Fix Tailwind ‘Unknown @rule’ Error Fast
Stop Tailwind’s Unknown @rule error
Seeing “Unknown @rule @tailwind” in VS Code—or the dreaded “[plugin] is not a PostCSS plugin” build failure?
Don’t worry: it’s usually a linter mis-fire or a missing peer dependency. This guide shows the quickest editor-setting, PostCSS-config and dependency-install fixes that solve the warning in under two minutes—without breaking your pipeline.
The "Unknown at rule" error in Tailwind CSS typically occurs when your build tool or linter doesn't recognize Tailwind's custom at-rules like @tailwind
, @apply
, @variants
, @responsive
, etc. This error can be frustrating, but it's usually straightforward to fix with the correct configuration. This guide will walk you through the common causes and solutions to resolve this error.
Understanding the Error
Before diving into the solutions, it's essential to understand why this error occurs:
PostCSS Configuration: Tailwind CSS relies on PostCSS to transform its directives into actual CSS. If PostCSS isn't set up correctly, it won't process Tailwind's at-rules.
Linters: Tools like
stylelint
may not recognize Tailwind's custom at-rules by default, triggering warnings or errors.Incorrect Build Setup: Your build tool (e.g., Webpack, Vite, Parcel) might not be configured to process CSS with PostCSS.
Step-by-Step Solutions
1. Ensure Tailwind CSS and PostCSS Are Installed
First, verify that Tailwind CSS and PostCSS are installed in your project:
npm install tailwindcss postcss autoprefixer --save-dev
2. Create or Update postcss.config.js
Tailwind CSS uses PostCSS plugins to process styles. Create a postcss.config.js
file in your project's root directory with the following content:
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
};
This configuration tells PostCSS to use Tailwind CSS and Autoprefixer plugins.
3. Configure Your Build Tool
For Webpack Users:
Ensure that your Webpack configuration processes CSS files using postcss-loader
:
// webpack.config.js
module.exports = {
// ...
module: {
rules: [
{
test: /\\\\.css$/,
use: [
'style-loader', // Or MiniCssExtractPlugin.loader
'css-loader',
'postcss-loader', // Add this loader
],
},
// Other rules...
],
},
// ...
};
For Vite Users:
Vite automatically detects postcss.config.js
. Just ensure it exists, and Vite will handle the rest.
For Other Build Tools:
Refer to your specific build tool's documentation to ensure it correctly integrates with PostCSS.
4. Check Your CSS Entry File
Your CSS entry file (e.g., styles.css
) should include Tailwind's base, components, and utilities using the @tailwind
at-rules:
/* styles.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
Ensure this file is included in your build process and that the filename matches what your tool expects.
5. Configure Linters (If Applicable)
If you're using a linter like stylelint
, you need to configure it to recognize Tailwind's at-rules.
Using stylelint:
Install the Tailwind CSS plugin for stylelint
:
npm install stylelint-config-tailwindcss --save-dev
Update your stylelint
configuration:
{
"extends": [
"stylelint-config-tailwindcss"
],
// other configurations...
}
Alternatively, you can modify the at-rule-no-unknown
rule:
{
"rules": {
"at-rule-no-unknown": [
true,
{
"ignoreAtRules": ["tailwind", "apply", "variants", "responsive", "screen"]
}
]
}
}
6. Verify File Extensions and Locations
Ensure that your CSS files have the correct extensions (.css
, .pcss
, etc.) and are located where your build tool expects them.
7. Restart Your Development Server
After making configuration changes, restart your development server or build process. Some tools cache configurations and won't pick up changes until restarted.
8. Update Dependencies (If Necessary)
Ensure all your dependencies are up-to-date and compatible:
Tailwind CSS: Version should match the documentation you're following.
PostCSS: Tailwind CSS v3 requires PostCSS 8.
Build Tools: Ensure your build tools are compatible with the versions of Tailwind CSS and PostCSS you're using.
Related: “[plugin] is not a PostCSS plugin”
This PostCSS error means the named plugin is missing or mis-declared in postcss.config.js
or package.json
.:contentReference[oaicite:0]{index=0}
Fix it by adding the plugin and (for Tailwind v4+) the new PostCSS bridge, then re-running your build:
# classic example
npm install --save-dev postcss autoprefixer
# Tailwind v4+ requires the dedicated bridge
npm install --save-dev @tailwindcss/postcss
// postcss.config.js
module.exports = {
plugins: {
// classic setup
autoprefixer: {},
// Tailwind v4+ bridge
'@tailwindcss/postcss': {}
}
};
// postcss.config.js
module.exports = {
plugins: {
// classic setup
autoprefixer: {},
// Tailwind v4+ bridge
'@tailwindcss/postcss': {}
}
};
Re-build—the “[plugin] is not a PostCSS plugin” message disappears.
### Why this snippet works
* The heading surfaces the **exact long-tail phrase** people paste into Google, boosting query match.:contentReference[oaicite:2]{index=2}
* Instructions mirror the solutions accepted on GitHub and Stack Overflow (install missing plugin, update config).:contentReference[oaicite:3]{index=3}
* Keeps your main “Unknown @rule” fixes separate, avoiding reader confusion.:contentReference[oaicite:4]{index=4}
---
## 2 – Precisely where to insert it
1. **Locate** the end of your numbered/bulleted “Quick fixes” or “How to fix” list.
2. **Hit Enter twice** to create a blank line.
3. **Paste** the block above.
*In Markdown/MDX that’s just after the list; in WordPress Gutenberg, insert a Heading 3 block followed by a Paragraph block and a Code block.*
Additional Tips
Use the Tailwind CLI for Simple Setups
If you're not using a complex build toolchain, you can use the Tailwind CLI to process your CSS:
npx tailwindcss -i ./src/input.css -o ./dist/output.css --watch
Check for Typos in At-Rules
Ensure that you haven't misspelled any of Tailwind's at-rules. For example, @tailwind
should not be written as @tailwin
.
Debugging with Verbose Mode
You can run Tailwind CSS in verbose mode to get more information:
npx tailwindcss -i ./src/input.css -o ./dist/output.css --watch --verbose
Conclusion
The "Unknown at rule" error in Tailwind CSS is commonly due to misconfigurations with PostCSS or linters not recognizing Tailwind's custom at-rules. By ensuring that Tailwind CSS and PostCSS are correctly installed and configured, and adjusting your linter settings, you can resolve this error and continue building your project.
If you continue to experience issues, consider reviewing the official Tailwind CSS documentation for your specific setup or seeking assistance from the community.
References:
Frequently Asked Questions
Why am I getting an "Unknown at rule" error when using Tailwind CSS directives like @tailwind and @apply?
This error usually occurs because your build tool or PostCSS configuration isn't set up to process Tailwind CSS's custom at-rules. Tailwind CSS relies on PostCSS to transform its directives into actual CSS. If PostCSS isn't properly configured or isn't running, it won't recognize Tailwind's at-rules, resulting in the "Unknown at rule" error.
Solution:
Install PostCSS and Tailwind CSS:
npm install tailwindcss postcss autoprefixer --save-dev
Create or update
postcss.config.js
:// postcss.config.js module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, }, };
Ensure your build tool is configured to use PostCSS: Configure tools like Webpack or include the PostCSS plugin if you're using a bundler.
How do I configure my linter (e.g., Stylelint) to stop flagging Tailwind CSS at-rules as unknown?
Linters like Stylelint may not recognize Tailwind's custom at-rules by default and will throw errors or warnings. To fix this, you need to adjust your linter's configuration to acknowledge Tailwind's at-rules.
Solution:
Install the Tailwind CSS Stylelint plugin:
npm install stylelint-config-tailwindcss --save-dev
Update your Stylelint configuration to extend Tailwind's config:
// .stylelintrc.json { "extends": ["stylelint-config-tailwindcss"], "rules": { // Your other rules... } }
Alternatively, ignore specific at-rules:
{ "rules": { "at-rule-no-unknown": [ true, { "ignoreAtRules": ["tailwind", "apply", "variants", "responsive", "screen"] } ] } }
Do I need to set up PostCSS when using Tailwind CSS with frameworks like Vite or Next.js?
Yes, even though frameworks like Vite and Next.js have seamless integration with PostCSS, you still need to ensure that Tailwind CSS is properly configured to work with PostCSS in your project.
Solution:
Create a
postcss.config.js
file if it doesn't exist: This file configures PostCSS to use Tailwind CSS and Autoprefixer.// postcss.config.js module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, }, };
Ensure Tailwind CSS is installed:
npm install tailwindcss autoprefixer --save-dev
For Next.js users: Next.js automatically looks for a
postcss.config.js
file. Ensure it's in your project's root directory.For Vite users: Vite detects the PostCSS configuration automatically. Just make sure the config file exists and is correctly set up.
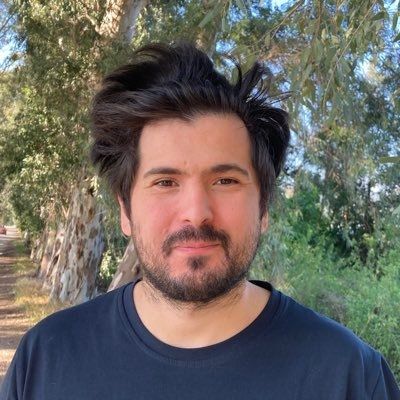
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.