How to Integrate Tailwind with Framer Motion
Learn to integrate Tailwind CSS and Framer Motion to create stunning web animations.
Creating visually appealing and interactive web designs is essential in modern web development. Combining the utility-first styling of Tailwind CSS with the powerful animation capabilities of Framer Motion allows developers to craft stunning animations and transitions with ease.
In this guide, we'll walk through the basics of setting up both tools and demonstrate how to use them together to enhance your web projects.
1. Introduction to Tailwind CSS and Framer Motion
Tailwind CSS
Tailwind CSS is a utility-first CSS framework that provides a set of classes to build custom designs directly in your markup. It eliminates the need to write custom CSS, allowing for rapid UI development.
Framer Motion
Framer Motion is a production-ready motion library for React that makes it simple to create animations and gestures. It provides a declarative API that is both powerful and easy to use.
2. Setting Up Tailwind CSS
To get started with Tailwind CSS in a React project:
Install Tailwind CSS and its dependencies:
npm install tailwindcss postcss autoprefixer
Initialize Tailwind CSS:
npx tailwindcss init -p
Configure Tailwind to remove unused styles in production:
Update the
tailwind.config.js
file:module.exports = { purge: ['./src/**/*.{js,jsx,ts,tsx}', './public/index.html'], darkMode: false, // or 'media' or 'class' theme: { extend: {}, }, variants: { extend: {}, }, plugins: [], }
Include Tailwind in your CSS:
In your main CSS file (e.g.,
index.css
), add:@tailwind base; @tailwind components; @tailwind utilities;
3. Installing Framer Motion
Install Framer Motion using npm:
npm install framer-motion
4. Integrating Tailwind CSS with Framer Motion
With both Tailwind CSS and Framer Motion set up, you can start combining them in your components.
Use Tailwind CSS classes for styling your components.
Wrap components with Framer Motion's
motion
components to add animations.
5. Creating Animations and Transitions
Here's how to create basic animations:
Example: Fading in a Component
import React from 'react';
import { motion } from 'framer-motion';
const FadeInComponent = () => {
return (
<motion.div
className="p-6 bg-blue-500 text-white rounded-lg"
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
transition={{ duration: 1 }}
>
Welcome to the Animated World!
</motion.div>
);
};
export default FadeInComponent;
Styling: The
className
prop uses Tailwind CSS classes for styling.Animation: The
initial
,animate
, andtransition
props from Framer Motion control the animation.
Example: Hover Effects
const HoverEffectComponent = () => {
return (
<motion.button
className="px-4 py-2 bg-green-500 text-white rounded"
whileHover={{ scale: 1.1 }}
whileTap={{ scale: 0.9 }}
>
Hover Me
</motion.button>
);
};
export default HoverEffectComponent;
Animation on Hover:
whileHover
andwhileTap
props provide interactivity.
6. Example Projects
Animated Navigation Menu
Create an interactive navigation menu that slides in:
const navVariants = {
hidden: { x: '-100%' },
visible: { x: 0 },
};
const NavigationMenu = () => {
return (
<motion.nav
className="fixed top-0 left-0 w-64 h-full bg-gray-800 text-white"
variants={navVariants}
initial="hidden"
animate="visible"
transition={{ type: 'spring', stiffness: 70 }}
>
{/* Menu items */}
</motion.nav>
);
};
Staggered List Animation
Animate list items with a staggered effect:
const list = {
visible: {
opacity: 1,
transition: {
when: "beforeChildren",
staggerChildren: 0.3,
},
},
hidden: { opacity: 0 },
};
const item = {
visible: { opacity: 1, y: 0 },
hidden: { opacity: 0, y: 20 },
};
const StaggeredList = () => (
<motion.ul
className="space-y-4"
variants={list}
initial="hidden"
animate="visible"
>
{[1, 2, 3].map((i) => (
<motion.li
key={i}
className="p-4 bg-indigo-500 text-white rounded"
variants={item}
>
Item {i}
</motion.li>
))}
</motion.ul>
);
Conclusion
Integrating Tailwind CSS with Framer Motion unlocks a powerful combination for building beautifully animated and styled web components.
By combining the strengths of Tailwind CSS and Framer Motion, you can elevate your web projects with exceptional design and interactivity.
FAQ
Is it possible to animate Tailwind CSS utility classes with Framer Motion?
Framer Motion animates inline styles rather than class names. However, you can use Tailwind CSS to define the base styles and use Framer Motion to animate specific properties. For properties not separately animatable, consider using the animate prop to toggle class names, though this approach requires additional handling.
How do I create custom animations with Framer Motion?
Framer Motion allows for extensive customization through its animation props like variants, initial, animate, and transition. You can define complex animations and state transitions using these props.
Do Tailwind CSS and Framer Motion impact performance?
Tailwind CSS is optimized for minimal CSS bloat by purging unused styles in production builds. Framer Motion is designed to be performant with hardware acceleration. However, excessive animations can impact performance, so use them judiciously.
Can I use Tailwind CSS and Framer Motion with Next.js projects?
Yes, both Tailwind CSS and Framer Motion can be seamlessly integrated into Next.js projects. Follow the Next.js specific setup guides for Tailwind CSS, and use Framer Motion as you would in any React application.
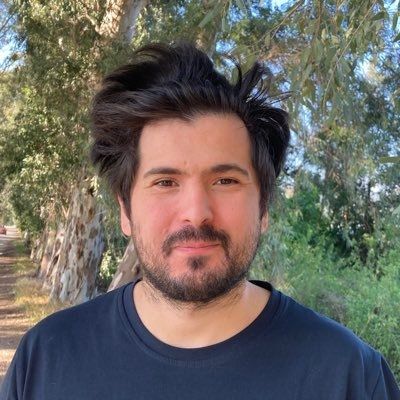
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.