Tailwind CSS Carousel with React: A Step-by-Step Guide
Learn how to create a Tailwind CSS carousel with React
Hey web developers!
Carousels are perfect for showcasing images, products, or any content in a rotating display, making your website more lively. While they can be tricky to build, we’ve got you covered!
This guide will take you step by step through creating a Tailwind CSS carousel with React. Ready to start? Let’s go!
Setting Up Your Project
Before we dive into the code, let’s get our environment ready.
Install Node.js and npm
First things first, make sure you have Node.js and npm (Node Package Manager) installed. If you haven’t, download and install them from Node.js.
Create a React App
Next, create a new React project using Create React App. Open your terminal and run:
npx create-react-app tailwind-carousel
cd tailwind-carousel
Install Tailwind CSS
Now, let’s install Tailwind CSS. Run the following commands in your project directory:
npm install -D tailwindcss
npx tailwindcss init
This will create a tailwind.config.js
file in your project.
Configure Tailwind CSS
Open the tailwind.config.js
file and replace the content with:
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Next, create a tailwind.css
file in the src
directory and add the following:
@tailwind base;
@tailwind components;
@tailwind utilities;
Finally, import this CSS file in your src/index.js
:
import './tailwind.css';
Great! Now your React project is set up with Tailwind CSS.
Building the Carousel Component
Now, let’s get to the fun part—building the carousel!
Create the Carousel Component
Create a new file named Carousel.js
in the src
directory. This file will contain our carousel component.
import React, { useState } from 'react';
const Carousel = ({ images }) => {
const [currentIndex, setCurrentIndex] = useState(0);
const nextSlide = () => {
setCurrentIndex((prevIndex) => (prevIndex + 1) % images.length);
};
const prevSlide = () => {
setCurrentIndex((prevIndex) => (prevIndex - 1 + images.length) % images.length);
};
return (
<div className="relative w-full max-w-3xl mx-auto">
<div className="overflow-hidden relative h-64">
{images.map((image, index) => (
<div
key={index}
className={`absolute inset-0 transition-transform transform ${
index === currentIndex ? 'translate-x-0' : 'translate-x-full'
}`}
>
<img src={image} alt={`Slide ${index}`} className="w-full h-full object-cover" />
</div>
))}
</div>
<button
className="absolute top-1/2 left-0 transform -translate-y-1/2 bg-gray-800 text-white p-2"
onClick={prevSlide}
>
Prev
</button>
<button
className="absolute top-1/2 right-0 transform -translate-y-1/2 bg-gray-800 text-white p-2"
onClick={nextSlide}
>
Next
</button>
</div>
);
};
export default Carousel;
Styling the Carousel
Tailwind CSS makes styling a breeze. Let’s add some styles to make our carousel look good.
Container Styling: The container has a relative positioning to hold the slides and buttons.
Slide Styling: Each slide has an absolute positioning and a transition for smooth sliding.
Button Styling: The navigation buttons are styled using Tailwind classes for positioning, background, and padding.
Integrating the Carousel Component
Now, let’s integrate the Carousel
component into our main app.
Update App.js
Open src/App.js
and update it as follows:
import React from 'react';
import Carousel from './Carousel';
const App = () => {
const images = [
'<https://via.placeholder.com/800x400.png?text=Slide+1>',
'
'<https://via.placeholder.com/800x400.png?text=Slide+2>',
'<https://via.placeholder.com/800x400.png?text=Slide+3>',
];
return (
<div className="App">
<header className="App-header">
<h1 className="text-4xl font-bold text-center my-6">Tailwind CSS Carousel with React</h1>
</header>
<main className="flex justify-center items-center min-h-screen bg-gray-100">
<Carousel images={images} />
</main>
</div>
);
};
export default App;
Running the Project
Now, let's run our project to see the carousel in action. In your terminal, run:
npm start
This will start the development server and open your project in the browser. You should see a carousel with three slides and navigation buttons. Congratulations! You've just built a responsive carousel using Tailwind CSS and React.
Adding More Features
Want to take your carousel to the next level? Here are some additional features you can add:
Auto-Slide Functionality
Add automatic sliding after a specific interval. Modify the Carousel.js
file to include a useEffect
hook that changes the slide at a set interval.
import React, { useState, useEffect } from 'react';
const Carousel = ({ images, autoSlide = true, autoSlideInterval = 3000 }) => {
const [currentIndex, setCurrentIndex] = useState(0);
useEffect(() => {
if (autoSlide) {
const slideInterval = setInterval(() => {
setCurrentIndex((prevIndex) => (prevIndex + 1) % images.length);
}, autoSlideInterval);
return () => clearInterval(slideInterval);
}
}, [autoSlide, autoSlideInterval, images.length]);
const nextSlide = () => {
setCurrentIndex((prevIndex) => (prevIndex + 1) % images.length);
};
const prevSlide = () => {
setCurrentIndex((prevIndex) => (prevIndex - 1 + images.length) % images.length);
};
return (
<div className="relative w-full max-w-3xl mx-auto">
<div className="overflow-hidden relative h-64">
{images.map((image, index) => (
<div
key={index}
className={`absolute inset-0 transition-transform transform ${
index === currentIndex ? 'translate-x-0' : 'translate-x-full'
}`}
>
<img src={image} alt={`Slide ${index}`} className="w-full h-full object-cover" />
</div>
))}
</div>
<button
className="absolute top-1/2 left-0 transform -translate-y-1/2 bg-gray-800 text-white p-2"
onClick={prevSlide}
>
Prev
</button>
<button
className="absolute top-1/2 right-0 transform -translate-y-1/2 bg-gray-800 text-white p-2"
onClick={nextSlide}
>
Next
</button>
</div>
);
};
export default Carousel;
Adding Pagination Dots
Add pagination dots to indicate the current slide. Update the Carousel.js
file to include pagination dots.
import React, { useState, useEffect } from 'react';
const Carousel = ({ images, autoSlide = true, autoSlideInterval = 3000 }) => {
const [currentIndex, setCurrentIndex] = useState(0);
useEffect(() => {
if (autoSlide) {
const slideInterval = setInterval(() => {
setCurrentIndex((prevIndex) => (prevIndex + 1) % images.length);
}, autoSlideInterval);
return () => clearInterval(slideInterval);
}
}, [autoSlide, autoSlideInterval, images.length]);
const nextSlide = () => {
setCurrentIndex((prevIndex) => (prevIndex + 1) % images.length);
};
const prevSlide = () => {
setCurrentIndex((prevIndex) => (prevIndex - 1 + images.length) % images.length);
};
return (
<div className="relative w-full max-w-3xl mx-auto">
<div className="overflow-hidden relative h-64">
{images.map((image, index) => (
<div
key={index}
className={`absolute inset-0 transition-transform transform ${
index === currentIndex ? 'translate-x-0' : 'translate-x-full'
}`}
>
<img src={image} alt={`Slide ${index}`} className="w-full h-full object-cover" />
</div>
))}
</div>
<button
className="absolute top-1/2 left-0 transform -translate-y-1/2 bg-gray-800 text-white p-2"
onClick={prevSlide}
>
Prev
</button>
<button
className="absolute top-1/2 right-0 transform -translate-y-1/2 bg-gray-800 text-white p-2"
onClick={nextSlide}
>
Next
</button>
<div className="absolute bottom-0 left-0 right-0 flex justify-center mb-4">
{images.map((_, index) => (
<div
key={index}
className={`w-2 h-2 rounded-full mx-1 ${index === currentIndex ? 'bg-gray-800' : 'bg-gray-400'}`}
onClick={() => setCurrentIndex(index)}
/>
))}
</div>
</div>
);
};
export default Carousel;
Conclusion
And there you have it!
You’ve successfully created a dynamic, responsive carousel using Tailwind CSS and React. This guide should give you a solid foundation to build upon and customize according to your project’s needs.
Remember, practice makes perfect. Happy coding!
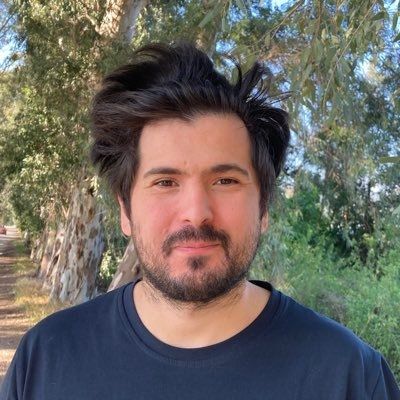
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.