Optimizing Tailwind CSS via CDNJS
Learn to optimize Tailwind CSS with CDNJS for creating lightweight and fast websites.
In today's fast-paced digital landscape, website performance is more critical than ever. Visitors expect sites to load swiftly, and search engines favor those that deliver a smooth user experience.
When combined with CDNJS—a free and public content delivery network—a quick setup is at your fingertips. However, using Tailwind CSS via CDNJS out-of-the-box may not offer the best performance. This article explores strategies to optimize Tailwind CSS through CDNJS, ensuring your website remains lightweight and fast.
The Challenge with Tailwind CSS via CDNJS
Using Tailwind CSS directly from CDNJS is convenient, but it comes with a significant drawback: file size.
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body>
<h1 class="text-3xl font-bold underline">
Hello world!
</h1>
</body>
</html>
The complete Tailwind CSS file is extensive because it includes thousands of utility classes to cover various use cases. When you load Tailwind CSS via CDNJS, you fetch the entire library, most of which your website might not need. This overhead can slow down your site's load times, particularly on devices with slower internet connections.
Optimizing Tailwind CSS for Performance
To keep your website snappy while still enjoying the benefits of Tailwind CSS via CDNJS, consider the following optimization techniques:
1. Load Only Essential Styles with PurgeCSS
Understanding PurgeCSS
PurgeCSS is a tool that removes unused CSS selectors from your stylesheets. By scanning your HTML and JavaScript files, it identifies which styles are actually used and eliminates the rest. This process can dramatically reduce the size of your CSS files.
Limitations with CDNJS
When using Tailwind CSS via CDNJS, you're importing a precompiled CSS file, leaving no room to modify it directly with PurgeCSS. However, you can still leverage PurgeCSS by:
Creating a Custom Build: Instead of relying on the CDN version, create a custom build of Tailwind CSS locally. Configure PurgeCSS to remove unused styles, and then host this optimized CSS file on your server or via a CDN.
Steps:
Install Tailwind CSS and PurgeCSS locally using npm.
Configure PurgeCSS in your
tailwind.config.js
file to include the paths to your content files.Generate the CSS by running the build process, which outputs a minimized CSS file.
Deploy the CSS: Upload the optimized CSS file to your server or a CDN for delivery.
Using Online Tools: Some online platforms allow you to generate a custom Tailwind CSS build without setting up a local environment.
Note: While this approach moves away from using CDNJS for Tailwind CSS, it significantly improves performance by serving only the styles your website needs.
2. Browser Caching with CDNJS
Browsers cache resources to reduce load times on subsequent visits. To make the most of browser caching:
Use Versioned URLs: CDNJS provides versioned URLs for its assets. By linking to a specific version of Tailwind CSS, you ensure that browsers cache the file effectively.
<link rel="stylesheet" href="<https://cdnjs.cloudflare.com/ajax/libs/tailwindcss/2.2.19/tailwind.min.css>" integrity="sha512-..." crossorigin="anonymous" referrerpolicy="no-referrer" />
Set Long Cache Expiry Headers: CDNJS sets long cache expiry headers by default, which tells browsers to cache the files for an extended period.
3. Utilize Subresource Integrity (SRI)
Subresource Integrity (SRI) allows browsers to verify that the files they fetch are delivered without unexpected manipulation. Including SRI hashes ensures security and can improve performance by enabling browsers to trust cached resources.
Example:
<link rel="stylesheet" href="<https://cdnjs.cloudflare.com/ajax/libs/tailwindcss/2.2.19/tailwind.min.css>" integrity="sha512-FpRbgUdcY..." crossorigin="anonymous" referrerpolicy="no-referrer" />
4. Prefetch and Preconnect to CDNJS
Reducing the latency in establishing connections to the CDN can improve load times:
Preconnect: Allows the browser to set up early connections before an HTTP request is sent.
<link rel="preconnect" href="<https://cdnjs.cloudflare.com>" />
Prefetch: Tells the browser to fetch resources that will be needed soon.
<link rel="dns-prefetch" href="<https://cdnjs.cloudflare.com>" />
5. Compress HTML to Reduce Initial Load
While not directly related to Tailwind CSS, compressing your HTML can reduce the overall size of the initial page load, making the inclusion of larger CSS files less impactful.
Enable GZIP or Brotli Compression: Configure your server to compress HTML, CSS, and JavaScript files.
6. Minimize Critical CSS
Identify the critical CSS needed for above-the-fold content and inline it within the <head>
of your HTML. This approach reduces render-blocking resources.
Steps:
Extract Critical CSS: Use tools like Critical or PurifyCSS to determine which styles are needed for initial rendering.
Inline Critical CSS: Place the critical CSS within
<style>
tags in the<head>
.
7. Asynchronously Load Non-Critical CSS
Deferring the loading of non-critical CSS can improve perceived performance:
Use
media
Attributes: Load CSS files with media queries that match after the initial render.<link rel="stylesheet" href="tailwind.css" media="print" onload="this.media='all'" />
Load CSS Asynchronously: Use JavaScript to load CSS files after the page has loaded.
8. Consider Using the Official Tailwind CDN with JIT Mode
Tailwind CSS offers a CDN version with Just-In-Time (JIT) mode enabled, which generates styles on the fly as they are needed.
Example:
<script src="<https://cdn.tailwindcss.com>"></script>
Advantages:
Smaller CSS Payload: Only the styles used in your HTML are generated.
No Build Step Required: Ideal for simple setups or prototypes.
Limitations:
Dependency on JavaScript: Styles are generated using JavaScript, which may not be suitable for all projects.
Not from CDNJS: This approach uses Tailwind's official CDN rather than CDNJS.
9. Optimize Delivery with HTTP/2
Ensure your server supports HTTP/2 to take advantage of multiplexing, which allows multiple resources to be sent over a single connection.
Benefits:
Reduced Latency: Improves performance by reducing the number of round trips needed.
Common Use Cases
How can I reduce the file size of Tailwind CSS when using it via CDNJS?
Answer:
When you use Tailwind CSS directly from CDNJS, you're loading the entire library, which can be substantial in size (often over 3 MB uncompressed). To reduce the file size:
Create a Custom Build with PurgeCSS:
Set Up Locally: Install Tailwind CSS and PurgeCSS using npm in a local development environment.
Configure PurgeCSS: In your
tailwind.config.js
, specify the paths to all your template files so PurgeCSS knows where to look for used classes.module.exports = { purge: ['./src/**/*.html', './src/**/*.js'], // rest of your configuration }
Build the CSS: Run the build process (
npx tailwindcss -o output.css --minify
) to generate an optimized CSS file that includes only the styles you use.Host the Optimized CSS: Upload this smaller CSS file to your server or a CDN, and link to it in your HTML.
Use the Official Tailwind CDN with JIT Mode:
Tailwind's official CDN (https://cdn.tailwindcss.com) comes with Just-In-Time (JIT) mode, which generates only the styles you use.
<script src="<https://cdn.tailwindcss.com>"></script>
Note: This method relies on JavaScript to generate styles, which may not be suitable if users have JavaScript disabled.
Is it possible to use PurgeCSS with Tailwind CSS when importing it from CDNJS?
Answer:
Unfortunately, you cannot directly use PurgeCSS to optimize Tailwind CSS when importing it from CDNJS because PurgeCSS operates on CSS files before they are sent to the client. Since the CDNJS version is a precompiled and minified CSS file, you don't have the opportunity to remove unused styles.
Workaround:
Local Build with PurgeCSS: Perform a local build of Tailwind CSS with PurgeCSS as described above, and then host the optimized CSS file yourself or on a CDN.
Benefits:
Significant Size Reduction: This process can reduce the Tailwind CSS file size from over 3 MB to just a few kilobytes, depending on how many utility classes your project uses.
Performance Improvement: Smaller CSS files lead to faster load times and better performance metrics.
What are the advantages of using the official Tailwind CDN with JIT mode over CDNJS?
Answer:
Using the official Tailwind CDN with Just-In-Time (JIT) mode offers several benefits over using the standard Tailwind CSS from CDNJS:
On-Demand CSS Generation:
Dynamic Styles: The JIT engine generates styles as they are encountered in your HTML, meaning only the required CSS is included.
Reduced File Size: This results in a much smaller CSS payload compared to the full library from CDNJS.
No Build Step Required:
Ease of Use: There's no need to set up a local development environment or build process.
Ideal for Prototyping: Quickly test ideas or build small projects without additional tooling.
Latest Features:
Up-to-Date: The official CDN often provides the latest version of Tailwind CSS, ensuring you have access to new utilities and fixes.
Considerations:
Dependency on JavaScript:
The JIT CDN relies on JavaScript to generate and inject styles, so users with JavaScript disabled won't receive the styles.
Not Suitable for All Projects:
For larger or production projects, it's recommended to perform a local build to have more control over the CSS output and to eliminate the JavaScript dependency.
How can I improve caching and load times when using Tailwind CSS from CDNJS?
Answer:
Optimizing caching and reducing load times when using Tailwind CSS from CDNJS involves several strategies:
Use Versioned URLs:
Consistency: Link to a specific version of Tailwind CSS to ensure that browsers can cache the file effectively.
<link rel="stylesheet" href="<https://cdnjs.cloudflare.com/ajax/libs/tailwindcss/2.2.19/tailwind.min.css>" integrity="sha512-..." crossorigin="anonymous" referrerpolicy="no-referrer" />
Cache Validation: Since the URL includes the version number, the browser knows when to fetch a new file if you update the version.
Leverage Subresource Integrity (SRI):
Security and Performance: Including the
integrity
attribute allows the browser to verify the file's integrity, which can improve caching efficiency.Example:
<link rel="stylesheet" href="<https://cdnjs.cloudflare.com/ajax/libs/tailwindcss/2.2.19/tailwind.min.css>" integrity="sha512-FpRbgUdcY..." crossorigin="anonymous" referrerpolicy="no-referrer" />
Prefetch and Preconnect to CDNJS:
Reduce Latency:
Preconnect: Initiates early connections to the CDN, reducing DNS lookup, TCP handshake, and TLS negotiation times.
<link rel="preconnect" href="<https://cdnjs.cloudflare.com>" />
DNS Prefetch: Resolves the CDN's DNS information ahead of time.
<link rel="dns-prefetch" href="<https://cdnjs.cloudflare.com>" />
Enable HTTP/2:
Server Configuration: Ensure your server and the CDN support HTTP/2, which allows multiplexing multiple requests over a single connection, reducing overhead.
Compress Assets:
GZIP/Brotli Compression: Although CDNJS typically serves compressed assets, verify that compression is enabled and working for your content.
Minify HTML: Reducing the overall page size helps the browser process and render content faster.
Conclusion
Optimizing Tailwind CSS via CDNJS for lightweight websites requires a multi-faceted approach. While loading the full Tailwind CSS from CDNJS is straightforward, it isn't the most performance-friendly method due to the large file size. By creating custom builds with PurgeCSS, leveraging browser caching, and utilizing modern web technologies like HTTP/2 and SRI, you can significantly enhance your website's load times.
For projects where every millisecond counts, consider alternative methods such as using Tailwind's official CDN with JIT mode or hosting your own optimized CSS files. Remember, the goal is to deliver the best possible experience to your users by keeping your website fast and responsive.
Additional Tips
Monitor Performance: Use tools like Google Lighthouse or WebPageTest to measure the impact of your optimizations.
Stay Updated: Keep an eye on updates to Tailwind CSS and CDNJS that might offer new optimization opportunities.
Test Across Devices: Ensure that your optimizations provide benefits across different browsers and devices.
By implementing these strategies, you can harness the power of Tailwind CSS via CDNJS without compromising on performance, delivering a fast and efficient experience to your website's visitors.
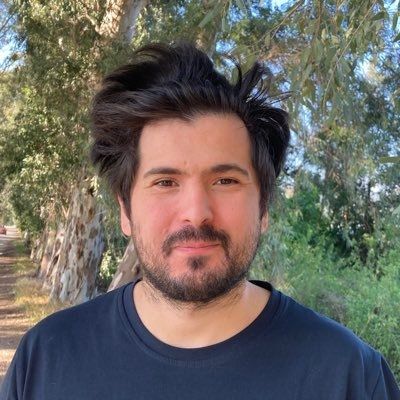
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.