Creating React Error Pages with Tailwind CSS
How to setup React 404 pages
Creating a custom React error page 404 with Tailwind CSS lets your users engage even when they hit a dead end.
And guess what? It's simpler than you think!
This guide'll walk you through the steps to build a sleek and functional 404 error page using React and Tailwind CSS.
Let's go!
Why Custom Error Pages Matter
User Experience
A custom error page can make a significant difference in user experience. Instead of a bland and confusing default error message, a well-designed 404 page can guide users back to the main content, making your site feel more polished and professional.
Branding Opportunity
Your 404 page is an extension of your brand. Use this opportunity to showcase your brand's personality and keep your design consistent across all pages, including the error ones.
Setting Up Your React Project
Installing React and Tailwind CSS
First things first, let's set up a new React project and install Tailwind CSS.
npx create-react-app my-error-page
cd my-error-page
npm install tailwindcss
Configuring Tailwind CSS
Next, you'll need to configure Tailwind CSS. Create a tailwind.config.js
file in the root of your project:
npx tailwindcss init
Then, update the tailwind.config.js
file to include your paths:
module.exports = {
purge: ['./src/**/*.{js,jsx,ts,tsx}', './public/index.html'],
darkMode: false, // or 'media' or 'class'
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [],
}
In your src/index.css
, import Tailwind's base, components, and utilities:
@tailwind base;
@tailwind components;
@tailwind utilities;
Creating the 404 Error Page Component
Basic Structure
Create a new component called ErrorPage.js
in your src
folder. This will be the foundation of your 404 error page.
import React from 'react';
const ErrorPage = () => {
return (
<div className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="text-center">
<h1 className="text-6xl font-bold text-gray-800">404</h1>
<p className="text-2xl text-gray-600">Oops! Page not found.</p>
<a href="/" className="mt-4 inline-block px-4 py-2 text-white bg-blue-500 rounded hover:bg-blue-600">Go Home</a>
</div>
</div>
);
}
export default ErrorPage;
Styling with Tailwind CSS
With Tailwind CSS, you can easily style your error page without writing a single line of custom CSS. Here's a breakdown of the classes used:
flex
,items-center
,justify-center
: Centers the content both vertically and horizontally.min-h-screen
,bg-gray-100
: Ensures the error page takes up the full viewport height and applies a light gray background.text-center
: Centers the text.text-6xl
,font-bold
,text-gray-800
: Styles the "404" text.text-2xl
,text-gray-600
: Styles the "Oops! Page not found." text.mt-4
,inline-block
,px-4
,py-2
,text-white
,bg-blue-500
,rounded
,hover:bg-blue-600
: Styles the "Go Home" button.
Integrating the 404 Error Page
Setting Up Routes
Handling Routes
To display the 404 error page for undefined routes, you'll need to set up routing in your React application. First, install react-router-dom
if you haven't already:
npm install react-router-dom
Configuring Routes
In your src/App.js
file, import the necessary components and set up your routes:
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import HomePage from './HomePage';
import ErrorPage from './ErrorPage';
const App = () => {
return (
<Router>
<Switch>
<Route exact path="/" component={HomePage} />
<Route component={ErrorPage} />
</Switch>
</Router>
);
}
export default App;
Here's a breakdown of what each part does:
Router: Wraps your application and enables routing.
Switch: Ensures that only one route is rendered at a time.
Route: Defines a specific path and the component to render. The
exact
prop ensures that the route matches exactly, so the HomePage component only renders for the/
path.ErrorPage: This route has no path, so it will catch all undefined routes, rendering the 404 error page.
By setting up your routes this way, you ensure that users who navigate to a non-existent page will see your custom 404 error page, enhancing their overall experience on your site.
In this setup, the Switch
component from react-router-dom
ensures that only one Route
is rendered at a time. The exact
prop on the home route (/
) ensures that it only matches when the path is exactly /
. Any other route will fall through to the ErrorPage
component.
Adding Some Flair to Your 404 Page
Adding an Image
Images can make your 404 page more engaging. Let's add an image to our error page. First, place your image in the public
folder. For this example, we'll use 404-image.png
.
Update your ErrorPage.js
to include the image:
import React from 'react';
import { Link } from 'react-router-dom';
const ErrorPage = () => {
return (
<div className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="text-center">
<img src="/404-image.png" alt="404" className="w-1/2 mx-auto mb-8" />
<h1 className="text-6xl font-bold text-gray-800">404</h1>
<p className="text-2xl text-gray-600">Oops! Page not found.</p>
<Link to="/" className="mt-4 inline-block px-4 py-2 text-white bg-blue-500 rounded hover:bg-blue-600">Go Home</Link>
</div>
</div>
);
}
export default ErrorPage;
Adding Animation
Animations can add a touch of dynamism to your error page. Let's use Tailwind CSS and a library called react-spring
for animations.
First, install react-spring
:
npm install react-spring
Now, let's add a simple fade-in animation to our ErrorPage.js
:
import React from 'react';
import { Link } from 'react-router-dom';
import { useSpring, animated } from 'react-spring';
const ErrorPage = () => {
const fadeIn = useSpring({ opacity: 1, from: { opacity: 0 } });
return (
<animated.div style={fadeIn} className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="text-center">
<img src="/404-image.png" alt="404" className="w-1/2 mx-auto mb-8" />
<h1 className="text-6xl font-bold text-gray-800">404</h1>
<p className="text-2xl text-gray-600">Oops! Page not found.</p>
<Link to="/" className="mt-4 inline-block px-4 py-2 text-white bg-blue-500 rounded hover:bg-blue-600">Go Home</Link>
</div>
</animated.div>
);
}
export default ErrorPage;
With react-spring
, the useSpring
hook allows us to create a fade-in animation effect. The animated.div
component applies this effect to our error page.
Conclusion
Creating a custom React error page 404 with Tailwind CSS is a simple way to enhance user experience and reinforce your brand.
By following the steps outlined in this guide, you can transform a frustrating experience into a delightful one.
FAQ
Why should I customize my 404 error page?
Customizing your 404 error page improves user experience, keeps users engaged, and aligns with your brand's design.
How do I redirect users from the 404 page?
You can use react-router-dom's Redirect component or a Link component to redirect users to another page, like the homepage.
How do I test my 404 error page?
To test, simply navigate to a non-existent route in your application. You should be redirected to the 404 error page.
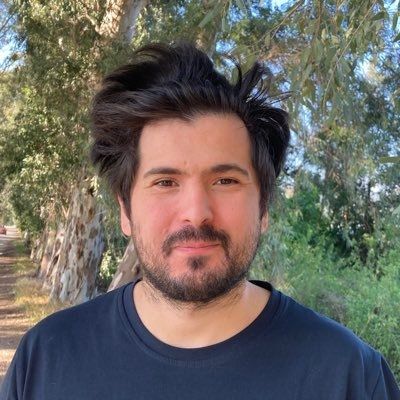
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.