape key. SitePoint and Stack Overflow both show minimal patterns for this.
// app.js
const overlay = document.getElementById("overlay");
const openBtn = document.getElementById("openModalBtn");
const closeBtn = document.getElementById("closeBtn");
const dialog = document.getElementById("dialog");
// open modal
openBtn.addEventListener("click", () => {
overlay.classList.remove("hidden");
dialog.focus(); // send focus inside
});
// close modal (button)
closeBtn.addEventListener("click", closeModal);
// close modal (Esc key)
window.addEventListener("keydown", e => {
if (e.key === "Escape" && !overlay.classList.contains("hidden")) {
closeModal();
}
});
function closeModal() {
overlay.classList.add("hidden");
openBtn.focus(); // return focus
}
That’s barely 30 lines—no dependencies, and it hits two accessibility must‑haves: focus trapping into the modal on open and out of it when you close.
💡 AlpineJS shortcut: If you’re already using Alpine, a single
x-data="{open:false}"
wrapper plusx-show="open"
andx-on:keydown.escape.window="open=false"
accomplishes the same thing. Livewire users follow an identical pattern.
Step 3 – Make it accessible
Role & aria‑modal – Already added above. Screen readers now know underlying content is inert.
Keyboard focus – Send focus into the first interactive element (
dialog.focus()
in the snippet). Headless UI’s Dialog component does this for you out of the box.Escape to close – Done with our keydown listener; all major a11y guidelines recommend it.
Return focus – Move it back to the button that opened the modal so screen‑reader flow resumes logically.
Label & description –
aria-labelledby="dialogTitle"
ties the heading to the dialog, improving screen‑reader context.
If you need advanced trapping (e.g., Tab cycling that never escapes the dialog), drop in Headless UI or Tiny‑Focus‑Trap and keep your custom styling. Headless UI ships completely unstyled but handles focus for you.
Step 4 – Styling variations
Alert‑style popup
Swap the width class for max-w-sm
, tweak colors, and you’ve got a quick “Are you sure?” prompt like Tailwind UI’s alert modal.citeturn0search5
<div class="bg-red-50 border border-red-200 text-red-800
rounded-lg p-4" role="alert">
<strong class="font-bold">Heads up!</strong>
<span class="block sm:inline ml-2">Something went wrong.</span>
</div>
<iframe class="language-embed" width="100%" height="300" src="//jsfiddle.net/tailkits/2rxd3w4z/embedded/html,result/" frameborder="0" loading="lazy" allowtransparency="true" allowfullscreen="true"></iframe>
Marketing offer
Flowbite’s marketing block wraps the dialog in sm:h-auto
plus bold imagery for those newsletter sign‑ups. Just replace the paragraph with an image and form fields—same toggle logic applies.
Full‑screen drawer
Add sm:items-start
to the overlay flex container, stretch height to h-full
, and animate slide‑in with Tailwind’s transition and transform utilities. Now you have a mobile‑friendly bottom sheet.
Step 5 – Sprinkle animation (optional)
Tailwind’s animate-fade-in
isn’t built‑in, but you can add a custom keyframe in your tailwind.config.js:
module.exports = {
theme: {
extend: {
keyframes: {
'fade-in': { '0%': {opacity:0}, '100%': {opacity:1} },
},
animation: {
'fade-in': 'fade-in 200ms ease-out',
},
}
}
}
Then add animate-fade-in
to the dialog for a subtle entrance.
Performance notes
Tailwind styles are “just classes,” so your CSS bundle doesn’t blow up if you purge unused classes in production. That keeps your popup implementation under 10 KB gzipped, which is lighter than most UI kits. Tailwind’s official docs outline how PurgeCSS removes dead styles automatically.
Pro tips
Inline SVG icons: Keep them as
<svg>
tags inside the button; they inherit Tailwind color classes automatically.Scrolling background: Add
overflow-hidden
on<body>
while the modal is open if long pages bleed through.Multiple modals on one page: Give each
dialog
unique IDs and listeners; or delegate withdata-modal-target
attributes to scale cleanly.Framework integration: React/Next users might prefer Headless UI’s
<Dialog>
for battle‑tested focus trapping; Vue 3 ports exist too.Don’t forget contrast: Material Tailwind’s dialog page demonstrates compliant color choices; borrow their palette or check with an a11y tool.
Conclusion
And that’s it—popups without the pain. By mixing Tailwind utility classes with a dash of vanilla JS (or Alpine/Headless UI if you want the heavy a11y lifting done for you), you can spin up alerts, marketing banners, and full modals in minutes. Remember to respect users with escape‑key closing, focus trapping, and meaningful labels. Happy building!
FAQ
How do I trigger a Tailwind popup on page load?
Set overlay.classList.remove('hidden') in a DOMContentLoaded handler or add x-init="open=true" if you’re using Alpine—no extra libraries needed.
Can I use Tailwind popups without JavaScript at all?
Sort of—use the <details> element for a no‑JS disclosure, but for true overlay behaviour you’ll still need minimal JS to trap focus and disable background scrolling.
What’s the easiest way to add focus trapping?
Install Headless UI and wrap your content with its <Dialog> component; it manages focus, aria‑modal, and escape handling automatically.
Do popups hurt SEO?
Not when implemented responsibly—hide them with CSS/JS rather than removing them from the DOM, and make sure they appear after user interaction instead of blocking the initial content and Google will ignore them.
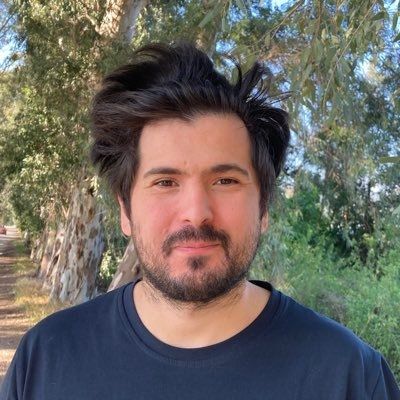
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.