Centering Spinners in Tailwind CSS
Learn how to center spinners in Tailwind CSS
Ever been stuck trying to center a spinner in Tailwind CSS? You're not alone! Centering elements, especially spinners, can be a bit tricky. Let's dive in!
Centering Spinners Matters
A well-centered spinner significantly improves user experience by ensuring that loading animations are visually appealing and perfectly aligned, preventing your site from looking unprofessional and frustrating to users.
Additionally, a centered spinner enhances the aesthetic appeal of your website, making the loading state appear cleaner and more organized, leaving a lasting positive impression on visitors.
Defining a Spinner in Tailwind CSS
Before we center the spinner, we need to create it. Tailwind CSS is a utility-first framework, so we can use its utilities to define a spinner. Here's a basic example of how you can define a spinner:
<!-- Add this in your HTML -->
<div class="loader"></div>
<!-- Add this in your CSS -->
<style>
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
.loader {
border: 4px solid rgba(0, 0, 0, 0.1);
border-left-color: #3490dc;
border-radius: 50%;
width: 40px;
height: 40px;
animation: spin 1s linear infinite;
}
</style>
Methods to Center Spinners in Tailwind CSS
Using Flexbox
Flexbox is a powerful layout tool that can easily center horizontally and vertically elements. Here's how you can use it to center a spinner:
<div class="flex items-center justify-center h-screen">
<div class="loader"></div>
</div>
In this example, flex makes the container a flexbox, items-center centers the spinner vertically, and justify-center centers it horizontally. The h-screen class ensures the container takes up the full height of the viewport.
Using Grid
Grid layout is another excellent way to center elements. It offers a bit more flexibility compared to Flexbox. Here's how to do it:
<div class="grid place-items-center h-screen">
<div class="loader"></div>
</div>
The grid class makes the container a grid, and place-items-center centers the spinner both horizontally and vertically.
Using Absolute Positioning
Absolute positioning can also be used to center a spinner, especially when you want to overlay it on top of other content:
<div class="relative h-screen">
<div class="absolute inset-0 flex items-center justify-center">
<div class="loader"></div>
</div>
</div>
Here, relative is assigned to the parent container to establish a positioning context. The absolute inset-0 class makes the spinner container fill the entire parent, and flex items-center justify-center centers the spinner within it.
Using Margin Auto
Margin auto is a simpler but less flexible method. It's best used when you have a fixed-width and height for your spinner:
<div class="h-screen flex items-center justify-center">
<div class="loader m-auto"></div>
</div>
The m-auto class sets the margin to auto, centering the spinner within its container.
Customizing Your Spinner
To integrate this loader style into your Tailwind CSS project, you'll need to extend your Tailwind CSS configuration to include custom styles. Here’s how you can do it:
Create the Loader HTML: Add the HTML structure for the loader somewhere in your HTML file:
<div class="loader"></div>
Extend Tailwind CSS Configuration: Modify your
tailwind.config.js
file to include the custom styles for the loader. Tailwind CSS allows you to extend the default theme with custom values.module.exports = { theme: { extend: { keyframes: { spin: { '0%': { transform: 'rotate(0deg)' }, '100%': { transform: 'rotate(360deg)' }, } }, animation: { spin: 'spin 1s linear infinite', }, borderColor: { loader: 'rgba(0, 0, 0, 0.1)', loaderLeft: '#38bdf8', }, }, }, }
Add Custom CSS: Add the custom CSS in your global CSS file or a specific component's CSS file:
/* global.css or component.css */ @layer components { .loader { @apply border-4 rounded-full w-10 h-10; border-color: theme('borderColor.loader'); border-left-color: theme('borderColor.loaderLeft'); animation: theme('animation.spin'); } }
Ensure Your Project is Set Up Correctly: Make sure your Tailwind CSS setup is correctly configured to process the custom configuration. This usually involves having Tailwind CSS installed and configured with a tailwind.config.js file and importing the necessary files in your main CSS file.
@tailwind base; @tailwind components; @tailwind utilities; @import 'path/to/global.css'; /* If you placed the custom CSS in a separate file */
By following these steps, you should be able to use the custom loader style within your Tailwind CSS project. The loader will have the desired border color and animation as specified in the custom configuration.
Adding Colors
Tailwind CSS makes it easy to customize the color of your spinner. You can modify the CSS to change the color:
You can modify styles on tailwind.config.js file
<style>
.loader {
border: 4px solid rgba(0, 0, 0, 0.1);
border-left-color: #38bdf8; /* Change this to any color you like */
border-radius: 50%;
width: 40px;
height: 40px;
animation: spin 1s linear infinite;
}
</style>
Adjusting Size
You can also adjust the size of your spinner using Tailwind's width and height utilities:
You can modify styles on
tailwind.config.js
file
<style>
.loader {
border: 4px solid rgba(0, 0, 0, 0.1); border-left-color: #3490dc; border-radius: 50%; width: 40px; height: 40px; animation: spin 1s linear infinite; } </style>
You can adjust the width and height properties in the CSS to change the size of your spinner. Alternatively, you can use Tailwind's utility classes directly in your HTML:
<div class="loader w-16 h-16"></div>
Animations
Tailwind CSS provides several animation utilities that you can use to make your spinner more dynamic. However, for custom animations like spinning, you'll need to define your own keyframes, as shown earlier. Here's a quick recap:
<style>
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
.loader {
border: 4px solid rgba(0, 0, 0, 0.1);
border-left-color: #3490dc;
border-radius: 50%;
width: 40px;
height: 40px;
animation: spin 1s linear infinite;
}
</style>
Conclusion
Centering spinners in Tailwind CSS doesn't have to be a headache. Whether you prefer Flexbox, Grid, Absolute Positioning, or Margin Auto, Tailwind offers a variety of methods to suit your needs. Remember, a well-centered spinner can significantly enhance user experience and add to the aesthetic appeal of your site.
By following this guide, you'll master the art of centering spinners in Tailwind CSS, ensuring that your loading animations are always perfectly aligned and visually appealing. If you have any more questions or need further assistance, feel free to reach out. Happy coding!
FAQ
How do I center a spinner both horizontally and vertically in Tailwind CSS?
You can use Flexbox or Grid for this purpose. For Flexbox, use flex, items-center, and justify-center. For Grid, use grid and place-items-center.
Can I customize the color of my spinner in Tailwind CSS?
Yes, you can easily customize the color by modifying the CSS properties like border-left-color.
How do I adjust the size of my spinner in Tailwind CSS?
Use Tailwind's width and height utilities like w-16 and h-16 or modify the width and height properties in your custom CSS.
Is it better to use Flexbox or Grid for centering a spinner?
Both Flexbox and Grid are excellent for centering elements. Flexbox is simpler and more intuitive for single-axis alignment (either horizontal or vertical), while Grid offers more flexibility and is better for complex layouts requiring two-dimensional alignment.
What is the best method for centering a spinner in a full-screen overlay?
Using Flexbox or Grid within a full-screen container is the best approach. Apply h-screen to the container and use either flex items-center justify-center or grid place-items-center to center the spinner.
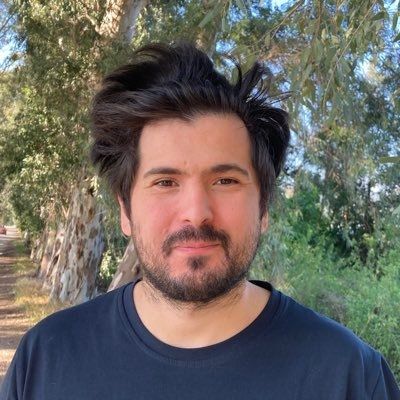
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.