Convert HTML to React Component
Learn the process of converting HTML markup into React components.
Have you ever found yourself staring at a block of HTML code and wishing it could do more? Maybe you’ve built a cool static webpage that looks great, but now you want to breathe some life into it with React.
In this guide, we’re diving into exactly how to convert your HTML code into a reusable React component.
Intro
Let’s face it—HTML is awesome for creating static pages, but when you need interactivity, React is where the magic happens. React components allow you to break down your UI into small, manageable pieces, making it easier to update, maintain, and reuse code. In this post, we’ll walk through the process of taking an existing HTML file and turning it into a fully functional React component.
Why Convert HTML to React Components?
Before diving into the “how,” let’s talk about the “why.” Here are a few reasons why you might want to convert your HTML into React components:
Modularity: React components allow you to isolate parts of your UI. Once a component is built, you can reuse it throughout your application.
Maintainability: With your code broken into components, it’s much easier to update or refactor your code without worrying about breaking the entire application.
Interactivity: HTML by itself is static. With React, you can add state, manage user input, and make your application dynamic.
Ecosystem: React has a rich ecosystem of tools and libraries. Converting to React opens up possibilities like using React Router, state management libraries, and more.
Getting Started with Your React App
Before you convert any HTML, you need a React environment set up on your machine. If you haven’t already, create a new React app using Create React App or your favorite React boilerplate. Here’s a quick reminder of how to do it with Create React App:
Install Create React App:
npx create-react-app my-react-app cd my-react-app npm start
Explore the Project Structure:
Once your app is running, take a look around the folder structure. Notice thesrc
folder—this is where your components will live. Thepublic
folder contains your static HTML, which is usually only used for things like the mainindex.html
.
With your environment ready, it’s time to dive into the actual conversion process.
Step-by-Step Conversion Process
1. Analyze Your HTML
Start by examining your HTML file. Identify the parts you want to convert into a React component. Ask yourself:
Which parts of the HTML are reusable?
Are there any sections that could be broken down further?
What elements might need to interact with JavaScript later on?
For instance, if you have a navigation bar, a main content area, and a footer, each of these can become its own component. This modular approach not only makes your code cleaner but also allows you to manage each piece independently.
2. Set Up Your Component File
Once you’ve decided what to convert, create a new file in your src
folder. Let’s say you’re converting a simple header; you might name your file Header.js
.
In Header.js
, start by importing React:
import React from 'react';
const Header = () => {
return (
<header className="header">
{/* Your HTML content will go here */}
</header>
);
};
export default Header;
This is your basic React component structure. Notice that we’re using a functional component here—it’s straightforward and perfect for most use cases.
3. Convert HTML to JSX
Now comes the crucial part: converting HTML to JSX. JSX is similar to HTML but has a few differences:
Class vs. className: In JSX, you use
className
instead ofclass
.Self-closing Tags: All tags need to be closed. For example,
<img src="..." />
instead of<img src="...">
.JavaScript Expressions: To embed JavaScript expressions, you use curly braces
{}
.
Here’s a quick example. Suppose your original HTML looked like this:
<header class="header">
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
In JSX, this would become:
<header className="header">
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
Notice how we simply changed class
to className
and ensured every tag is properly closed.
4. Organize Your Components
After converting a single block of HTML, think about the overall structure of your page. It might make sense to create separate components for different sections. For example, if your header contains a navigation menu, consider splitting it into a <NavBar />
component. This way, your code is easier to read and manage.
// Header.js
import React from 'react';
import NavBar from './NavBar';
const Header = () => {
return (
<header className="header">
<h1>Welcome to My Website</h1>
<NavBar />
</header>
);
};
export default Header;
And then create a separate NavBar.js
:
import React from 'react';
const NavBar = () => {
return (
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
);
};
export default NavBar;
Breaking your code into smaller components not only helps with readability but also makes it easier to debug and update later on.
5. Incorporate Interactivity
One of the major benefits of converting to React is the ability to add interactivity. Suppose you have a button in your HTML that used to do nothing. Now you can easily add state and event handlers.
For example:
import React, { useState } from 'react';
const InteractiveButton = () => {
const [clickCount, setClickCount] = useState(0);
const handleClick = () => {
setClickCount(clickCount + 1);
};
return (
<button onClick={handleClick}>
Clicked {clickCount} times
</button>
);
};
export default InteractiveButton;
You can then insert this interactive component anywhere within your layout. This is the beauty of React—adding dynamic behavior is a breeze.
Tips and Tricks for a Smooth Conversion
Use Online Tools
There are several online tools available that can help you convert HTML to JSX. While it’s beneficial to understand the conversion process, these tools can save you time for larger projects. They automatically fix some common pitfalls, such as converting attribute names and closing tags.
Leverage Your Code Editor
Modern code editors like Visual Studio Code offer extensions and linting tools that can help catch common errors when writing JSX. These tools can flag issues like missing closing tags or incorrect attribute names in real time.
Keep It Simple
When converting, try to keep your components simple. If you notice a component is doing too much, it’s a sign that you should break it down into smaller, more focused components. This not only makes your code easier to understand but also improves its reusability.
Comment Your Code
During the conversion process, add comments to sections where you’ve made significant changes. Comments help you remember why certain decisions were made, especially when you come back to the code later.
Experiment and Iterate
Don’t be afraid to experiment. React encourages you to try new things and iterate quickly. If you encounter issues or something doesn’t work as expected, take it as an opportunity to learn and adjust your approach.
Common Issues
Converting HTML to React isn’t always a smooth ride. Here are some common challenges you might encounter:
Syntactical Issues
Unclosed Tags: Unlike HTML, JSX requires every tag to be closed. Double-check for self-closing tags, especially with elements like
<img />
or<input />
.Attribute Names: Remember that attributes like
class
andfor
have different names in JSX (className
andhtmlFor
respectively).
JavaScript Integration
Embedding JavaScript: If your HTML file contained inline JavaScript, you’ll need to find a way to integrate that logic into your React component. Often, this means moving the code into event handlers or useEffect hooks.
External Scripts: Instead of relying on external script tags, try to import the required modules using npm. This approach keeps your code cleaner and takes advantage of React’s ecosystem.
CSS and Styling
CSS Files: When converting, ensure that your CSS is correctly imported into your component or globally in your application. Create React App supports importing CSS directly into your JavaScript files, making it easier to maintain styles.
Inline Styles: If you need dynamic styling, consider using inline styles or libraries like styled-components. This makes it easier to update styles based on state changes.
Tools and Libraries to Ease the Conversion Process
Create React App
This is the most common starting point for any new React project. It comes with a lot of built-in functionality and configuration, allowing you to focus on building your components without worrying about setting up Webpack or Babel.
Babel
Babel is a powerful tool that converts JSX into JavaScript that browsers can understand. While Create React App handles Babel configuration for you, knowing a bit about it can help you troubleshoot issues that arise during conversion.
Online Converters
There are online tools available that can automatically convert HTML to JSX. While these aren’t perfect, they can give you a good starting point and help you catch simple syntax issues quickly.
Code Editors
Using an editor like Visual Studio Code with React-specific extensions can drastically improve your workflow. These tools provide real-time feedback, auto-completion, and error checking that can be incredibly helpful during the conversion process.
Best Practices for Organizing Your React Components
Keep Components Focused
Aim to have each component do one thing well. If a component starts to grow too large, consider breaking it down into smaller subcomponents. This approach not only makes your code easier to maintain but also encourages reusability across your project.
File and Folder Structure
Organize your components in a logical folder structure. Many developers use a structure where each component has its own folder containing the component file, styles, and tests. This helps keep related files together and simplifies navigation within your project.
Naming Conventions
Choose clear, descriptive names for your components and CSS classes. Consistent naming makes it easier to understand the purpose of each component and helps avoid confusion when your project scales.
Wrapping Up
Converting HTML to React components is a fantastic way to modernize your web projects. By breaking down your static HTML into modular, reusable pieces, you gain more control over your user interface and set the stage for adding dynamic behavior.
In this guide, we walked through:
Analyzing your HTML: Understanding which parts can be reused.
Setting up your React component: Creating a basic functional component.
Converting HTML to JSX: Adjusting syntax and attributes.
Organizing your code: Breaking your layout into smaller, manageable components.
Enhancing interactivity: Adding state and event handlers.
Using tools and best practices: Leveraging modern tools to make the conversion smoother.
Remember, the key to mastering this conversion process is to take it step-by-step and not get overwhelmed by the details. With practice, you’ll find that what once seemed like a daunting task becomes second nature.
FAQ
Why should I convert HTML to a React component?
It helps you build modular, reusable, and interactive parts of your UI while simplifying maintenance.
What changes are needed when converting HTML to JSX?
You need to update attribute names, ensure all tags are properly closed, and integrate JavaScript expressions as needed.
How can I handle external scripts when converting HTML?
Instead of script tags, import modules via npm and manage functionality using React’s hooks or lifecycle methods.
What tools can simplify the conversion process?
Use tools like Create React App, online HTML-to-JSX converters, and modern code editors with React extensions for a smoother workflow.
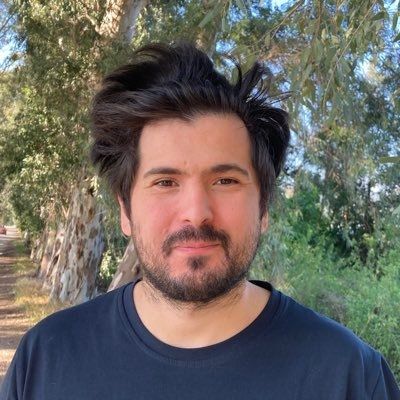
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.