Building Progress Bars with Tailwind CSS
A Step-by-Step Guide to build Tailwind progress bar components
Introduction
Hey there, web developers!
Progress bars are essential UI components that visually represent the completion of a task or process. Whether you're tracking a file upload, a quiz completion, or a project milestone, progress bars can make your web app more interactive and user-friendly.
In this guide, we'll explore building progress bars with Tailwind CSS.
Why Tailwind CSS for Progress Bars?
Before we jump into the nitty-gritty of coding, let's discuss why Tailwind CSS is a fantastic choice for building progress bars. Tailwind CSS is:
Utility-First: Tailwind provides low-level utility classes that let you build custom designs directly in your HTML.
Responsive: Easily create responsive designs that look great on any device.
Customizable: Tailwind's configuration file allows for extensive customization to fit your project's needs.
Efficient: Write less custom CSS and speed up your development process.
Getting Started with Tailwind CSS
First things first, you need to set up Tailwind CSS in your project.
If you haven't done so, follow these steps:
1. Install Tailwind CSS:
npm install tailwindcss
2. Create a Configuration File:
npx tailwindcss init
3. Configure Your CSS File:
Add the following lines to your CSS file:
@tailwind base;
@tailwind components;
@tailwind utilities;
4. Build Your CSS:
Use the Tailwind CLI to generate your CSS:
npx tailwindcss build -o output.css
Creating a Basic Progress Bar
Now that we have Tailwind CSS set up, let's create a basic progress bar.
HTML Structure
First, create a simple HTML structure for your progress bar:
<div class="w-full bg-gray-200 rounded-full">
<div class="bg-blue-600 text-xs font-medium text-blue-100 text-center p-0.5 leading-none rounded-l-full" style="width: 50%">50%</div>
</div>
Tailwind Classes Explanation
w-full
: Sets the width of the progress bar container to 100%.bg-gray-200
: Applies a light gray background to the container.rounded-full
: Rounds the corners of the container.bg-blue-600
: Sets the background color of the progress bar to blue.text-xs
: Applies a small font size to the text inside the progress bar.font-medium
: Sets the font weight to medium.text-blue-100:
Changes the text color to light blue.text-center
: Centers the text inside the progress bar.p-0.5
: Adds padding to the text.leading-none
: Removes extra line height.rounded-l-full
: Rounds the left side of the progress bar.style="width: 50%"
: Sets the width of the progress bar to 50%.
Adding Animation to Your Progress Bar
Static progress bars are great, but adding animation can make them even more engaging. Let's animate our progress bar using Tailwind CSS.
HTML Structure with Animation
<div class="w-full bg-gray-200 rounded-full">
<div class="bg-blue-600 text-xs font-medium text-blue-100 text-center p-0.5 leading-none rounded-l-full animate-progress" style="width: 50%">50%</div>
</div>
Tailwind Classes and Custom Animation
To add animation, we need to define a custom animation in Tailwind's configuration file.
1. Open Your Tailwind Configuration File (`tailwind.config.js`):
module.exports = {
theme: {
extend: {
keyframes: {
progress: {
'0%': { width: '0%' },
'100%': { width: '50%' },
},
},
animation: {
progress: 'progress 2s ease-in-out',
},
},
},
}
2. Rebuild your CSS:
npx tailwindcss build -o output.css
Explanation
Keyframes: Define the animation steps, starting from 0% width to 50% width.
Animation: Name the animation progress and specify that it runs for 2 seconds with an ease-in-out timing function.
Customizing Your Progress Bar
Tailwind CSS makes it easy to customize your progress bars to fit your design requirements. Here are some customization ideas:
Changing Colors
Want a different color for your progress bar? No problem! Tailwind CSS offers a wide range of color utilities.
<div class="w-full bg-gray-200 rounded-full">
<div class="bg-green-500 text-xs font-medium text-green-100 text-center p-0.5 leading-none rounded-l-full" style="width: 75%">75%</div>
</div>
Adding Stripes
You can add stripes to your progress bar for a more dynamic look.
<div class="w-full bg-gray-200 rounded-full">
<div class="bg-blue-600 text-xs font-medium text-blue-100 text-center p-0.5 leading-none rounded-l-full animate-progress striped-bar" style="width: 50%">50%</div>
</div>
CSS for Stripes
Add the following CSS to your stylesheet:
.striped-bar {
background-image: linear-gradient(45deg, rgba(255, 255, 255, 0.15) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, 0.15) 50%, rgba(255, 255, 255, 0.15) 75%, transparent 75%, transparent);
background-size: 1rem 1rem;
}
Adding a Gradient
Gradients can make your progress bar look more modern.
<div class="w-full bg-gray-200 rounded-full">
<div class="bg-gradient-to-r from-green-400 to-blue-500 text-xs font-medium text-white text-center p-0.5 leading-none rounded-l-full animate-progress" style="width: 60%">60%</div>
</div>
Making Progress Bars Responsive
Responsive design is crucial for modern web development. Tailwind CSS makes it straightforward to create responsive progress bars.
Example
Here's how you can make your progress bar responsive:
<div class="w-full sm:w-1/2 md:w-1/3 lg:w-1/4 bg-gray-200 rounded-full">
<div class="bg-blue-600 text-xs font-medium text-blue-100 text-center p-0.5 leading-none rounded-l-full animate-progress" style="width: 50%">50%</div>
</div>
Explanation
sm:w-1/2
: Sets the width to 50% on small screens.md:w-1/3
: Sets the width to one-third on medium screens.lg:w-1/4
: Sets the width to one-fourth on large screens.
Conclusion
And there you have it! You've learned how to create, customize, and animate progress bars using Tailwind CSS. Whether you're a seasoned developer or just getting started, Tailwind's utility-first approach makes it easy to build beautiful, responsive progress bars quickly. So go ahead, add some polish to your web projects and keep your users engaged!
Happy coding!
FAQ
Can I customize the colors of my progress bar?
Absolutely! Tailwind CSS offers a wide range of color utilities that you can use to customize your progress bar. You can also define custom colors in your Tailwind configuration file.
How can I make my progress bar responsive?
You can make your progress bar responsive by using Tailwind's responsive design utilities. For example, you can set different widths for different screen sizes using classes like sm:w-1/2, md:w-1/3, and lg:w-1/4.
Is it possible to add animations to my progress bar?
Yes, you can add animations to your progress bar using Tailwind's animation utilities. You can define custom animations in your Tailwind configuration file and apply them to your progress bar.
Can I add stripes or gradients to my progress bar?
Yes, you can add stripes or gradients to your progress bar by using custom CSS. Tailwind CSS makes it easy to integrate custom styles with its utility classes.
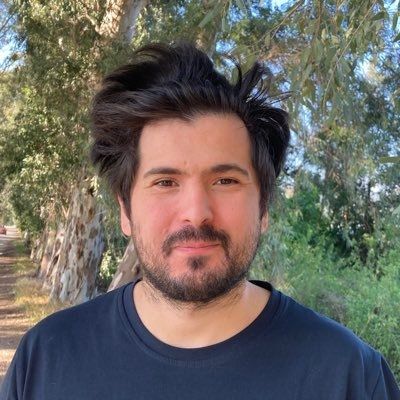
Yucel is a digital product creator and content writer with a knack for full-stack development. He loves blending technical know-how with engaging storytelling to build practical, user-friendly solutions. When he's not coding or writing, you'll likely find him exploring new tech trends or getting inspired by nature.