How to Build Modals in React JS: A Step-by-Step Guide
Learn to build modals in React JS with this easy step-by-step guide.
Modals are an essential component of modern web applications, providing a way to display content in a layer above the main app without navigating away from the current screen. In this guide, we'll explore how to build modals in React JS using the react-modal library and style them with Tailwind CSS colors. We'll also cover how to manage modal state and customize the modal's appearance
Prerequisites
Before we begin, make sure you have the following:
Node.js and npm installed on your machine.
Basic understanding of React JS and component states.
Familiarity with CSS and Tailwind CSS for styling.
Setting Up the React Project
Start by creating a new React application using Create React App:
npx create-react-app react-modal-tutorial
cd react-modal-tutorial
Installing Dependencies
We'll need react-modal for the modal functionality and Tailwind CSS for styling.
Install react-modal
npm install react-modal
Set Up Tailwind CSS
Install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Configure tailwind.config.js
to remove unused styles in production:
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Add Tailwind directives to src/index.css
:
@tailwind base;
@tailwind components;
@tailwind utilities;
Creating the Modal Component
Create a new file src/Modal.js
for the modal component.
import React from 'react';
import Modal from 'react-modal';
Modal.setAppElement('#root'); // Accessibility feature
function CustomModal({ isOpen, onRequestClose }) {
return (
<Modal
isOpen={isOpen}
onRequestClose={onRequestClose}
contentLabel="Example Modal"
className="bg-white rounded-lg p-6 max-w-lg mx-auto mt-20"
overlayClassName="fixed inset-0 bg-black bg-opacity-50 flex justify-center items-center"
>
<h2 className="text-2xl font-bold mb-4 text-gray-800">Modal Title</h2>
<p className="mb-4 text-gray-600">
This is a customizable modal window built with React JS and styled using Tailwind CSS.
</p>
<button
onClick={onRequestClose}
className="bg-blue-500 hover:bg-blue-600 text-white font-semibold py-2 px-4 rounded"
>
Close Modal
</button>
</Modal>
);
}
export default CustomModal;
In this component:
Modal.setAppElement('#root') is called for accessibility purposes.
The modal content is styled using Tailwind CSS classes.
The onRequestClose handler closes the modal when the overlay is clicked or the ESC key is pressed.
Styling the Modal with Tailwind CSS
We're using Tailwind CSS colors and utility classes to style the modal:
bg-white
,text-gray-800
for the modal background and text color.bg-blue-500
,hover:bg-blue-600
for the button with hover effect.Spacing is managed with
p-6
,mb-4
,mt-20
, etc.The overlay is styled with
bg-black bg-opacity-50
to create a semi-transparent background.
Implementing Modal Functionality
Now, integrate the CustomModal
component into your main App.js
file.
import React, { useState } from 'react';
import CustomModal from './Modal';
function App() {
const [modalIsOpen, setModalIsOpen] = useState(false);
const openModal = () => {
setModalIsOpen(true);
};
const closeModal = () => {
setModalIsOpen(false);
};
return (
<div className="App">
<button
onClick={openModal}
className="mt-20 bg-green-500 hover:bg-green-600 text-white font-semibold py-2 px-6 rounded"
>
Open Modal
</button>
<CustomModal isOpen={modalIsOpen} onRequestClose={closeModal} />
</div>
);
}
export default App;
Explanation:
State Management: We use the
useState
hook to control the modal's open state.Event Handlers:
openModal
andcloseModal
functions handle opening and closing the modal.Button Styling: The "Open Modal" button is styled with Tailwind CSS classes.
Conclusion
By following this guide, you've learned how to create a customizable modal window in React JS using react-modal and style it with Tailwind CSS colors and utility classes. Modals enhance user experience by displaying important content without navigating away from the current page. You can now implement modals in your projects to display alerts, forms, images, and more.
Use Cases
Can I create a modal without using external libraries like react-modal
?
Yes, you can create modals using pure React by managing component state and conditionally rendering modal content. However, using react-modal
simplifies accessibility concerns and provides built-in functionality for handling overlays, focus management, and more.
How do I customize the overlay background color or opacity?
You can customize the overlay by modifying the overlayClassName
prop in the Modal
component. For example, to change the overlay to a semi-transparent red:
overlayClassName="fixed inset-0 bg-red-500 bg-opacity-50 flex justify-center items-center"
This uses Tailwind CSS colors to adjust the overlay appearance.
By integrating modals into your React applications and styling them with Tailwind CSS, you enhance user interaction and interface aesthetics. Whether you're displaying alerts, forms, or additional information, modals are a powerful tool in your React JS toolkit.
FAQ
Does react-modal support server-side rendering (SSR)?
Yes, react-modal works with SSR frameworks like Next.js. Ensure that you handle the Modal.setAppElement appropriately, possibly within a useEffect hook to avoid SSR issues.
How can I make the modal accessible for screen readers?
The react-modal library is designed with accessibility in mind. By calling Modal.setAppElement('#root'), you inform screen readers about the main content area. Additionally, react-modal handles focus trapping within the modal when it's open, improving accessibility.
Is it possible to animate the modal appearance and disappearance?
Yes, you can add animations using CSS transitions or by integrating animation libraries like Framer Motion or React Spring. Apply animations to the modal's classNames or wrap the modal content with animated components.
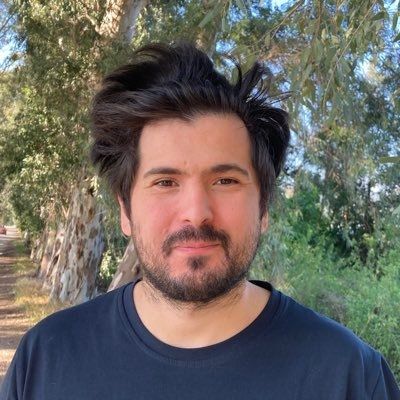
Yucel is a digital product maker and content writer specializing in full-stack development. He is passionate about crafting engaging content and creating innovative solutions that bridge technology and user needs. In his free time, he enjoys discovering new technologies and drawing inspiration from the great outdoors.