Creating Active Tabs in Tailwind CSS
Learn how to create active tabs in Tailwind CSS
Highlighting the active tab in your web application can be a challenge. Many developers face this issue. With web development always changing, making interfaces user-friendly is important. Active tabs help users move through content smoothly. In this guide, we'll show how Tailwind CSS can help you style active tabs easily and improve your site's navigation.
The Basics of Tabs in Tailwind CSS
Before we look at the code, let's see what tabs are and why they matter.
What Are Tabs?
Tabs are parts of a user interface that let visitors switch between different views or content sections without leaving the page. They organize content neatly and help users access information without clutter.
Why Use Tailwind CSS for Tabs?
Tailwind CSS is a utility-first CSS framework that offers many low-level utility classes. It lets developers build custom designs without writing lots of CSS from scratch. With Tailwind, you can quickly create personalized tab components.
Benefits of Using Tailwind CSS:
Fast Development: Utility classes make styling quick.
Customization: Tailwind offers many options to tweak designs.
Responsive Design: Built-in utilities help design for all screen sizes.
Consistency: Keeps styling uniform across your app.
Small CSS Files: Unused styles are removed, keeping CSS files small.
Setting Up Your Tailwind CSS Project
Starting a new project or adding Tailwind to an existing one? Here's how to set it up.
1. Install Tailwind CSS
Use npm to install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
2. Initialize Tailwind Configuration
Create the tailwind.config.js
and postcss.config.js
files:
npx tailwindcss init -p
3. Configure Content Paths
In your tailwind.config.js
, set the paths to your template files. This helps remove unused styles in production:
module.exports = {
content: [
'./public/**/*.html',
'./src/**/*.{js,jsx,ts,tsx,vue}',
],
theme: {
extend: {},
},
plugins: [],
}
4. Include Tailwind in Your CSS
In your main CSS file (like src/index.css
), add:
@tailwind base;
@tailwind components;
@tailwind utilities;
5. Build Your CSS
Set up your build process to compile your CSS. The Tailwind CLI is a simple option:
npx tailwindcss -i ./src/input.css -o ./dist/output.css --watch
You can also integrate it with build tools like Webpack, Gulp, or Vite.
Creating the Tab Component
With Tailwind set up, let's build our tab component.
HTML Structure
Here's a basic layout for the tabs:
<div class="tabs flex border-b" id="tabs">
<button class="tab py-2 px-4 text-blue-500 border-b-2 font-medium" data-tab="1">
Tab 1
</button>
<button class="tab py-2 px-4 text-gray-500 hover:text-blue-500" data-tab="2">
Tab 2
</button>
<button class="tab py-2 px-4 text-gray-500 hover:text-blue-500" data-tab="3">
Tab 3
</button>
</div>
<div class="tab-content p-4" id="tab-1">
<p>Content for Tab 1.</p>
</div>
<div class="tab-content p-4 hidden" id="tab-2">
<p>Content for Tab 2.</p>
</div>
<div class="tab-content p-4 hidden" id="tab-3">
<p>Content for Tab 3.</p>
</div>
Explanation:
Tabs Container (
div.tabs
): Holds all the tab buttons.Tab Buttons (
button.tab
): Each button represents a tab.Data Attributes (
data-tab
): Links tabs to their content.Tab Content (
div.tab-content
): Contains content for each tab.hidden
Class: Uses Tailwind'shidden
utility to hide inactive content.
Styling Tabs with Tailwind CSS
Now, let's style the tabs to look good and function well.
Active Tab Styling
We need the active tab to stand out from the rest.
Base Styles for Tabs:
<button class="tab py-2 px-4 text-gray-500 hover:text-blue-500 border-b-2 border-transparent font-medium" data-tab="1">
Explanation:
py-2 px-4
: Adds padding.text-gray-500
: Sets default text color.hover:text-blue-500
: Changes text color when hovered.border-b-2 border-transparent
: Adds a bottom border that's invisible by default.font-medium
: Sets font weight.
Active Tab Classes:
For the active tab, we change text and border color:
<button class="tab py-2 px-4 text-blue-500 border-b-2 border-blue-500 font-medium" data-tab="1">
Tailwind Classes Used for Active Tab:
text-blue-500
: Sets active text color.border-blue-500
: Sets active border color.
Making Tabs Interactive with JavaScript
To switch between tabs and show the right content, we'll add some JavaScript.
JavaScript Code
const tabs = document.querySelectorAll('#tabs .tab');
const tabContents = document.querySelectorAll('.tab-content');
tabs.forEach((tab) => {
tab.addEventListener('click', () => {
const target = tab.getAttribute('data-tab');
// Remove active classes from all tabs
tabs.forEach((t) => {
t.classList.remove('text-blue-500', 'border-blue-500');
t.classList.add('text-gray-500', 'border-transparent');
});
// Hide all tab contents
tabContents.forEach((c) => {
c.classList.add('hidden');
});
// Activate clicked tab
tab.classList.add('text-blue-500', 'border-blue-500');
tab.classList.remove('text-gray-500');
// Show corresponding tab content
document.getElementById('tab-' + target).classList.remove('hidden');
});
});
Explanation:
Event Listener: Adds a click event to each tab.
Data Attributes: Uses
data-tab
to identify which content to show.Class Manipulation: Toggles classes to show or hide content and style tabs.
Advanced Styling Techniques
To enhance the tabs further, consider these techniques.
Adding Icons to Tabs
Include an icon inside the button:
<button class="tab py-2 px-4 flex items-center" data-tab="1">
<svg class="w-4 h-4 mr-2"><!-- SVG icon here --></svg>
<span>Tab 1</span>
</button>
Explanation:
flex items-center
: Aligns icon and text.w-4 h-4 mr-2
: Sets icon size and margin.
Using Tailwind CSS Plugins
Install plugins like @tailwindcss/typography
for better content styling:
npm install @tailwindcss/typography
In tailwind.config.js
:
plugins: [
require('@tailwindcss/typography'),
],
Apply to tab content:
<div class="tab-content p-4 prose">
<!-- Content here -->
</div>
Hover and Focus States
Improve user feedback with hover and focus styles:
<button class="tab py-2 px-4 text-gray-500 hover:text-blue-500 focus:outline-none focus:text-blue-500" data-tab="1">
Explanation:
focus:outline-none
: Removes the default outline on focus.focus:text-blue-500
: Changes text color when focused.
Adding Transitions
Smooth out changes with transitions:
<button class="tab py-2 px-4 text-gray-500 hover:text-blue-500 transition-colors duration-300" data-tab="1">
Explanation:
transition-colors
: Applies transitions to color changes.duration-300
: Sets transition duration to 300ms.
Making Tabs Responsive
Ensure your tabs work well on all devices.
Stack Tabs Vertically on Mobile
Use responsive utilities to change layout based on screen size:
<div class="tabs flex flex-col sm:flex-row" id="tabs">
<!-- Tab buttons -->
</div>
This stacks the tabs vertically on small screens and horizontally on larger ones.
Adjusting Spacing
Change padding for different screen sizes:
<button class="tab py-2 px-4 sm:px-6 md:px-8" data-tab="1">
Handling Many Tabs
If you have many tabs, they might not fit on smaller screens. Use horizontal scrolling:
<div class="tabs flex overflow-x-auto" id="tabs">
<!-- Tab buttons -->
</div>
Explanation:
overflow-x-auto
: Allows horizontal scrolling when content overflows.
Enhancing Accessibility
Making your tabs accessible ensures everyone can use your site.
Use Semantic HTML Elements
Buttons vs. Links: Use
<button>
elements for actions.ARIA Attributes: Add
aria-selected
,role
, andtabindex
for better screen reader support.
Example:
<button
class="tab py-2 px-4"
data-tab="1"
role="tab"
aria-selected="true"
tabindex="0"
>
Tab 1
</button>
Keyboard Navigation
Allow users to navigate tabs using the keyboard:
tabs.forEach((tab, index) => {
tab.addEventListener('keydown', (e) => {
if (e.key === 'ArrowRight') {
const nextTab = tabs[index + 1] || tabs[0];
nextTab.focus();
} else if (e.key === 'ArrowLeft') {
const prevTab = tabs[index - 1] || tabs[tabs.length - 1];
prevTab.focus();
}
});
});
Explanation:
e.key === 'ArrowRight'
: Checks if the right arrow key is pressed.focus()
: Moves focus to the next or previous tab.
Descriptive Labels
Use clear labels and consider adding extra context for screen readers:
<button
class="tab py-2 px-4"
data-tab="1"
role="tab"
aria-selected="true"
tabindex="0"
aria-controls="tab-1"
>
<span class="sr-only">Section One: </span>Tab 1
</button>
Explanation:
sr-only
: Utility class that hides content visually but is accessible to screen readers.
Testing Your Tabs
Make sure your tabs work as they should across different browsers and devices.
Cross-Browser Compatibility
Test your tabs on various browsers:
Chrome
Firefox
Safari
Edge
Mobile Testing
Since many users access websites from mobile devices, ensure your tabs are:
Responsive
Easy to tap
Visually clear
Accessibility Testing
Use tools like:
Lighthouse Audit in Chrome DevTools
WAVE Accessibility Extension
These tools help find issues that might affect users with disabilities.
Troubleshooting Common Issues
Even with careful setup, problems can happen.
Styles Not Applying
Incorrect Content Paths: Ensure your content paths in
tailwind.config.js
are correct.Conflicting Classes: Check for classes that might override your styles.
JavaScript Errors
Script Loading Order: Make sure your script runs after the DOM is loaded.
Selector Mistakes: Verify your selectors match the elements in your HTML.
Responsive Issues
Breakpoints Not Working: Confirm you're using Tailwind's default breakpoints or have set them correctly in
tailwind.config.js
.Overflow Problems: Use utilities like
overflow-x-auto
to handle overflow on small screens.
Integrating with Frameworks
Using Tailwind CSS with React
When using React, manage state with hooks.
Example:
import { useState } from 'react';
function Tabs() {
const [activeTab, setActiveTab] = useState(1);
return (
<>
<div className="tabs flex border-b">
{[1, 2, 3].map((tab) => (
<button
key={tab}
className={`tab py-2 px-4 ${
activeTab === tab
? 'text-blue-500 border-b-2 border-blue-500'
: 'text-gray-500 hover:text-blue-500'
}`}
onClick={() => setActiveTab(tab)}
>
Tab {tab}
</button>
))}
</div>
<div className="tab-content p-4">
{activeTab === 1 && <p>Content for Tab 1</p>}
{activeTab === 2 && <p>Content for Tab 2</p>}
{activeTab === 3 && <p>Content for Tab 3</p>}
</div>
</>
);
}
Advantages:
State Management: Easier handling of active states.
Reusable Components: Create tab components you can use elsewhere.
Tailwind CSS with Vue.js
In Vue, use reactive data properties.
Example:
<template>
<div>
<div class="tabs flex border-b">
<button
v-for="tab in tabs"
:key="tab"
@click="activeTab = tab"
:class="activeTab === tab ? activeClass : inactiveClass"
>
Tab {{ tab }}
</button>
</div>
<div class="tab-content p-4">
<p v-if="activeTab === 1">Content for Tab 1</p>
<p v-else-if="activeTab === 2">Content for Tab 2</p>
<p v-else>Content for Tab 3</p>
</div>
</div>
</template>
<script>
export default {
data() {
return {
tabs: [1, 2, 3],
activeTab: 1,
activeClass: 'py-2 px-4 text-blue-500 border-b-2 border-blue-500',
inactiveClass: 'py-2 px-4 text-gray-500 hover:text-blue-500',
};
},
};
</script>
Best Practices for Tab Design
Keep It Simple
Don't overload tabs with too much information. Clear and concise is best.
Visual Feedback
Make sure active tabs stand out. Use colors, underlines, or bold text.
Descriptive Labels
Labels should tell users exactly what they'll find. Avoid vague terms.
Limit the Number of Tabs
Too many tabs can overwhelm users. If needed, group related content.
Accessibility First
Design with accessibility in mind from the start. It's easier than fixing it later.
Common Mistakes to Avoid
Ignoring Accessibility
Not adding ARIA attributes or keyboard navigation can make your tabs unusable for some users.
Overcomplicating Design
Complex designs can confuse users. Stick to familiar patterns unless there's a good reason to change.
Inconsistent Styling
Tabs should look and behave the same throughout your application.
Forgetting Mobile Users
Always test on mobile devices. Touch targets should be large enough, and content should be readable.
Conclusion
Creating active tabs in Tailwind CSS improves your application's navigation and user experience. With Tailwind's utility classes and a bit of JavaScript, you can build responsive, accessible, and engaging tab components. Try out these techniques to enhance your projects. Happy coding!
FAQ
How can I style an active tab in Tailwind CSS?
Apply conditional classes to the active tab, such as text-blue-500 for active text color and border-b-2 border-blue-500 for an active bottom border.
How do I manage active tab state in a Tailwind CSS component?
Use JavaScript frameworks like React or Vue to handle state management, updating the active tab based on user interaction.
What are best practices for implementing accessible tabs in Tailwind CSS?
Ensure each tab has appropriate ARIA roles and properties, such as role="tablist" for the container and role="tab" for each tab, to improve accessibility.
Can I create responsive tab components with Tailwind CSS?
Yes, utilize Tailwind's responsive utility classes to adjust tab styles across different screen sizes, ensuring a consistent user experience.
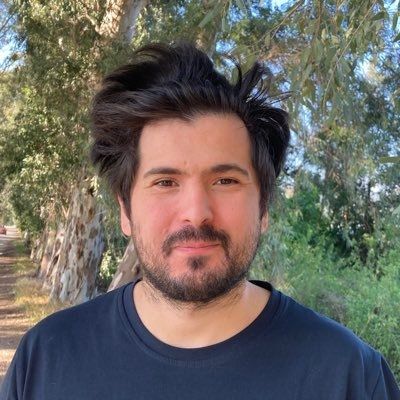
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.