How to Build a Responsive Dialog with Tailwind CSS
Build responsive dialogs using Tailwind CSS
When designing applications, it’s often necessary to draw the user’s attention to a critical piece of information or prompt them to make a decision. Dialog is —commonly known as a modal—an overlay element that focuses the user’s attention on a single task.
With Tailwind CSS, creating a clean, responsive, and accessible dialog is straightforward. In this guide, we’ll walk through how to implement and customize a dialog using Tailwind’s utility-first classes and reference patterns demonstrated in Tailwind UI’s Modal Dialogs.
Why Use Tailwind for Dialogs?
Tailwind CSS gives you low-level utility classes that allow you to style elements without leaving the HTML. For dialogs, this means you can quickly change sizing, spacing, colors, and positioning without wrestling with complex CSS files. Coupled with Tailwind UI—which provides professionally designed, fully responsive UI components—you can have a polished dialog up and running in minutes.
Benefits include:
Rapid Customization: Tweak padding, margins, background colors, and animation directly in the markup.
Responsive by Default: Tailwind’s responsive classes make ensuring a great mobile and desktop experience a breeze.
Accessible Foundations: Tailwind UI’s examples include proper ARIA attributes and focus management patterns, helping ensure your dialogs are accessible to all users.
Getting Started
Prerequisites
A project with Tailwind CSS already set up. (If not, follow the Tailwind CSS installation guide.)
Basic knowledge of HTML and JavaScript (for toggling the dialog’s visibility).
Basic Structure of a Dialog
A dialog typically consists of three main parts:
Overlay: A semi-transparent backdrop that covers the page, drawing attention to the dialog.
Dialog Container: A centered box containing your content—usually a title, descriptive text, and action buttons.
Close Mechanisms: A button or icon to close the dialog, and possibly support closing on clicking the backdrop or pressing the
ESC
key.
Here’s a simplified HTML structure before we apply Tailwind classes:
<!-- Trigger Button -->
<button id="openDialogBtn">Open Dialog</button>
<!-- Dialog Wrapper -->
<div id="dialogWrapper" class="hidden fixed inset-0 z-50 flex items-center justify-center">
<!-- Overlay -->
<div class="fixed inset-0 bg-gray-500 bg-opacity-75 transition-opacity"></div>
<!-- Dialog Box -->
<div class="bg-white rounded-lg shadow-xl overflow-hidden transform transition-all max-w-lg w-full">
<div class="px-4 py-5 sm:px-6">
<h3 class="text-lg leading-6 font-medium text-gray-900">Dialog Title</h3>
<p class="mt-2 text-sm text-gray-500">This is the dialog content area. Use it to share information, warnings, or confirmation steps with the user.</p>
</div>
<div class="px-4 py-3 sm:px-6 sm:flex sm:flex-row-reverse">
<button id="confirmBtn" class="ml-3 inline-flex justify-center rounded-md border border-transparent bg-indigo-600 px-4 py-2 text-base font-medium text-white hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500">
Confirm
</button>
<button id="closeDialogBtn" class="mt-3 inline-flex justify-center rounded-md border border-gray-300 bg-white px-4 py-2 text-base font-medium text-gray-700 hover:bg-gray-50 focus:outline-none focus:ring-2 focus:ring-indigo-500 sm:mt-0 sm:ml-3">
Cancel
</button>
</div>
</div>
</div>
Applying Tailwind Classes for Styling and Responsiveness
Above, we’ve already introduced some Tailwind classes:
Positioning & Layout:
fixed inset-0
places the dialog wrapper and overlay to cover the entire screen.z-50
ensures the dialog appears above other page elements.flex items-center justify-center
centers the dialog box on the screen.
Overlay:
bg-gray-500 bg-opacity-75
creates a semi-transparent dark backdrop.
Dialog Box:
bg-white rounded-lg shadow-xl
gives the dialog a clean, modern look.max-w-lg w-full
ensures it’s responsive and never grows too wide for smaller screens.
Typography & Spacing:
px-4 py-5
andsm:px-6
for responsive padding.text-lg leading-6 font-medium text-gray-900
for the title.text-sm text-gray-500
for the supporting text.
Buttons:
Primary button:
bg-indigo-600 text-white hover:bg-indigo-700
for a clear call-to-action.Secondary button:
bg-white border border-gray-300 text-gray-700 hover:bg-gray-50
blends well with the dialog background.
Feel free to change colors, sizing, and spacing with Tailwind classes until it matches your brand’s style.
Adding Interactivity with JavaScript
You’ll need a bit of JavaScript to toggle the dialog’s visibility. We can do this by toggling the hidden
class on the #dialogWrapper
container.
<script>
const openDialogBtn = document.getElementById('openDialogBtn');
const dialogWrapper = document.getElementById('dialogWrapper');
const closeDialogBtn = document.getElementById('closeDialogBtn');
// Open Dialog
openDialogBtn.addEventListener('click', () => {
dialogWrapper.classList.remove('hidden');
});
// Close Dialog
closeDialogBtn.addEventListener('click', () => {
dialogWrapper.classList.add('hidden');
});
// Close on overlay click
dialogWrapper.addEventListener('click', (event) => {
if (event.target === dialogWrapper) {
dialogWrapper.classList.add('hidden');
}
});
// Close on ESC key
document.addEventListener('keydown', (event) => {
if (event.key === 'Escape') {
dialogWrapper.classList.add('hidden');
}
});
</script>
With just a few lines of JavaScript, we’ve added the essential interactivity that a modal dialog needs:
Clicking the “Open Dialog” button opens the dialog.
Clicking “Cancel” or pressing the
ESC
key closes the dialog.Clicking outside the dialog box also closes it.
Additional Accessibility Considerations
Dialogs should meet accessibility standards:
Focus Management: Ensure that when the dialog opens, focus moves inside it (e.g., onto the first focusable element, like the primary action button). When it closes, return focus to the element that triggered it.
ARIA Attributes: Add
role="dialog"
andaria-modal="true"
to the dialog box. Label your dialog witharia-labelledby
linked to the title to help screen readers interpret the structure and purpose.
A more accessible dialog box might look like this:
<div
id="dialogWrapper"
class="hidden fixed inset-0 z-50 flex items-center justify-center"
role="dialog"
aria-modal="true"
aria-labelledby="dialogTitle"
>
<div class="fixed inset-0 bg-gray-500 bg-opacity-75"></div>
<div class="bg-white rounded-lg shadow-xl overflow-hidden transform transition-all max-w-lg w-full">
<div class="px-4 py-5 sm:px-6">
<h3 id="dialogTitle" class="text-lg leading-6 font-medium text-gray-900">Dialog Title</h3>
<p class="mt-2 text-sm text-gray-500">This is the dialog content area...</p>
</div>
<div class="px-4 py-3 sm:px-6 sm:flex sm:flex-row-reverse">
<button id="confirmBtn" class="ml-3 inline-flex justify-center rounded-md border border-transparent bg-indigo-600 px-4 py-2 text-base font-medium text-white hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500">
Confirm
</button>
<button id="closeDialogBtn" class="mt-3 inline-flex justify-center rounded-md border border-gray-300 bg-white px-4 py-2 text-base font-medium text-gray-700 hover:bg-gray-50 focus:outline-none focus:ring-2 focus:ring-indigo-500 sm:mt-0 sm:ml-3">
Cancel
</button>
</div>
</div>
</div>
By including these attributes and managing focus in JavaScript, you’ll ensure that your dialog is not only beautiful but also usable by everyone.
Customization Ideas
Animations: Tailwind’s
transition
classes allow you to add a fade-in/fade-out effect or scale transitions for a more polished experience.Different Colors/Themes: Change
bg-white
tobg-gray-100
or tweak button colors to match your brand.Multiple Dialog Sizes: Use responsive classes like
sm:max-w-md
ormd:max-w-xl
to provide different dialog sizes based on device width.
Conclusion
With Tailwind CSS and references from Tailwind UI Modal Dialogs, you can build clean, accessible, and stylish dialogs in no time. By leveraging utility classes, you’ll have full control over the visual design. Adding a sprinkle of JavaScript ensures a smooth user experience—opening, closing, and properly handling focus and accessibility concerns.
Tailwind dialogs are a powerful addition to your UI toolkit. Give them a try in your next project and see how quickly you can implement a polished, professional user experience.
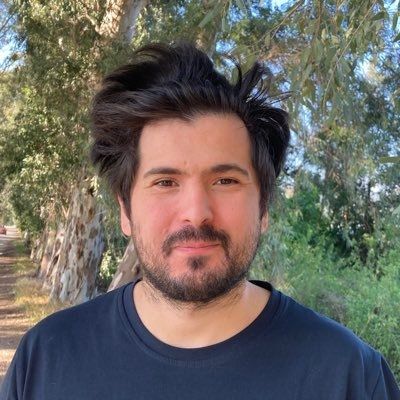
Yucel is a digital product maker and content writer specializing in full-stack development. He is passionate about crafting engaging content and creating innovative solutions that bridge technology and user needs. In his free time, he enjoys discovering new technologies and drawing inspiration from the great outdoors.