Extend Tailwind Padding Scale for Precise Layouts
Learn to customize Tailwind CSS's padding scale for advanced spacing control.
Padding plays a crucial role in web design, influencing the spacing and overall aesthetics of a layout. While Tailwind CSS offers a robust set of padding utilities out of the box, there are scenarios where you might need to push beyond the default scale to achieve a unique design or align with a specific design system.
In this article, we'll explore advanced padding strategies in Tailwind CSS, including how to extend and customize the padding scale, create utility plugins for specific needs, and integrate custom padding configurations into your projects.
Extending the Padding Scale
Tailwind CSS allows you to customize its default padding scale to fit your project's requirements. By extending the padding scale, you can add new values that align with your design system or unique layout needs.
Adding New Padding Values
To add new padding values, you'll need to extend the padding
section in your tailwind.config.js
file:
// tailwind.config.js
module.exports = {
theme: {
extend: {
padding: {
'18': '4.5rem', // Add a custom value for p-18
'double': '200%', // Custom value using percentage
},
},
},
variants: {},
plugins: [],
}
With this configuration, you can now use p-18
and p-double
in your HTML:
<div class="p-18 bg-gray-200">
This div has 4.5rem padding.
</div>
<div class="p-double bg-gray-300">
This div has 200% padding.
</div>
Using Arbitrary Values
Tailwind CSS also supports arbitrary values, allowing you to apply custom padding on the fly without extending the scale:
<div class="p-[3.75rem] bg-gray-400">
This div has 3.75rem padding using an arbitrary value.
</div>
While arbitrary values offer flexibility, it's recommended to extend the padding scale for values you'll reuse to maintain consistency.
Creating Custom Padding Utilities with Plugins
For more specific padding needs, you can create custom utilities using Tailwind CSS plugins. This approach is useful when you need complex padding behaviors that aren't supported by the default utilities.
Creating a Custom Plugin
Let's create a plugin that adds padding utilities for aspect ratios:
// plugins/aspect-ratio-padding.js
module.exports = function({ addUtilities }) {
const newUtilities = {
'.p-aspect-1-1': {
paddingTop: '100%',
},
'.p-aspect-16-9': {
paddingTop: '56.25%',
},
'.p-aspect-4-3': {
paddingTop: '75%',
},
};
addUtilities(newUtilities, ['responsive']);
};
Then include the plugin in your tailwind.config.js
:
// tailwind.config.js
const aspectRatioPadding = require('./plugins/aspect-ratio-padding');
module.exports = {
// ...
plugins: [
aspectRatioPadding,
],
}
Now you can use these custom padding utilities:
<div class="relative w-full p-aspect-16-9 bg-gray-500">
<!-- Content that maintains a 16:9 aspect ratio -->
</div>
Benefits of Custom Plugins
Reusability: Encapsulate complex padding patterns for reuse.
Readability: Improve code clarity by using meaningful class names.
Maintainability: Centralize padding logic, making it easier to update.
Integrating Custom Padding with Design Systems
When working with a design system, ensuring that your padding scales align with the defined spacing units is critical for consistency.
Aligning with Design Tokens
Suppose your design system defines spacing tokens like so:
spacing-xs
: 4pxspacing-sm
: 8pxspacing-md
: 16pxspacing-lg
: 24pxspacing-xl
: 32px
You can integrate these into Tailwind CSS:
// tailwind.config.js
module.exports = {
theme: {
extend: {
padding: {
'xs': '4px',
'sm': '8px',
'md': '16px',
'lg': '24px',
'xl': '32px',
},
},
},
}
Now, use these tokens in your classes:
<div class="p-md bg-gray-100">
This div uses the medium padding defined in the design system.
</div>
Synchronizing with Design Updates
By centralizing these values in your Tailwind configuration, any updates to your design system's spacing can be easily propagated throughout your project by updating the configuration.
Unique Padding Configurations
Advanced layouts often require unique padding configurations to achieve a particular look and feel.
Responsive Padding Adjustments
You can adjust padding at different breakpoints for responsive designs:
<div class="p-4 md:p-8 lg:p-16 bg-gray-200">
This div has different padding at small, medium, and large screen sizes.
</div>
Directional Padding Utilities
Tailwind provides utilities for specific sides:
pt-*
for padding-toppr-*
for padding-rightpb-*
for padding-bottompl-*
for padding-leftpx-*
for padding-left and padding-rightpy-*
for padding-top and padding-bottom
Example:
<div class="pt-6 pr-4 pb-2 pl-8 bg-gray-300">
Custom directional padding.
</div>
Combined Arbitrary Values and Directional Utilities
Use arbitrary values with directional utilities for precise control:
<div class="pt-[10%] pb-[5rem] bg-gray-400">
Top padding of 10% and bottom padding of 5rem.
</div>
Maintaining Design Consistency
While it's tempting to use custom padding values liberally, it's essential to maintain consistency to ensure a cohesive design.
Best Practices
Stick to a Scale: Define a spacing scale and use it consistently.
Limit Arbitrary Values: Use them sparingly for exceptions rather than the rule.
Document Custom Utilities: Maintain a reference for any custom utilities added.
Review Responsiveness: Ensure custom padding works well across all screen sizes.
Collaborating with Designers
Work closely with your design team to ensure that any custom padding aligns with the overall design goals and that any new values are approved for use within the system.
Common Use Cases
Is it possible to create padding utilities based on viewport units?
Absolutely! You can define padding values using viewport units like vw
or vh
in your tailwind.config.js
:
// tailwind.config.js
module.exports = {
theme: {
extend: {
padding: {
'5vw': '5vw',
'10vh': '10vh',
},
},
},
}
Conclusion
Extending and customizing the padding scale in Tailwind CSS unlocks a new level of flexibility, allowing you to create unique and consistent designs that align with your project's needs. By carefully integrating custom padding values, creating utility plugins, and adhering to design principles, you can push the boundaries of what's possible with Tailwind's padding capabilities while maintaining a cohesive and user-friendly design.
FAQ
Why should I extend the padding scale instead of using inline styles?
Extending the scale promotes consistency and reusability, while inline styles can lead to inconsistencies.
How does extending the padding scale affect file size?
Adding many custom utilities can increase the generated CSS file size. To mitigate this, use PurgeCSS or Tailwind's built-in purging to remove unused styles in production builds.
Can I use custom padding with Tailwind's JIT mode?
Yes, Tailwind's JIT mode supports arbitrary values and generates styles on demand, including custom padding.
Can I customize the padding for only one component or section?
Yes, you can use component-specific classes or utility-first approaches by combining Tailwind's padding utilities with custom classes in your CSS files or within a <style> block if you're using a framework like Vue or React
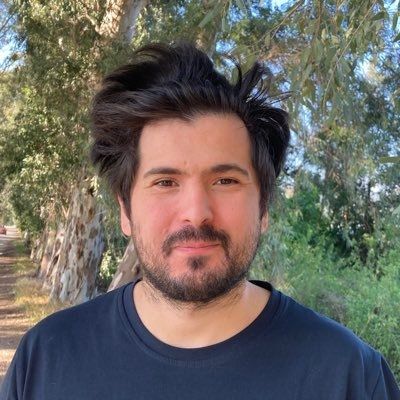
Yucel is a digital product maker and content writer specializing in full-stack development. He is passionate about crafting engaging content and creating innovative solutions that bridge technology and user needs. In his free time, he enjoys discovering new technologies and drawing inspiration from the great outdoors.