Resolve Tailwind CSS Class Conflicts Using tailwind-merge
How to use tailwind-merge to combine Tailwind CSS
When working with Tailwind CSS, there’s no shortage of utility classes at your disposal. Tailwind provides a vast collection of classes for nearly every conceivable styling need, ranging from spacing and typography to layout, interaction states, and even responsive variants. This abundance of options is one of its greatest strengths. However, as your project grows and you start reusing or programmatically generating classes, you may bump into scenarios where multiple classes from different sources end up colliding. These conflicts can lead to unexpected outcomes or redundant code that’s tough to maintain.
This is where tailwind-merge steps onto the stage. This tiny yet powerful package lets you blend multiple Tailwind class strings into one unified collection, automatically resolving conflicts so that your final output makes sense. No more sifting through long strings of classes to figure out which takes priority—tailwind-merge does it for you.
In this post, we’ll explore what tailwind-merge does, how it works, and how you can integrate it into your workflow. We’ll also look into use cases, performance considerations, advanced customization, and a few best practices for making your codebase cleaner, leaner, and more maintainable.
Understanding Class Conflicts in Tailwind
Before diving into tailwind-merge, let’s step back and consider the problem it aims to solve. Tailwind encourages composition of small, single-purpose utility classes. For example, setting a text color might involve text-gray-700
, while adding a hover state could be hover:text-gray-900
. A button might have a base set of classes, but depending on a condition, you might append classes that tweak its appearance. Over time, your class strings can become large and conditionally generated.
In a straightforward scenario, you might have code like this:
const baseClasses = "bg-blue-500 text-white px-4 py-2 rounded";
const disabledClasses = isDisabled ? "opacity-50 cursor-not-allowed" : "";
const finalClasses = `${baseClasses} ${disabledClasses}`;
This works fine as long as you control the order and know what’s being appended. But what if you dynamically generate classes in multiple places, or combine multiple sets of classes each with their own logic? If you’re not careful, you might end up with something like:
const classes = "bg-blue-500 text-white px-4 py-2 rounded bg-red-500"
Now you have a conflict: two background colors (blue and red) in the same string. CSS will prioritize the one that appears last, which might not be the intended behavior. In more complex examples, you may end up with multiple contradicting classes that make debugging a chore.
tailwind-merge helps ensure that when classes collide, the “winning” class is clearly defined according to Tailwind’s rules, letting you craft dynamic class sets without worrying about unintended overrides.
What is tailwind-merge?
tailwind-merge is a utility that takes multiple Tailwind class strings and merges them into a single, conflict-free string. It uses Tailwind’s own configuration and normalization logic to figure out which classes belong to the same group and which of them should prevail.
Here’s a simple example:
import { twMerge } from 'tailwind-merge';
const finalClasses = twMerge("bg-blue-500 text-white", "bg-red-500 p-4");
console.log(finalClasses);
This will print:
"bg-red-500 text-white p-4"
Note how bg-blue-500
got replaced by bg-red-500
since both are background color classes, and the second one takes priority. This is precisely what you want, especially in scenarios where classes come from various sources and conditions.
Installing tailwind-merge
Getting started is easy. tailwind-merge is available as a package on npm, so all you need to do is add it to your project:
npm install tailwind-merge
If you’re using Yarn:
yarn add tailwind-merge
Once installed, you can import and use it anywhere you generate class strings. This makes it perfect for React or Vue components where you’re dynamically building className or class attributes based on props or state.
Basic Usage Examples
Let’s explore a few straightforward examples to illustrate how tailwind-merge handles different conflicts.
Multiple Spacing Classes:
twMerge("p-2 p-4");
// Result: "p-4"
The p-4
class takes precedence over p-2
, leaving you with a single final class.
Font Sizing and Weights:
twMerge("text-lg font-medium", "font-bold text-sm");
// Result: "text-sm font-bold"
Classes that define a single conceptual property (like font size or weight) are merged so the latter replaces the former.
Combining Conditional Classes:
const base = "px-6 py-3 bg-blue-500 text-white";
const hoverState = isHovered ? "bg-blue-700" : "";
const disabledState = isDisabled ? "opacity-50 cursor-not-allowed" : "";
const final = twMerge(base, hoverState, disabledState);
Here, tailwind-merge ensures that if isHovered
and isDisabled
logic tries to add conflicting classes, you’ll still end up with a sensible result. It’s all about finalizing a cohesive class string that respects Tailwind’s utility order.
Handling More Complex Classes
Some Tailwind classes can overlap in subtle ways. Consider variants like hover:
, focus:
, md:
, lg:
, and so forth. When merging classes, tailwind-merge also understands these variants and knows how to handle them.
For instance, if you merge:
twMerge("hover:bg-blue-500", "hover:bg-red-500")
// Result: "hover:bg-red-500"
Similarly, if you have responsive classes:
twMerge("md:text-left lg:text-center", "md:text-right lg:text-left")
// Result: "md:text-right lg:text-left"
tailwind-merge respects the boundaries of these variants, ensuring that the last specified variant class for the same property wins.
Integration With Popular Frameworks
While tailwind-merge can be used in any JavaScript-based project, it’s especially helpful in UI libraries like React, Vue, or Svelte. Components often receive props that determine certain classes, and merging them reliably can prevent a host of styling headaches.
React Example:
import React from 'react';
import { twMerge } from 'tailwind-merge';
const Button = ({ primary, disabled, children }) => {
const base = "px-4 py-2 rounded";
const variant = primary ? "bg-blue-500 text-white" : "bg-gray-200 text-black";
const state = disabled ? "opacity-50 cursor-not-allowed" : "hover:bg-blue-600";
return (
<button className={twMerge(base, variant, state)}>
{children}
</button>
);
};
This example shows how you can confidently stack classes derived from different boolean props without worrying about which one might override another. The final string is always clean and predictable.
Performance Considerations
Merging classes might sound like extra overhead, but tailwind-merge is surprisingly efficient. It’s written with performance in mind, handling class strings in a way that’s optimized for speed. While you shouldn’t notice any significant slowdown in development mode, it’s still worth being mindful of how often you call twMerge
.
If you have computationally heavy logic generating class strings on every render, consider memoizing results or integrating twMerge
only at points where the classes meaningfully change. For many applications, calling twMerge
on each render is perfectly fine, but if performance becomes a concern in a highly dynamic interface, caching results can be a wise move.
Advanced Customization
Out of the box, tailwind-merge comes preconfigured with Tailwind’s default class groups and standard conflict resolution logic. But what if your project has custom Tailwind config values, or maybe even custom utilities?
tailwind-merge offers advanced APIs for customization, allowing you to redefine which classes belong to which groups and how conflicts are resolved. This is especially useful if you’ve extended Tailwind’s default theme with additional classes, or if you are using plugins that create new types of utilities.
You can define custom config objects to override the defaults. For example:
import { createTailwindMerge } from 'tailwind-merge';
const customTwMerge = createTailwindMerge({
// Custom configuration here
});
With this custom instance, you can specify how new classes should be merged. This level of control ensures that as your Tailwind setup becomes more intricate, tailwind-merge remains aligned with your chosen conventions.
Debugging and Maintenance Tips
When working with complex styling scenarios, debugging can become tricky. Here are a few tips to maintain clarity and sanity:
Keep Base Classes Minimal: Start with a small set of base classes that handle the foundational styling. Additional conditions should layer on top. By keeping base classes simple, you minimize unexpected overrides.
Isolate Variant Logic: Move variant logic (such as responsive breakpoints, hover states, or condition-based overrides) into separate variables or helper functions. This compartmentalization makes it clearer why certain classes appear or vanish.
Utilize tailwind-merge Early: Don’t wait until you have a mess of classes before starting to use
twMerge
. Incorporate it into your workflow as soon as you start seeing dynamically combined classes. This preemptive approach can reduce complexity down the road.Test Class Outputs: For critical components, consider logging out the final merged classes in development or even writing simple snapshot tests. Ensuring that the final class strings match expectations can catch issues early.
Common Use Cases
Theming and Dark Mode:
If you’re supporting dark mode or multiple themes, you might toggle entire sets of classes. Without tailwind-merge, you might repeat a lot of logic or suffer from conflicts. With it, you can have a base theme class and conditionally applied dark classes that automatically reconcile with one another.
Conditional Rendering in Frameworks:
When using frameworks like Next.js, you might dynamically load components or styles. If these components define classes that need to work together, merging ensures the final UI looks right without having to meticulously track which classes won out.
Complex Component Libraries:
If you’re building a design system or component library on top of Tailwind, you might offer a wide array of props that tweak your components. Each prop may introduce new classes. Rather than carefully crafting a large className
string, you can rely on merging logic so your code stays tidy even as you add more variations.
Conclusion
As your Tailwind CSS project matures, there will almost inevitably come a time when you’re juggling multiple sets of classes. Without a system in place, it’s easy to fall into a tangle of conflicting styles that obscure rather than clarify your intended design. tailwind-merge provides a streamlined solution: simply pass in all your class sets and let it produce a final, coherent string. The result is cleaner code, easier debugging, and a more robust styling approach.
From handling basic utility conflicts to customizing merging logic for a heavily extended theme, tailwind-merge offers flexibility and peace of mind. It fits smoothly into most build pipelines and is easy to integrate in React, Vue, and other libraries.
FAQ
Can I rely on tailwind-merge to handle multiple breakpoints, hover states, or focus states?
Yes. It understands variants and merges them based on Tailwind’s established rules.
Do I have to worry about the order of classes I pass into tailwind-merge?
No. tailwind-merge automatically determines which class should take precedence, ensuring the last defined conflicting utility wins.
Is using tailwind-merge going to slow down my application?
Typically no. It’s optimized for speed and can be used frequently, though you can cache results if performance becomes a concern.
Will tailwind-merge work if I add custom utilities or extend my Tailwind configuration?
Yes. You can configure it to align with your custom setup, allowing it to correctly merge any new or extended classes.
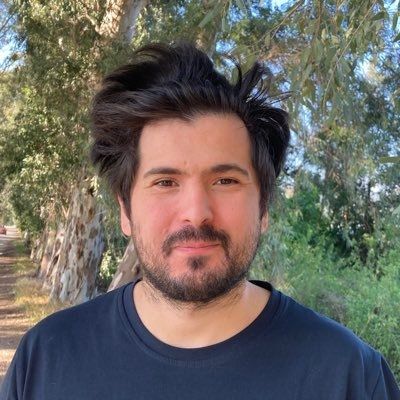
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.