Tailwind Child Selector Guide
Use Tailwind's child selector to efficiently style direct descendants.
Tailwind CSS is all about helping you write less and do more. One of the coolest tricks it offers is the child selector syntax, which makes styling direct descendants a breeze. In this guide, we’re diving deep into how you can harness this powerful feature to boost your CSS productivity, keep your HTML neat, and write code that’s both easy to maintain and fun to work with.
What Are Child Selectors in Tailwind CSS?
Before we jump into the details, let’s start with the basics. In CSS, a child selector targets the direct children of a parent element. Think of it as telling your stylesheet, “Hey, style these elements that sit right under this container, but not the ones nested deeper.”
In traditional CSS, you might write something like:
.parent > .child {
/* styles */
}
But when working with Tailwind CSS, things get a bit more interesting. Tailwind lets you use an arbitrary value syntax to mimic this behavior directly in your HTML. This means you can add classes that specifically target the immediate children of an element, keeping your markup cleaner and your styles more predictable.
Why Use Child Selectors?
Using child selectors is a game changer for several reasons:
Less Repetition: Instead of applying the same classes to multiple elements, you apply them once to the parent. It’s like using a magic wand to style all the direct kids in one go!
Cleaner HTML: Your HTML remains less cluttered because you don’t need to add extra classes on every single child element.
Easier Maintenance: When you need to update the style, you change it in one place—the parent—rather than hunting down each individual element.
Improved Productivity: This approach speeds up development time, as you’re writing fewer lines of code overall.
Basic Syntax for Direct Children Styling
Tailwind CSS v3 introduced an elegant solution to style direct children using what’s called the arbitrary value syntax. Here’s the core idea:
Syntax:
[&>*]:{class}
&
represents the current element.>
is the child combinator.`` selects all direct children.
:{class}
applies the specified Tailwind utility class.
For example, if you want to add a padding of p-4
to all direct children of a div, you simply write:
<div class="[&>*]:p-4">
<div>Child 1</div>
<div>Child 2</div>
</div>
This one line of code ensures that every element directly inside the div gets the padding you want, without having to repeat p-4
on each child.
Practical Example: Styling a Navigation Menu
Let’s put theory into practice with a real-life example. Imagine you’re building a simple navigation menu and you want to apply the same styles to all the menu items. Instead of writing the classes for each <li>
element, you can apply them once to the parent <ul>
.
Before Using Child Selectors
<ul class="flex space-x-4">
<li class="text-blue-500 hover:text-blue-700">Home</li>
<li class="text-blue-500 hover:text-blue-700">About</li>
<li class="text-blue-500 hover:text-blue-700">Services</li>
<li class="text-blue-500 hover:text-blue-700">Contact</li>
</ul>
After Using Child Selectors
<ul class="flex space-x-4 [&>*]:text-blue-500 [&>*]:hover:text-blue-700">
<li>Home</li>
<li>About</li>
<li>Services</li>
<li>Contact</li>
</ul>
Notice how much cleaner the markup looks now. The repeated class names have been abstracted away and applied to all direct children using [&>*]:
.
Advanced Techniques with Arbitrary Variants
Once you’re comfortable with the basics, you might want to explore more complex scenarios. Tailwind’s arbitrary variants let you target specific child elements using more refined selectors. Here are a few examples:
Targeting Specific Elements
If you only want to style direct <p>
elements inside a container, you can use:
<div class="[&_p]:text-lg">
<p>This paragraph gets the large text.</p>
<span>This span won’t be affected.</span>
</div>
This instructs Tailwind to only apply text-lg
to <p>
tags that are direct children.
Combining Multiple Styles
You can combine multiple arbitrary variants to target different styling needs simultaneously:
<div class="[&>*]:p-4 [&>*]:mb-2 [&>h2]:text-2xl [&>h2]:font-bold">
<h2>Section Title</h2>
<p>Paragraph text goes here.</p>
<p>Another paragraph with the same padding and margin.</p>
</div>
This setup applies:
Padding (
p-4
) and margin-bottom (mb-2
) to all direct children.Specific styles (
text-2xl
andfont-bold
) only to<h2>
elements that are direct children.
Handling Hover, Focus, and Other States
Tailwind makes it super easy to combine pseudo-classes with the child selector. Let’s say you want to change the background color of child elements on hover. You can do:
<div class="[&>*]:transition-colors [&>*]:hover:bg-gray-200">
<div>Item 1</div>
<div>Item 2</div>
<div>Item 3</div>
</div>
This code makes every direct child change its background color to a light gray when hovered over, with a smooth transition effect.
Real-World Use Cases
Now that you understand the basics and some advanced techniques, let’s discuss some real-world scenarios where child selectors can be a huge win.
Building Responsive Layouts
Responsive design is key in today’s web development, and Tailwind makes it a breeze. Imagine you have a card layout where you want all direct children to have consistent margins and padding on mobile devices, but you want to adjust these styles on larger screens.
<div class="p-4 md:p-8 [&>*]:mb-4 md:[&>*]:mb-6">
<div class="bg-white shadow rounded p-4">Card 1</div>
<div class="bg-white shadow rounded p-4">Card 2</div>
<div class="bg-white shadow rounded p-4">Card 3</div>
</div>
Here, child selectors help maintain consistency in spacing, making your layout look neat across different screen sizes.
Styling Lists and Grids
If you’re working with lists or grid layouts, you might want to apply the same spacing or typography settings to every item. Child selectors ensure that each element looks exactly as intended without redundant classes.
<ul class="grid grid-cols-2 gap-4 [&>*]:bg-gray-100 [&>*]:p-4">
<li>List Item 1</li>
<li>List Item 2</li>
<li>List Item 3</li>
<li>List Item 4</li>
</ul>
This simple approach not only reduces code repetition but also keeps the styling consistent throughout the component.
Enhancing Component Libraries
For those of you building reusable components, applying a single style rule to all direct children can be a huge time-saver. Instead of forcing users to remember to add the same class to every instance of a child element, you can encapsulate the styling within the parent.
Consider a component for a card that contains an image, title, and description:
<div class="card border rounded overflow-hidden [&>*]:p-4">
<img src="image.jpg" alt="Card Image" class="w-full">
<h3 class="text-xl font-semibold">Card Title</h3>
<p class="text-gray-600">A short description of the card goes here.</p>
</div>
By using the child selector, you ensure that every direct element inside the card gets the padding, making the card’s content evenly spaced and visually appealing.
Common Pitfalls and How to Avoid Them
While child selectors in Tailwind CSS are incredibly powerful, they come with a few gotchas. Here are some tips to keep in mind:
Over-Application
It’s tempting to apply a style to every child element, but sometimes that might lead to unintended consequences. Always double-check which elements are direct children, especially when your HTML structure is complex.
Tip: Use your browser’s inspector tool to see the final computed styles. This helps ensure that only the intended elements are affected.
Specificity Conflicts
Sometimes, your direct child styles might conflict with other classes applied directly to child elements. Remember that Tailwind’s utility classes are designed to be highly specific, so if you override a child selector with an inline class, the inline class might take precedence.
Tip: Keep your styling hierarchy in mind and test changes across different components to ensure consistency.
Readability and Maintainability
Using too many arbitrary variants can make your HTML look cryptic. It’s essential to strike a balance between DRY (Don’t Repeat Yourself) principles and keeping your markup readable.
Tip: When working on large projects, consider adding comments or documentation within your code to explain why certain child selectors are used.
Best Practices for Using Child Selectors
To get the most out of Tailwind child selectors, follow these best practices:
1. Use Meaningful Container Names
Name your container classes in a way that makes their purpose clear. This not only helps with readability but also ensures that the styling is applied correctly. For instance, a class like .nav-menu
clearly indicates that the child selector rules apply to a navigation menu.
2. Keep It Modular
If you find yourself repeatedly applying the same child selector rules across multiple components, consider creating a reusable component. This reduces redundancy and makes your project easier to maintain.
3. Test Across Devices
Child selectors can sometimes behave differently depending on the structure of your HTML. Always test your components on various devices and screen sizes to ensure the styles are applied as expected.
4. Combine With Responsive Utilities
Tailwind’s responsive utilities work seamlessly with child selectors. Use media query prefixes to adjust styles for different screen sizes without having to write extra CSS.
<div class="flex flex-col md:flex-row [&>*]:p-2 md:[&>*]:p-4">
<div>Item 1</div>
<div>Item 2</div>
<div>Item 3</div>
</div>
5. Document Your Code
When using advanced selectors, adding a comment can be a lifesaver for future you (or your teammates). Explain why a certain rule is in place, which can help during code reviews or later updates.
Expanding Beyond Basics: Combining with Pseudo-Classes
Tailwind’s child selector syntax doesn’t stop at applying static styles. You can combine it with pseudo-classes like hover
, focus
, and even active
to create dynamic effects. For example:
<div class="[&>*]:transition-colors [&>*]:hover:bg-blue-100">
<div>Dynamic Item 1</div>
<div>Dynamic Item 2</div>
<div>Dynamic Item 3</div>
</div>
Here, every direct child element not only gets a transition effect but also changes its background color when hovered over. This kind of interactivity can make your user interfaces feel more responsive and engaging.
Pseudo-Classes and Child Selectors in Real-World Scenarios
Imagine you’re designing a blog post list. You might want each post summary to have a subtle hover effect, indicating that it’s clickable. With child selectors combined with pseudo-classes, you can set this up effortlessly:
<div class="space-y-4 [&>*]:p-4 [&>*]:rounded-md [&>*]:shadow [&>*]:transition-all [&>*]:hover:shadow-lg">
<div class="bg-white">Post Summary 1</div>
<div class="bg-white">Post Summary 2</div>
<div class="bg-white">Post Summary 3</div>
</div>
In this example, every post summary card gets a padding, rounded corners, a shadow, and a hover effect that increases the shadow’s intensity. It’s a clean, efficient way to create a polished UI without repetitive code.
Integrating Child Selectors with Tailwind Plugins
Tailwind’s ecosystem is rich with plugins that extend its capabilities further. Some plugins even allow you to create custom variants for targeting child elements in ways that might be more complex than what the basic syntax offers.
For instance, you might come across plugins that let you group variants together, reducing the verbosity of your classes. While native support for such grouping isn’t available yet, the community continuously comes up with creative solutions. Keep an eye on the Tailwind CSS community forums and GitHub discussions to stay updated on the latest tools and tips.
Performance and CSS Optimization
A common question is: "Does using child selectors affect performance?" The answer is generally no—Tailwind’s approach is designed to generate optimized CSS. However, it’s essential to be mindful of the overall size of your HTML and CSS. Overusing complex arbitrary variants may increase your compiled CSS size slightly, but for most projects, the convenience and maintainability gains far outweigh any minor performance costs.
Remember:
Keep your selectors focused: Use child selectors only when necessary.
Test and optimize: Tools like PurgeCSS can help remove unused CSS, ensuring your production build stays lean.
Balance simplicity and functionality: If a selector becomes too complex, consider refactoring your HTML structure.
Tips for Beginners and Intermediate Developers
Start Small and Experiment
If you’re new to Tailwind, start with the basics. Try applying simple child selectors to a small component or a section of your website. Experiment with different classes and see how they affect your elements. Over time, you’ll build the confidence to use more advanced selectors.
Leverage Documentation and Community Resources
Tailwind’s documentation is a goldmine of information. Additionally, blogs, tutorials, and community forums are excellent places to see how others are using child selectors in their projects. Sometimes, a real-world example from another developer can clear up confusion faster than reading through a long documentation page.
Don’t Overcomplicate Things
It’s tempting to try and use child selectors for every little style change, but sometimes simplicity is best. Use child selectors where they genuinely reduce repetition and improve readability. In cases where a direct class on each element is more straightforward, don’t hesitate to use that approach.
Use Browser Developer Tools
When in doubt, open your browser’s developer tools to inspect the computed styles. This can help you confirm that your child selectors are working as expected and give you insights into any unexpected behavior.
Conclusion
Tailwind CSS’s child selector syntax is a fantastic tool in your development arsenal. It allows you to write less code, maintain cleaner HTML, and create dynamic, responsive designs quickly. From the basics of applying padding to all direct children, to advanced techniques that combine pseudo-classes and arbitrary variants, there’s a lot you can do with this feature.
FAQ
What is a Tailwind CSS child selector?
It’s a feature that allows you to apply styles to direct children of an element using a simple, declarative syntax.
How does the child selector syntax work?
By adding a prefix like "[&>*]:" to your utility classes on the parent, you can style all its immediate children at once.
What benefits do child selectors offer?
They reduce code repetition, keep your HTML cleaner, and make your CSS easier to maintain.
Can child selectors be combined with pseudo-classes?
Yes, you can easily mix them with states like hover or focus for interactive styling of direct children.
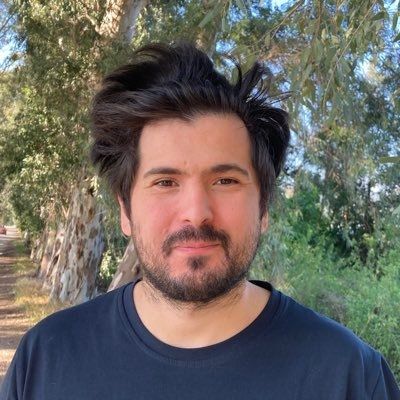
Yucel is a digital product maker and content writer specializing in full-stack development. He is passionate about crafting engaging content and creating innovative solutions that bridge technology and user needs. In his free time, he enjoys discovering new technologies and drawing inspiration from the great outdoors.