Install Tailwind CSS with Vite
A detailed guide on installing Tailwind in Vite projects
Intro: Why Pair Tailwind CSS with Vite?
If you’ve been working on front-end projects lately, you’ve probably noticed more developers talking about Tailwind CSS. At the same time, Vite has been gaining a lot of traction as a build tool that focuses on speed and simplicity.
Tailwind provides a utility-first approach to styling, while Vite gives you lightning-fast bundling and a smooth development workflow. Putting them together can feel like combining two reliable teammates: one handles your style strategy with total flexibility, and the other makes sure your development process is snappy and efficient.
Before we dig into how to get Tailwind working inside a Vite project, let’s take a moment to understand what each tool brings to the table:
Tailwind CSS: Instead of writing complicated CSS files full of custom classes, Tailwind encourages you to build interfaces with small, reusable utility classes. With Tailwind, you no longer need to switch between HTML and separate style sheets; style decisions happen right in your markup, making it easier to experiment with design.
Vite: This tool has quickly grown in popularity as a replacement for older bundlers. It uses native ES modules in the browser during development, avoiding the heavy lifting other tools do before you even begin coding. The result is that your dev environment spins up instantly. There’s less waiting, and changes update faster as you code, so your feedback loop is much tighter.
Combine the two, and you get a modern front-end workflow that’s efficient, maintainable, and delightful to work with.
Prerequisites
To follow along, you’ll want to have a few things in place:
Node.js and npm Installed: Make sure you have Node.js and npm set up on your machine. You can verify this by running
node -v
andnpm -v
in your terminal.Basic Understanding of JavaScript and CSS: While you don’t need to be an expert, you should be familiar with basic web dev concepts. If you’re new to Tailwind or Vite, don’t stress. This guide breaks everything down step by step.
That’s all you really need! Once those are in place, you’re good to move on.
Setting Up a New Vite Project
If you already have a Vite project ready to go, feel free to skip ahead. But if you’re starting fresh, this section shows you how to quickly set one up.
Create a New Project Directory:
Open your terminal and run:
mkdir my-vite-project cd my-vite-project
Initialize With Vite:
Vite provides a handy command-line tool. If you have npm 6.x, you can install it with:
npm init vite@latest
If you’re using npm 7+, you might just type:
npm create vite@latest
Follow the prompts: Vite will ask you to choose a framework. If you want a vanilla JavaScript setup, pick that. If you’re working with something like React, Vue, or Svelte, choose the framework that best matches your needs.
Install Dependencies:
Once the Vite setup is done, move into your project directory (if you aren’t already) and install:
npm install
Run the Development Server:
Test that everything’s working by starting the dev server:
npm run dev
Open your browser at the given URL—usually something like
http://localhost:5173/
—and you should see the default Vite welcome screen.
Nice! We have our baseline project working. Now it’s time to bring in Tailwind.
Installing Tailwind CSS
Adding Tailwind to a project is straightforward. We’ll be using npm, but you can also use yarn or pnpm if you prefer.
From your project’s root directory, run:
npm install -D tailwindcss postcss autoprefixer
These three packages are all you need to get Tailwind up and running:
tailwindcss: The main Tailwind library.
postcss: A tool for transforming CSS; Tailwind relies on this to function properly.
autoprefixer: Automatically handles vendor prefixes, so you don’t have to worry about browser quirks as much.
Initializing Tailwind
Next, you’ll need to set up a config file for Tailwind. This file tells Tailwind what files to scan for class names and allows you to customize your design system if you want.
Run:
npx tailwindcss init -p
This command will create two files in your project’s root directory:
tailwind.config.cjs
(ortailwind.config.js
depending on your setup): Tailwind’s configuration file.postcss.config.cjs
(orpostcss.config.js
): PostCSS configuration.
Your tailwind.config.cjs
file will look something like this:
module.exports = {
content: [],
theme: {
extend: {},
},
plugins: [],
}
We need to update the content
array so Tailwind knows where to look for class names. If you set up a vanilla JS Vite project, your main HTML file and JavaScript or framework files are probably in index.html
and src/
directory. Update the content
field like so:
module.exports = {
content: [
'./index.html',
'./src/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
This tells Tailwind to scan your main HTML file and all files under src
for Tailwind classes.
Adding Tailwind’s Base Styles
To start using Tailwind, you need to include its layers: base, components, and utilities. The standard approach is to create a CSS file—often src/index.css
—and add the following:
@tailwind base;
@tailwind components;
@tailwind utilities;
If you don’t already have a CSS file to serve as an entry point, just create one. If your Vite project already has one—like src/style.css
—feel free to rename it or use that. The key is that these lines must be at the top of your main stylesheet.
Now, in your main JavaScript entry file (often main.js
or main.tsx
if you’re using a framework), import that CSS file:
import './index.css';
If you run npm run dev
again and open your browser, Tailwind is now running in the background. You won’t see anything different yet, but trust that it’s ready to go.
Verifying the Setup
Let’s do a quick check to confirm everything’s working. In index.html
, change a piece of text and add a Tailwind class to style it. For example:
<h1 class="text-4xl font-bold text-blue-600 text-center mt-10">Hello, Tailwind!</h1>
If you save and look at the page in your browser, you should see a big, bold, blue, centered heading. Congratulations—Tailwind is running inside your Vite project!
Understanding the Build Process with Vite and Tailwind
One of the best parts of using Tailwind with Vite is the speed and simplicity of the build process. In development mode, Vite takes your source files and serves them as modules, updating changes almost instantly. Tailwind uses Just-In-Time (JIT) mode by default in recent versions, which means it generates only the styles you actually use. This makes your development cycle feel incredibly light.
When you’re ready to build for production, simply run:
npm run build
Vite will bundle your code, and Tailwind will purge unused classes automatically (based on the content paths you specified). This ensures your final CSS output is as small as possible—no unnecessary bloat, just what your project needs.
Customizing the Theme
Out of the box, Tailwind gives you a well-thought-out default design system: spacing scales, color palettes, responsive breakpoints, and more. But what if you need to adjust things to fit a unique design?
That’s where the tailwind.config.cjs
file shines. The theme
field lets you override defaults or extend them. For example, if you want to add a custom color or adjust spacing:
module.exports = {
content: [
'./index.html',
'./src/**/*.{js,ts,jsx,tsx}'
],
theme: {
extend: {
colors: {
brand: '#4F46E5',
},
spacing: {
'128': '32rem',
}
},
},
plugins: [],
}
Now you have a custom brand color (brand
) and a new spacing utility class (w-128
or h-128
). Tailwind makes it easy to build your own design language on top of its robust defaults.
Using Tailwind Plugins
Tailwind’s ecosystem includes a variety of official and community-made plugins. These can add new utility classes, handle complex patterns, or provide shortcuts for things like typography or aspect ratios.
For example, the official typography plugin helps style long-form content effortlessly. To install it:
npm install -D @tailwindcss/typography
Then add it to your tailwind.config.cjs
file:
plugins: [
require('@tailwindcss/typography'),
],
After that, you can apply classes like prose
to content blocks, and Tailwind automatically handles typographic styles. This reduces a lot of guesswork and boilerplate when styling articles or blogs.
Tips and Best Practices
1. Keep Your Class Names Manageable:
Tailwind is about small utility classes, but it’s easy to go overboard. If you find a single element with 15 classes, consider whether it’s too complicated or if a simpler approach works. Sometimes, grouping a few utilities using the @apply
directive in a CSS file can make your markup cleaner. For instance:
.btn {
@apply inline-block px-4 py-2 bg-brand text-white rounded hover:bg-blue-700;
}
Then in your HTML, you just use <button class="btn">Click Me</button>
.
2. Embrace the JIT Mode:
Tailwind’s JIT mode is on by default, and it’s a game-changer. It generates classes on-demand, which means you can write arbitrary values like bg-[#123456]
or mt-[3.25rem]
and get immediate styling. This flexibility makes experimenting with design a breeze. Just be careful not to rely too heavily on arbitrary values—using predefined scales helps keep your design consistent.
3. Leverage IntelliSense and Editor Extensions:
If you use VS Code, there are extensions that suggest Tailwind classes as you type. This can speed up your workflow significantly. When combined with frameworks like React or Vue, the integration feels quite smooth.
4. Consider a Component-Driven Approach:
Even though Tailwind is utility-first, you’ll often use it in frameworks that encourage reusable components. Try defining components that are already styled and then reuse them across your app. This lets you keep your code DRY (Don’t Repeat Yourself) and consistent.
5. Regularly Review Your Design System:
Over time, you might add lots of customizations. Make a habit of revisiting your tailwind.config.cjs
to ensure everything still aligns with your project’s design goals. If you’ve added custom colors or spacing that nobody uses, it might be time to clean them up. A tidy configuration file means less confusion down the road.
Performance
One of Tailwind’s big selling points is that it removes unused classes from your CSS in production, making your final bundle smaller. When paired with Vite’s fast bundling and code splitting, you get a super performant setup by default. Still, keep a few things in mind:
Minimize Arbitrary Values: While convenient, arbitrary values mean Tailwind can’t rely as much on pre-compiled classes. This won’t drastically hurt performance, but it’s something to keep in mind.
Use the Right Content Paths: Make sure your
content
paths intailwind.config.cjs
accurately reflect where your markup lives. If Tailwind isn’t sure where to look, it might include unnecessary classes or miss some you actually need.Test Your Production Build: After running
npm run build
, inspect the output. Check the file sizes to ensure they’re reasonable. Tools like Lighthouse in Chrome’s DevTools can help identify if there’s any performance issue lurking around.
Working with Frameworks (React, Vue, Svelte)
Vite makes it incredibly easy to use frameworks like React, Vue, or Svelte. Each of these frameworks pairs nicely with Tailwind’s utility classes:
React: You’ll often write your components in
.jsx
or.tsx
files. Tailwind classes apply directly to your JSX. It feels natural to build UI elements by mixing and matching these utilities.Vue: In Vue’s single-file components (
.vue
), you can add Tailwind classes to your template section without any fuss. It just works out of the box.Svelte: Similar story—just use the classes in your
<script>
and<template>
blocks as needed.
Regardless of the framework, the idea remains the same: Tailwind classes live right in your markup. This close proximity makes it simpler to maintain and update styling as your components evolve.
Debugging Tailwind + Vite Issues
Sometimes, things don’t go as planned. Maybe your classes aren’t applying, or the build is acting weird. Here are a few steps to troubleshoot common issues:
Check Your Tailwind Config:
Make sure the
content
paths are correct. A small typo can cause Tailwind not to pick up classes.Look for Syntax Errors:
Sometimes a missing bracket in
tailwind.config.cjs
orpostcss.config.cjs
can break the build. Double-check these files for any mistakes.Clear Cache and Rebuild:
If things still don’t look right, try stopping the dev server, clearing your browser cache, and running
npm run dev
again.Check Versions:
Occasionally, version mismatches between Tailwind, PostCSS, or Autoprefixer can cause odd issues. Ensure you’re using compatible versions, and update them if necessary.
Consult the Docs or Community:
If you’re still stuck, the Tailwind and Vite docs are excellent resources. Their communities are active, and a quick search often surfaces answers to common problems.
Conclusion
Integrating Tailwind CSS with Vite offers a powerful combination of speed, flexibility, and simplicity. As you’ve seen, the installation is straightforward:
Start a Vite project.
Install Tailwind, PostCSS, and Autoprefixer.
Initialize Tailwind and configure your paths.
Add the
@tailwind
directives to your main stylesheet.Enjoy the instant feedback loop and clean, maintainable utility classes.
From here, you can take your styling game to the next level. Tweak your theme, use plugins, embrace responsive variants, and explore advanced features like dark mode. With Tailwind and Vite, you have a modern environment that encourages experimentation without getting bogged down by slow build times or messy CSS.
FAQ
Is Tailwind compatible with popular frameworks in Vite projects?
Yes. Tailwind works seamlessly with frameworks like React, Vue, and Svelte, enhancing their styling workflows.
Can I customize Tailwind’s default styling?
Absolutely. Update the Tailwind config file to add or override colors, fonts, spacing, and more.
Will Tailwind classes slow down my Vite build?
Tailwind’s JIT mode and Vite’s efficient bundling help keep builds quick, even with many utility classes.
Do I need a separate CSS file for Tailwind?
Yes, you should create a main CSS file and include Tailwind’s directives there before importing it into your project.
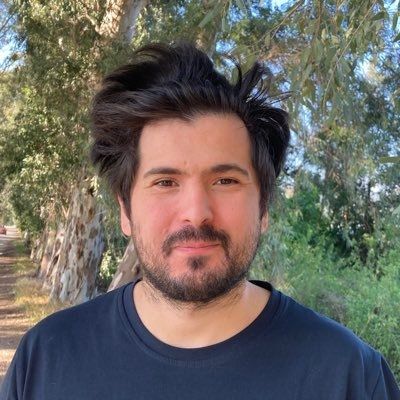
Yucel is a digital product maker and content writer specializing in full-stack development. He is passionate about crafting engaging content and creating innovative solutions that bridge technology and user needs. In his free time, he enjoys discovering new technologies and drawing inspiration from the great outdoors.